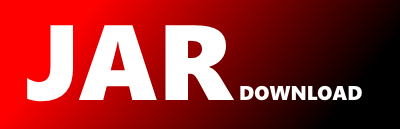
org.bimserver.models.ifc4.impl.IfcPersonImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pluginbase Show documentation
Show all versions of pluginbase Show documentation
Base project for BIMserver plugin development. Some plugins mights also need the Shared library
The newest version!
/**
* Copyright (C) 2009-2014 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.bimserver.models.ifc4.impl;
import org.bimserver.emf.IdEObjectImpl;
import org.bimserver.models.ifc4.Ifc4Package;
import org.bimserver.models.ifc4.IfcActorRole;
import org.bimserver.models.ifc4.IfcAddress;
import org.bimserver.models.ifc4.IfcPerson;
import org.bimserver.models.ifc4.IfcPersonAndOrganization;
import org.eclipse.emf.common.util.EList;
import org.eclipse.emf.ecore.EClass;
/**
*
* An implementation of the model object 'Ifc Person'.
*
*
* The following features are implemented:
*
*
* - {@link org.bimserver.models.ifc4.impl.IfcPersonImpl#getIdentification Identification}
* - {@link org.bimserver.models.ifc4.impl.IfcPersonImpl#getFamilyName Family Name}
* - {@link org.bimserver.models.ifc4.impl.IfcPersonImpl#getGivenName Given Name}
* - {@link org.bimserver.models.ifc4.impl.IfcPersonImpl#getMiddleNames Middle Names}
* - {@link org.bimserver.models.ifc4.impl.IfcPersonImpl#getPrefixTitles Prefix Titles}
* - {@link org.bimserver.models.ifc4.impl.IfcPersonImpl#getSuffixTitles Suffix Titles}
* - {@link org.bimserver.models.ifc4.impl.IfcPersonImpl#getRoles Roles}
* - {@link org.bimserver.models.ifc4.impl.IfcPersonImpl#getAddresses Addresses}
* - {@link org.bimserver.models.ifc4.impl.IfcPersonImpl#getEngagedIn Engaged In}
*
*
* @generated
*/
public class IfcPersonImpl extends IdEObjectImpl implements IfcPerson {
/**
*
*
* @generated
*/
protected IfcPersonImpl() {
super();
}
/**
*
*
* @generated
*/
@Override
protected EClass eStaticClass() {
return Ifc4Package.Literals.IFC_PERSON;
}
/**
*
*
* @generated
*/
@Override
protected int eStaticFeatureCount() {
return 0;
}
/**
*
*
* @generated
*/
public String getIdentification() {
return (String) eGet(Ifc4Package.Literals.IFC_PERSON__IDENTIFICATION, true);
}
/**
*
*
* @generated
*/
public void setIdentification(String newIdentification) {
eSet(Ifc4Package.Literals.IFC_PERSON__IDENTIFICATION, newIdentification);
}
/**
*
*
* @generated
*/
public void unsetIdentification() {
eUnset(Ifc4Package.Literals.IFC_PERSON__IDENTIFICATION);
}
/**
*
*
* @generated
*/
public boolean isSetIdentification() {
return eIsSet(Ifc4Package.Literals.IFC_PERSON__IDENTIFICATION);
}
/**
*
*
* @generated
*/
public String getFamilyName() {
return (String) eGet(Ifc4Package.Literals.IFC_PERSON__FAMILY_NAME, true);
}
/**
*
*
* @generated
*/
public void setFamilyName(String newFamilyName) {
eSet(Ifc4Package.Literals.IFC_PERSON__FAMILY_NAME, newFamilyName);
}
/**
*
*
* @generated
*/
public void unsetFamilyName() {
eUnset(Ifc4Package.Literals.IFC_PERSON__FAMILY_NAME);
}
/**
*
*
* @generated
*/
public boolean isSetFamilyName() {
return eIsSet(Ifc4Package.Literals.IFC_PERSON__FAMILY_NAME);
}
/**
*
*
* @generated
*/
public String getGivenName() {
return (String) eGet(Ifc4Package.Literals.IFC_PERSON__GIVEN_NAME, true);
}
/**
*
*
* @generated
*/
public void setGivenName(String newGivenName) {
eSet(Ifc4Package.Literals.IFC_PERSON__GIVEN_NAME, newGivenName);
}
/**
*
*
* @generated
*/
public void unsetGivenName() {
eUnset(Ifc4Package.Literals.IFC_PERSON__GIVEN_NAME);
}
/**
*
*
* @generated
*/
public boolean isSetGivenName() {
return eIsSet(Ifc4Package.Literals.IFC_PERSON__GIVEN_NAME);
}
/**
*
*
* @generated
*/
@SuppressWarnings("unchecked")
public EList getMiddleNames() {
return (EList) eGet(Ifc4Package.Literals.IFC_PERSON__MIDDLE_NAMES, true);
}
/**
*
*
* @generated
*/
public void unsetMiddleNames() {
eUnset(Ifc4Package.Literals.IFC_PERSON__MIDDLE_NAMES);
}
/**
*
*
* @generated
*/
public boolean isSetMiddleNames() {
return eIsSet(Ifc4Package.Literals.IFC_PERSON__MIDDLE_NAMES);
}
/**
*
*
* @generated
*/
@SuppressWarnings("unchecked")
public EList getPrefixTitles() {
return (EList) eGet(Ifc4Package.Literals.IFC_PERSON__PREFIX_TITLES, true);
}
/**
*
*
* @generated
*/
public void unsetPrefixTitles() {
eUnset(Ifc4Package.Literals.IFC_PERSON__PREFIX_TITLES);
}
/**
*
*
* @generated
*/
public boolean isSetPrefixTitles() {
return eIsSet(Ifc4Package.Literals.IFC_PERSON__PREFIX_TITLES);
}
/**
*
*
* @generated
*/
@SuppressWarnings("unchecked")
public EList getSuffixTitles() {
return (EList) eGet(Ifc4Package.Literals.IFC_PERSON__SUFFIX_TITLES, true);
}
/**
*
*
* @generated
*/
public void unsetSuffixTitles() {
eUnset(Ifc4Package.Literals.IFC_PERSON__SUFFIX_TITLES);
}
/**
*
*
* @generated
*/
public boolean isSetSuffixTitles() {
return eIsSet(Ifc4Package.Literals.IFC_PERSON__SUFFIX_TITLES);
}
/**
*
*
* @generated
*/
@SuppressWarnings("unchecked")
public EList getRoles() {
return (EList) eGet(Ifc4Package.Literals.IFC_PERSON__ROLES, true);
}
/**
*
*
* @generated
*/
public void unsetRoles() {
eUnset(Ifc4Package.Literals.IFC_PERSON__ROLES);
}
/**
*
*
* @generated
*/
public boolean isSetRoles() {
return eIsSet(Ifc4Package.Literals.IFC_PERSON__ROLES);
}
/**
*
*
* @generated
*/
@SuppressWarnings("unchecked")
public EList getAddresses() {
return (EList) eGet(Ifc4Package.Literals.IFC_PERSON__ADDRESSES, true);
}
/**
*
*
* @generated
*/
public void unsetAddresses() {
eUnset(Ifc4Package.Literals.IFC_PERSON__ADDRESSES);
}
/**
*
*
* @generated
*/
public boolean isSetAddresses() {
return eIsSet(Ifc4Package.Literals.IFC_PERSON__ADDRESSES);
}
/**
*
*
* @generated
*/
@SuppressWarnings("unchecked")
public EList getEngagedIn() {
return (EList) eGet(Ifc4Package.Literals.IFC_PERSON__ENGAGED_IN, true);
}
/**
*
*
* @generated
*/
public void unsetEngagedIn() {
eUnset(Ifc4Package.Literals.IFC_PERSON__ENGAGED_IN);
}
/**
*
*
* @generated
*/
public boolean isSetEngagedIn() {
return eIsSet(Ifc4Package.Literals.IFC_PERSON__ENGAGED_IN);
}
} //IfcPersonImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy