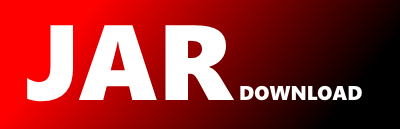
org.bimserver.models.ifc4.impl.IfcGridAxisImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pluginbase Show documentation
Show all versions of pluginbase Show documentation
Base project for BIMserver plugin development. Some plugins mights also need the Shared library
The newest version!
/**
* Copyright (C) 2009-2014 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.bimserver.models.ifc4.impl;
import org.bimserver.emf.IdEObjectImpl;
import org.bimserver.models.ifc4.Ifc4Package;
import org.bimserver.models.ifc4.IfcCurve;
import org.bimserver.models.ifc4.IfcGrid;
import org.bimserver.models.ifc4.IfcGridAxis;
import org.bimserver.models.ifc4.IfcVirtualGridIntersection;
import org.bimserver.models.ifc4.Tristate;
import org.eclipse.emf.common.util.EList;
import org.eclipse.emf.ecore.EClass;
/**
*
* An implementation of the model object 'Ifc Grid Axis'.
*
*
* The following features are implemented:
*
*
* - {@link org.bimserver.models.ifc4.impl.IfcGridAxisImpl#getAxisTag Axis Tag}
* - {@link org.bimserver.models.ifc4.impl.IfcGridAxisImpl#getAxisCurve Axis Curve}
* - {@link org.bimserver.models.ifc4.impl.IfcGridAxisImpl#getSameSense Same Sense}
* - {@link org.bimserver.models.ifc4.impl.IfcGridAxisImpl#getPartOfW Part Of W}
* - {@link org.bimserver.models.ifc4.impl.IfcGridAxisImpl#getPartOfV Part Of V}
* - {@link org.bimserver.models.ifc4.impl.IfcGridAxisImpl#getPartOfU Part Of U}
* - {@link org.bimserver.models.ifc4.impl.IfcGridAxisImpl#getHasIntersections Has Intersections}
*
*
* @generated
*/
public class IfcGridAxisImpl extends IdEObjectImpl implements IfcGridAxis {
/**
*
*
* @generated
*/
protected IfcGridAxisImpl() {
super();
}
/**
*
*
* @generated
*/
@Override
protected EClass eStaticClass() {
return Ifc4Package.Literals.IFC_GRID_AXIS;
}
/**
*
*
* @generated
*/
@Override
protected int eStaticFeatureCount() {
return 0;
}
/**
*
*
* @generated
*/
public String getAxisTag() {
return (String) eGet(Ifc4Package.Literals.IFC_GRID_AXIS__AXIS_TAG, true);
}
/**
*
*
* @generated
*/
public void setAxisTag(String newAxisTag) {
eSet(Ifc4Package.Literals.IFC_GRID_AXIS__AXIS_TAG, newAxisTag);
}
/**
*
*
* @generated
*/
public void unsetAxisTag() {
eUnset(Ifc4Package.Literals.IFC_GRID_AXIS__AXIS_TAG);
}
/**
*
*
* @generated
*/
public boolean isSetAxisTag() {
return eIsSet(Ifc4Package.Literals.IFC_GRID_AXIS__AXIS_TAG);
}
/**
*
*
* @generated
*/
public IfcCurve getAxisCurve() {
return (IfcCurve) eGet(Ifc4Package.Literals.IFC_GRID_AXIS__AXIS_CURVE, true);
}
/**
*
*
* @generated
*/
public void setAxisCurve(IfcCurve newAxisCurve) {
eSet(Ifc4Package.Literals.IFC_GRID_AXIS__AXIS_CURVE, newAxisCurve);
}
/**
*
*
* @generated
*/
public Tristate getSameSense() {
return (Tristate) eGet(Ifc4Package.Literals.IFC_GRID_AXIS__SAME_SENSE, true);
}
/**
*
*
* @generated
*/
public void setSameSense(Tristate newSameSense) {
eSet(Ifc4Package.Literals.IFC_GRID_AXIS__SAME_SENSE, newSameSense);
}
/**
*
*
* @generated
*/
@SuppressWarnings("unchecked")
public EList getPartOfW() {
return (EList) eGet(Ifc4Package.Literals.IFC_GRID_AXIS__PART_OF_W, true);
}
/**
*
*
* @generated
*/
public void unsetPartOfW() {
eUnset(Ifc4Package.Literals.IFC_GRID_AXIS__PART_OF_W);
}
/**
*
*
* @generated
*/
public boolean isSetPartOfW() {
return eIsSet(Ifc4Package.Literals.IFC_GRID_AXIS__PART_OF_W);
}
/**
*
*
* @generated
*/
@SuppressWarnings("unchecked")
public EList getPartOfV() {
return (EList) eGet(Ifc4Package.Literals.IFC_GRID_AXIS__PART_OF_V, true);
}
/**
*
*
* @generated
*/
public void unsetPartOfV() {
eUnset(Ifc4Package.Literals.IFC_GRID_AXIS__PART_OF_V);
}
/**
*
*
* @generated
*/
public boolean isSetPartOfV() {
return eIsSet(Ifc4Package.Literals.IFC_GRID_AXIS__PART_OF_V);
}
/**
*
*
* @generated
*/
@SuppressWarnings("unchecked")
public EList getPartOfU() {
return (EList) eGet(Ifc4Package.Literals.IFC_GRID_AXIS__PART_OF_U, true);
}
/**
*
*
* @generated
*/
public void unsetPartOfU() {
eUnset(Ifc4Package.Literals.IFC_GRID_AXIS__PART_OF_U);
}
/**
*
*
* @generated
*/
public boolean isSetPartOfU() {
return eIsSet(Ifc4Package.Literals.IFC_GRID_AXIS__PART_OF_U);
}
/**
*
*
* @generated
*/
@SuppressWarnings("unchecked")
public EList getHasIntersections() {
return (EList) eGet(Ifc4Package.Literals.IFC_GRID_AXIS__HAS_INTERSECTIONS, true);
}
/**
*
*
* @generated
*/
public void unsetHasIntersections() {
eUnset(Ifc4Package.Literals.IFC_GRID_AXIS__HAS_INTERSECTIONS);
}
/**
*
*
* @generated
*/
public boolean isSetHasIntersections() {
return eIsSet(Ifc4Package.Literals.IFC_GRID_AXIS__HAS_INTERSECTIONS);
}
} //IfcGridAxisImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy