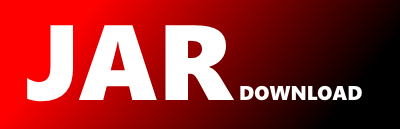
org.bimserver.models.ifc4.IfcWindowStyleOperationEnum Maven / Gradle / Ivy
Show all versions of pluginbase Show documentation
/**
* Copyright (C) 2009-2014 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.bimserver.models.ifc4;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import org.eclipse.emf.common.util.Enumerator;
/**
*
* A representation of the literals of the enumeration 'Ifc Window Style Operation Enum',
* and utility methods for working with them.
*
* @see org.bimserver.models.ifc4.Ifc4Package#getIfcWindowStyleOperationEnum()
* @model
* @generated
*/
public enum IfcWindowStyleOperationEnum implements Enumerator {
/**
* The 'NULL' literal object.
*
*
* @see #NULL_VALUE
* @generated
* @ordered
*/
NULL(0, "NULL", "NULL"),
/**
* The 'NOTDEFINED' literal object.
*
*
* @see #NOTDEFINED_VALUE
* @generated
* @ordered
*/
NOTDEFINED(1, "NOTDEFINED", "NOTDEFINED"),
/**
* The 'DOUBLE PANEL HORIZONTAL' literal object.
*
*
* @see #DOUBLE_PANEL_HORIZONTAL_VALUE
* @generated
* @ordered
*/
DOUBLE_PANEL_HORIZONTAL(2, "DOUBLE_PANEL_HORIZONTAL", "DOUBLE_PANEL_HORIZONTAL"),
/**
* The 'TRIPLE PANEL BOTTOM' literal object.
*
*
* @see #TRIPLE_PANEL_BOTTOM_VALUE
* @generated
* @ordered
*/
TRIPLE_PANEL_BOTTOM(3, "TRIPLE_PANEL_BOTTOM", "TRIPLE_PANEL_BOTTOM"),
/**
* The 'TRIPLE PANEL LEFT' literal object.
*
*
* @see #TRIPLE_PANEL_LEFT_VALUE
* @generated
* @ordered
*/
TRIPLE_PANEL_LEFT(4, "TRIPLE_PANEL_LEFT", "TRIPLE_PANEL_LEFT"),
/**
* The 'DOUBLE PANEL VERTICAL' literal object.
*
*
* @see #DOUBLE_PANEL_VERTICAL_VALUE
* @generated
* @ordered
*/
DOUBLE_PANEL_VERTICAL(5, "DOUBLE_PANEL_VERTICAL", "DOUBLE_PANEL_VERTICAL"),
/**
* The 'TRIPLE PANEL HORIZONTAL' literal object.
*
*
* @see #TRIPLE_PANEL_HORIZONTAL_VALUE
* @generated
* @ordered
*/
TRIPLE_PANEL_HORIZONTAL(6, "TRIPLE_PANEL_HORIZONTAL", "TRIPLE_PANEL_HORIZONTAL"),
/**
* The 'SINGLE PANEL' literal object.
*
*
* @see #SINGLE_PANEL_VALUE
* @generated
* @ordered
*/
SINGLE_PANEL(7, "SINGLE_PANEL", "SINGLE_PANEL"),
/**
* The 'TRIPLE PANEL RIGHT' literal object.
*
*
* @see #TRIPLE_PANEL_RIGHT_VALUE
* @generated
* @ordered
*/
TRIPLE_PANEL_RIGHT(8, "TRIPLE_PANEL_RIGHT", "TRIPLE_PANEL_RIGHT"),
/**
* The 'USERDEFINED' literal object.
*
*
* @see #USERDEFINED_VALUE
* @generated
* @ordered
*/
USERDEFINED(9, "USERDEFINED", "USERDEFINED"),
/**
* The 'TRIPLE PANEL VERTICAL' literal object.
*
*
* @see #TRIPLE_PANEL_VERTICAL_VALUE
* @generated
* @ordered
*/
TRIPLE_PANEL_VERTICAL(10, "TRIPLE_PANEL_VERTICAL", "TRIPLE_PANEL_VERTICAL"),
/**
* The 'TRIPLE PANEL TOP' literal object.
*
*
* @see #TRIPLE_PANEL_TOP_VALUE
* @generated
* @ordered
*/
TRIPLE_PANEL_TOP(11, "TRIPLE_PANEL_TOP", "TRIPLE_PANEL_TOP");
/**
* The 'NULL' literal value.
*
*
* If the meaning of 'NULL' literal object isn't clear,
* there really should be more of a description here...
*
*
* @see #NULL
* @model
* @generated
* @ordered
*/
public static final int NULL_VALUE = 0;
/**
* The 'NOTDEFINED' literal value.
*
*
* If the meaning of 'NOTDEFINED' literal object isn't clear,
* there really should be more of a description here...
*
*
* @see #NOTDEFINED
* @model
* @generated
* @ordered
*/
public static final int NOTDEFINED_VALUE = 1;
/**
* The 'DOUBLE PANEL HORIZONTAL' literal value.
*
*
* If the meaning of 'DOUBLE PANEL HORIZONTAL' literal object isn't clear,
* there really should be more of a description here...
*
*
* @see #DOUBLE_PANEL_HORIZONTAL
* @model
* @generated
* @ordered
*/
public static final int DOUBLE_PANEL_HORIZONTAL_VALUE = 2;
/**
* The 'TRIPLE PANEL BOTTOM' literal value.
*
*
* If the meaning of 'TRIPLE PANEL BOTTOM' literal object isn't clear,
* there really should be more of a description here...
*
*
* @see #TRIPLE_PANEL_BOTTOM
* @model
* @generated
* @ordered
*/
public static final int TRIPLE_PANEL_BOTTOM_VALUE = 3;
/**
* The 'TRIPLE PANEL LEFT' literal value.
*
*
* If the meaning of 'TRIPLE PANEL LEFT' literal object isn't clear,
* there really should be more of a description here...
*
*
* @see #TRIPLE_PANEL_LEFT
* @model
* @generated
* @ordered
*/
public static final int TRIPLE_PANEL_LEFT_VALUE = 4;
/**
* The 'DOUBLE PANEL VERTICAL' literal value.
*
*
* If the meaning of 'DOUBLE PANEL VERTICAL' literal object isn't clear,
* there really should be more of a description here...
*
*
* @see #DOUBLE_PANEL_VERTICAL
* @model
* @generated
* @ordered
*/
public static final int DOUBLE_PANEL_VERTICAL_VALUE = 5;
/**
* The 'TRIPLE PANEL HORIZONTAL' literal value.
*
*
* If the meaning of 'TRIPLE PANEL HORIZONTAL' literal object isn't clear,
* there really should be more of a description here...
*
*
* @see #TRIPLE_PANEL_HORIZONTAL
* @model
* @generated
* @ordered
*/
public static final int TRIPLE_PANEL_HORIZONTAL_VALUE = 6;
/**
* The 'SINGLE PANEL' literal value.
*
*
* If the meaning of 'SINGLE PANEL' literal object isn't clear,
* there really should be more of a description here...
*
*
* @see #SINGLE_PANEL
* @model
* @generated
* @ordered
*/
public static final int SINGLE_PANEL_VALUE = 7;
/**
* The 'TRIPLE PANEL RIGHT' literal value.
*
*
* If the meaning of 'TRIPLE PANEL RIGHT' literal object isn't clear,
* there really should be more of a description here...
*
*
* @see #TRIPLE_PANEL_RIGHT
* @model
* @generated
* @ordered
*/
public static final int TRIPLE_PANEL_RIGHT_VALUE = 8;
/**
* The 'USERDEFINED' literal value.
*
*
* If the meaning of 'USERDEFINED' literal object isn't clear,
* there really should be more of a description here...
*
*
* @see #USERDEFINED
* @model
* @generated
* @ordered
*/
public static final int USERDEFINED_VALUE = 9;
/**
* The 'TRIPLE PANEL VERTICAL' literal value.
*
*
* If the meaning of 'TRIPLE PANEL VERTICAL' literal object isn't clear,
* there really should be more of a description here...
*
*
* @see #TRIPLE_PANEL_VERTICAL
* @model
* @generated
* @ordered
*/
public static final int TRIPLE_PANEL_VERTICAL_VALUE = 10;
/**
* The 'TRIPLE PANEL TOP' literal value.
*
*
* If the meaning of 'TRIPLE PANEL TOP' literal object isn't clear,
* there really should be more of a description here...
*
*
* @see #TRIPLE_PANEL_TOP
* @model
* @generated
* @ordered
*/
public static final int TRIPLE_PANEL_TOP_VALUE = 11;
/**
* An array of all the 'Ifc Window Style Operation Enum' enumerators.
*
*
* @generated
*/
private static final IfcWindowStyleOperationEnum[] VALUES_ARRAY = new IfcWindowStyleOperationEnum[] { NULL,
NOTDEFINED, DOUBLE_PANEL_HORIZONTAL, TRIPLE_PANEL_BOTTOM, TRIPLE_PANEL_LEFT, DOUBLE_PANEL_VERTICAL,
TRIPLE_PANEL_HORIZONTAL, SINGLE_PANEL, TRIPLE_PANEL_RIGHT, USERDEFINED, TRIPLE_PANEL_VERTICAL,
TRIPLE_PANEL_TOP, };
/**
* A public read-only list of all the 'Ifc Window Style Operation Enum' enumerators.
*
*
* @generated
*/
public static final List VALUES = Collections
.unmodifiableList(Arrays.asList(VALUES_ARRAY));
/**
* Returns the 'Ifc Window Style Operation Enum' literal with the specified literal value.
*
*
* @param literal the literal.
* @return the matching enumerator or null
.
* @generated
*/
public static IfcWindowStyleOperationEnum get(String literal) {
for (int i = 0; i < VALUES_ARRAY.length; ++i) {
IfcWindowStyleOperationEnum result = VALUES_ARRAY[i];
if (result.toString().equals(literal)) {
return result;
}
}
return null;
}
/**
* Returns the 'Ifc Window Style Operation Enum' literal with the specified name.
*
*
* @param name the name.
* @return the matching enumerator or null
.
* @generated
*/
public static IfcWindowStyleOperationEnum getByName(String name) {
for (int i = 0; i < VALUES_ARRAY.length; ++i) {
IfcWindowStyleOperationEnum result = VALUES_ARRAY[i];
if (result.getName().equals(name)) {
return result;
}
}
return null;
}
/**
* Returns the 'Ifc Window Style Operation Enum' literal with the specified integer value.
*
*
* @param value the integer value.
* @return the matching enumerator or null
.
* @generated
*/
public static IfcWindowStyleOperationEnum get(int value) {
switch (value) {
case NULL_VALUE:
return NULL;
case NOTDEFINED_VALUE:
return NOTDEFINED;
case DOUBLE_PANEL_HORIZONTAL_VALUE:
return DOUBLE_PANEL_HORIZONTAL;
case TRIPLE_PANEL_BOTTOM_VALUE:
return TRIPLE_PANEL_BOTTOM;
case TRIPLE_PANEL_LEFT_VALUE:
return TRIPLE_PANEL_LEFT;
case DOUBLE_PANEL_VERTICAL_VALUE:
return DOUBLE_PANEL_VERTICAL;
case TRIPLE_PANEL_HORIZONTAL_VALUE:
return TRIPLE_PANEL_HORIZONTAL;
case SINGLE_PANEL_VALUE:
return SINGLE_PANEL;
case TRIPLE_PANEL_RIGHT_VALUE:
return TRIPLE_PANEL_RIGHT;
case USERDEFINED_VALUE:
return USERDEFINED;
case TRIPLE_PANEL_VERTICAL_VALUE:
return TRIPLE_PANEL_VERTICAL;
case TRIPLE_PANEL_TOP_VALUE:
return TRIPLE_PANEL_TOP;
}
return null;
}
/**
*
*
* @generated
*/
private final int value;
/**
*
*
* @generated
*/
private final String name;
/**
*
*
* @generated
*/
private final String literal;
/**
* Only this class can construct instances.
*
*
* @generated
*/
private IfcWindowStyleOperationEnum(int value, String name, String literal) {
this.value = value;
this.name = name;
this.literal = literal;
}
/**
*
*
* @generated
*/
public int getValue() {
return value;
}
/**
*
*
* @generated
*/
public String getName() {
return name;
}
/**
*
*
* @generated
*/
public String getLiteral() {
return literal;
}
/**
* Returns the literal value of the enumerator, which is its string representation.
*
*
* @generated
*/
@Override
public String toString() {
return literal;
}
} //IfcWindowStyleOperationEnum