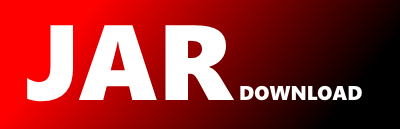
org.bimserver.models.ifc4.impl.IfcSectionReinforcementPropertiesImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pluginbase Show documentation
Show all versions of pluginbase Show documentation
Base project for BIMserver plugin development. Some plugins mights also need the Shared library
The newest version!
/**
* Copyright (C) 2009-2014 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.bimserver.models.ifc4.impl;
import org.bimserver.models.ifc4.Ifc4Package;
import org.bimserver.models.ifc4.IfcReinforcementBarProperties;
import org.bimserver.models.ifc4.IfcReinforcingBarRoleEnum;
import org.bimserver.models.ifc4.IfcSectionProperties;
import org.bimserver.models.ifc4.IfcSectionReinforcementProperties;
import org.eclipse.emf.common.util.EList;
import org.eclipse.emf.ecore.EClass;
/**
*
* An implementation of the model object 'Ifc Section Reinforcement Properties'.
*
*
* The following features are implemented:
*
*
* - {@link org.bimserver.models.ifc4.impl.IfcSectionReinforcementPropertiesImpl#getLongitudinalStartPosition Longitudinal Start Position}
* - {@link org.bimserver.models.ifc4.impl.IfcSectionReinforcementPropertiesImpl#getLongitudinalStartPositionAsString Longitudinal Start Position As String}
* - {@link org.bimserver.models.ifc4.impl.IfcSectionReinforcementPropertiesImpl#getLongitudinalEndPosition Longitudinal End Position}
* - {@link org.bimserver.models.ifc4.impl.IfcSectionReinforcementPropertiesImpl#getLongitudinalEndPositionAsString Longitudinal End Position As String}
* - {@link org.bimserver.models.ifc4.impl.IfcSectionReinforcementPropertiesImpl#getTransversePosition Transverse Position}
* - {@link org.bimserver.models.ifc4.impl.IfcSectionReinforcementPropertiesImpl#getTransversePositionAsString Transverse Position As String}
* - {@link org.bimserver.models.ifc4.impl.IfcSectionReinforcementPropertiesImpl#getReinforcementRole Reinforcement Role}
* - {@link org.bimserver.models.ifc4.impl.IfcSectionReinforcementPropertiesImpl#getSectionDefinition Section Definition}
* - {@link org.bimserver.models.ifc4.impl.IfcSectionReinforcementPropertiesImpl#getCrossSectionReinforcementDefinitions Cross Section Reinforcement Definitions}
*
*
* @generated
*/
public class IfcSectionReinforcementPropertiesImpl extends IfcPreDefinedPropertiesImpl
implements IfcSectionReinforcementProperties {
/**
*
*
* @generated
*/
protected IfcSectionReinforcementPropertiesImpl() {
super();
}
/**
*
*
* @generated
*/
@Override
protected EClass eStaticClass() {
return Ifc4Package.Literals.IFC_SECTION_REINFORCEMENT_PROPERTIES;
}
/**
*
*
* @generated
*/
public double getLongitudinalStartPosition() {
return (Double) eGet(Ifc4Package.Literals.IFC_SECTION_REINFORCEMENT_PROPERTIES__LONGITUDINAL_START_POSITION,
true);
}
/**
*
*
* @generated
*/
public void setLongitudinalStartPosition(double newLongitudinalStartPosition) {
eSet(Ifc4Package.Literals.IFC_SECTION_REINFORCEMENT_PROPERTIES__LONGITUDINAL_START_POSITION,
newLongitudinalStartPosition);
}
/**
*
*
* @generated
*/
public String getLongitudinalStartPositionAsString() {
return (String) eGet(
Ifc4Package.Literals.IFC_SECTION_REINFORCEMENT_PROPERTIES__LONGITUDINAL_START_POSITION_AS_STRING, true);
}
/**
*
*
* @generated
*/
public void setLongitudinalStartPositionAsString(String newLongitudinalStartPositionAsString) {
eSet(Ifc4Package.Literals.IFC_SECTION_REINFORCEMENT_PROPERTIES__LONGITUDINAL_START_POSITION_AS_STRING,
newLongitudinalStartPositionAsString);
}
/**
*
*
* @generated
*/
public double getLongitudinalEndPosition() {
return (Double) eGet(Ifc4Package.Literals.IFC_SECTION_REINFORCEMENT_PROPERTIES__LONGITUDINAL_END_POSITION,
true);
}
/**
*
*
* @generated
*/
public void setLongitudinalEndPosition(double newLongitudinalEndPosition) {
eSet(Ifc4Package.Literals.IFC_SECTION_REINFORCEMENT_PROPERTIES__LONGITUDINAL_END_POSITION,
newLongitudinalEndPosition);
}
/**
*
*
* @generated
*/
public String getLongitudinalEndPositionAsString() {
return (String) eGet(
Ifc4Package.Literals.IFC_SECTION_REINFORCEMENT_PROPERTIES__LONGITUDINAL_END_POSITION_AS_STRING, true);
}
/**
*
*
* @generated
*/
public void setLongitudinalEndPositionAsString(String newLongitudinalEndPositionAsString) {
eSet(Ifc4Package.Literals.IFC_SECTION_REINFORCEMENT_PROPERTIES__LONGITUDINAL_END_POSITION_AS_STRING,
newLongitudinalEndPositionAsString);
}
/**
*
*
* @generated
*/
public double getTransversePosition() {
return (Double) eGet(Ifc4Package.Literals.IFC_SECTION_REINFORCEMENT_PROPERTIES__TRANSVERSE_POSITION, true);
}
/**
*
*
* @generated
*/
public void setTransversePosition(double newTransversePosition) {
eSet(Ifc4Package.Literals.IFC_SECTION_REINFORCEMENT_PROPERTIES__TRANSVERSE_POSITION, newTransversePosition);
}
/**
*
*
* @generated
*/
public void unsetTransversePosition() {
eUnset(Ifc4Package.Literals.IFC_SECTION_REINFORCEMENT_PROPERTIES__TRANSVERSE_POSITION);
}
/**
*
*
* @generated
*/
public boolean isSetTransversePosition() {
return eIsSet(Ifc4Package.Literals.IFC_SECTION_REINFORCEMENT_PROPERTIES__TRANSVERSE_POSITION);
}
/**
*
*
* @generated
*/
public String getTransversePositionAsString() {
return (String) eGet(Ifc4Package.Literals.IFC_SECTION_REINFORCEMENT_PROPERTIES__TRANSVERSE_POSITION_AS_STRING,
true);
}
/**
*
*
* @generated
*/
public void setTransversePositionAsString(String newTransversePositionAsString) {
eSet(Ifc4Package.Literals.IFC_SECTION_REINFORCEMENT_PROPERTIES__TRANSVERSE_POSITION_AS_STRING,
newTransversePositionAsString);
}
/**
*
*
* @generated
*/
public void unsetTransversePositionAsString() {
eUnset(Ifc4Package.Literals.IFC_SECTION_REINFORCEMENT_PROPERTIES__TRANSVERSE_POSITION_AS_STRING);
}
/**
*
*
* @generated
*/
public boolean isSetTransversePositionAsString() {
return eIsSet(Ifc4Package.Literals.IFC_SECTION_REINFORCEMENT_PROPERTIES__TRANSVERSE_POSITION_AS_STRING);
}
/**
*
*
* @generated
*/
public IfcReinforcingBarRoleEnum getReinforcementRole() {
return (IfcReinforcingBarRoleEnum) eGet(
Ifc4Package.Literals.IFC_SECTION_REINFORCEMENT_PROPERTIES__REINFORCEMENT_ROLE, true);
}
/**
*
*
* @generated
*/
public void setReinforcementRole(IfcReinforcingBarRoleEnum newReinforcementRole) {
eSet(Ifc4Package.Literals.IFC_SECTION_REINFORCEMENT_PROPERTIES__REINFORCEMENT_ROLE, newReinforcementRole);
}
/**
*
*
* @generated
*/
public IfcSectionProperties getSectionDefinition() {
return (IfcSectionProperties) eGet(
Ifc4Package.Literals.IFC_SECTION_REINFORCEMENT_PROPERTIES__SECTION_DEFINITION, true);
}
/**
*
*
* @generated
*/
public void setSectionDefinition(IfcSectionProperties newSectionDefinition) {
eSet(Ifc4Package.Literals.IFC_SECTION_REINFORCEMENT_PROPERTIES__SECTION_DEFINITION, newSectionDefinition);
}
/**
*
*
* @generated
*/
@SuppressWarnings("unchecked")
public EList getCrossSectionReinforcementDefinitions() {
return (EList) eGet(
Ifc4Package.Literals.IFC_SECTION_REINFORCEMENT_PROPERTIES__CROSS_SECTION_REINFORCEMENT_DEFINITIONS,
true);
}
} //IfcSectionReinforcementPropertiesImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy