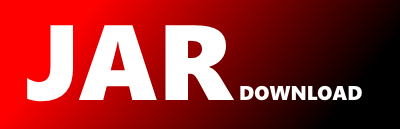
org.bimserver.models.ifc4.impl.IfcDoorPanelPropertiesImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pluginbase Show documentation
Show all versions of pluginbase Show documentation
Base project for BIMserver plugin development. Some plugins mights also need the Shared library
The newest version!
/**
* Copyright (C) 2009-2014 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.bimserver.models.ifc4.impl;
import org.bimserver.models.ifc4.Ifc4Package;
import org.bimserver.models.ifc4.IfcDoorPanelOperationEnum;
import org.bimserver.models.ifc4.IfcDoorPanelPositionEnum;
import org.bimserver.models.ifc4.IfcDoorPanelProperties;
import org.bimserver.models.ifc4.IfcShapeAspect;
import org.eclipse.emf.ecore.EClass;
/**
*
* An implementation of the model object 'Ifc Door Panel Properties'.
*
*
* The following features are implemented:
*
*
* - {@link org.bimserver.models.ifc4.impl.IfcDoorPanelPropertiesImpl#getPanelDepth Panel Depth}
* - {@link org.bimserver.models.ifc4.impl.IfcDoorPanelPropertiesImpl#getPanelDepthAsString Panel Depth As String}
* - {@link org.bimserver.models.ifc4.impl.IfcDoorPanelPropertiesImpl#getPanelOperation Panel Operation}
* - {@link org.bimserver.models.ifc4.impl.IfcDoorPanelPropertiesImpl#getPanelWidth Panel Width}
* - {@link org.bimserver.models.ifc4.impl.IfcDoorPanelPropertiesImpl#getPanelWidthAsString Panel Width As String}
* - {@link org.bimserver.models.ifc4.impl.IfcDoorPanelPropertiesImpl#getPanelPosition Panel Position}
* - {@link org.bimserver.models.ifc4.impl.IfcDoorPanelPropertiesImpl#getShapeAspectStyle Shape Aspect Style}
*
*
* @generated
*/
public class IfcDoorPanelPropertiesImpl extends IfcPreDefinedPropertySetImpl implements IfcDoorPanelProperties {
/**
*
*
* @generated
*/
protected IfcDoorPanelPropertiesImpl() {
super();
}
/**
*
*
* @generated
*/
@Override
protected EClass eStaticClass() {
return Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES;
}
/**
*
*
* @generated
*/
public double getPanelDepth() {
return (Double) eGet(Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES__PANEL_DEPTH, true);
}
/**
*
*
* @generated
*/
public void setPanelDepth(double newPanelDepth) {
eSet(Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES__PANEL_DEPTH, newPanelDepth);
}
/**
*
*
* @generated
*/
public void unsetPanelDepth() {
eUnset(Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES__PANEL_DEPTH);
}
/**
*
*
* @generated
*/
public boolean isSetPanelDepth() {
return eIsSet(Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES__PANEL_DEPTH);
}
/**
*
*
* @generated
*/
public String getPanelDepthAsString() {
return (String) eGet(Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES__PANEL_DEPTH_AS_STRING, true);
}
/**
*
*
* @generated
*/
public void setPanelDepthAsString(String newPanelDepthAsString) {
eSet(Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES__PANEL_DEPTH_AS_STRING, newPanelDepthAsString);
}
/**
*
*
* @generated
*/
public void unsetPanelDepthAsString() {
eUnset(Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES__PANEL_DEPTH_AS_STRING);
}
/**
*
*
* @generated
*/
public boolean isSetPanelDepthAsString() {
return eIsSet(Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES__PANEL_DEPTH_AS_STRING);
}
/**
*
*
* @generated
*/
public IfcDoorPanelOperationEnum getPanelOperation() {
return (IfcDoorPanelOperationEnum) eGet(Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES__PANEL_OPERATION, true);
}
/**
*
*
* @generated
*/
public void setPanelOperation(IfcDoorPanelOperationEnum newPanelOperation) {
eSet(Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES__PANEL_OPERATION, newPanelOperation);
}
/**
*
*
* @generated
*/
public double getPanelWidth() {
return (Double) eGet(Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES__PANEL_WIDTH, true);
}
/**
*
*
* @generated
*/
public void setPanelWidth(double newPanelWidth) {
eSet(Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES__PANEL_WIDTH, newPanelWidth);
}
/**
*
*
* @generated
*/
public void unsetPanelWidth() {
eUnset(Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES__PANEL_WIDTH);
}
/**
*
*
* @generated
*/
public boolean isSetPanelWidth() {
return eIsSet(Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES__PANEL_WIDTH);
}
/**
*
*
* @generated
*/
public String getPanelWidthAsString() {
return (String) eGet(Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES__PANEL_WIDTH_AS_STRING, true);
}
/**
*
*
* @generated
*/
public void setPanelWidthAsString(String newPanelWidthAsString) {
eSet(Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES__PANEL_WIDTH_AS_STRING, newPanelWidthAsString);
}
/**
*
*
* @generated
*/
public void unsetPanelWidthAsString() {
eUnset(Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES__PANEL_WIDTH_AS_STRING);
}
/**
*
*
* @generated
*/
public boolean isSetPanelWidthAsString() {
return eIsSet(Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES__PANEL_WIDTH_AS_STRING);
}
/**
*
*
* @generated
*/
public IfcDoorPanelPositionEnum getPanelPosition() {
return (IfcDoorPanelPositionEnum) eGet(Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES__PANEL_POSITION, true);
}
/**
*
*
* @generated
*/
public void setPanelPosition(IfcDoorPanelPositionEnum newPanelPosition) {
eSet(Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES__PANEL_POSITION, newPanelPosition);
}
/**
*
*
* @generated
*/
public IfcShapeAspect getShapeAspectStyle() {
return (IfcShapeAspect) eGet(Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES__SHAPE_ASPECT_STYLE, true);
}
/**
*
*
* @generated
*/
public void setShapeAspectStyle(IfcShapeAspect newShapeAspectStyle) {
eSet(Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES__SHAPE_ASPECT_STYLE, newShapeAspectStyle);
}
/**
*
*
* @generated
*/
public void unsetShapeAspectStyle() {
eUnset(Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES__SHAPE_ASPECT_STYLE);
}
/**
*
*
* @generated
*/
public boolean isSetShapeAspectStyle() {
return eIsSet(Ifc4Package.Literals.IFC_DOOR_PANEL_PROPERTIES__SHAPE_ASPECT_STYLE);
}
} //IfcDoorPanelPropertiesImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy