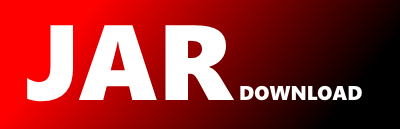
org.bimserver.models.store.DatabaseInformation Maven / Gradle / Ivy
Show all versions of pluginbase Show documentation
/**
* Copyright (C) 2009-2014 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.bimserver.models.store;
import java.util.Date;
import org.bimserver.emf.IdEObject;
import org.eclipse.emf.common.util.EList;
/**
*
* A representation of the model object 'Database Information'.
*
*
*
* The following features are supported:
*
*
* - {@link org.bimserver.models.store.DatabaseInformation#getNumberOfProjects Number Of Projects}
* - {@link org.bimserver.models.store.DatabaseInformation#getNumberOfUsers Number Of Users}
* - {@link org.bimserver.models.store.DatabaseInformation#getNumberOfRevisions Number Of Revisions}
* - {@link org.bimserver.models.store.DatabaseInformation#getNumberOfCheckouts Number Of Checkouts}
* - {@link org.bimserver.models.store.DatabaseInformation#getDatabaseSizeInBytes Database Size In Bytes}
* - {@link org.bimserver.models.store.DatabaseInformation#getType Type}
* - {@link org.bimserver.models.store.DatabaseInformation#getCreated Created}
* - {@link org.bimserver.models.store.DatabaseInformation#getLocation Location}
* - {@link org.bimserver.models.store.DatabaseInformation#getSchemaVersion Schema Version}
* - {@link org.bimserver.models.store.DatabaseInformation#getCategories Categories}
*
*
* @see org.bimserver.models.store.StorePackage#getDatabaseInformation()
* @model
* @extends IdEObject
* @generated
*/
public interface DatabaseInformation extends IdEObject {
/**
* Returns the value of the 'Number Of Projects' attribute.
*
*
* If the meaning of the 'Number Of Projects' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Number Of Projects' attribute.
* @see #setNumberOfProjects(Integer)
* @see org.bimserver.models.store.StorePackage#getDatabaseInformation_NumberOfProjects()
* @model
* @generated
*/
Integer getNumberOfProjects();
/**
* Sets the value of the '{@link org.bimserver.models.store.DatabaseInformation#getNumberOfProjects Number Of Projects}' attribute.
*
*
* @param value the new value of the 'Number Of Projects' attribute.
* @see #getNumberOfProjects()
* @generated
*/
void setNumberOfProjects(Integer value);
/**
* Returns the value of the 'Number Of Users' attribute.
*
*
* If the meaning of the 'Number Of Users' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Number Of Users' attribute.
* @see #setNumberOfUsers(Integer)
* @see org.bimserver.models.store.StorePackage#getDatabaseInformation_NumberOfUsers()
* @model
* @generated
*/
Integer getNumberOfUsers();
/**
* Sets the value of the '{@link org.bimserver.models.store.DatabaseInformation#getNumberOfUsers Number Of Users}' attribute.
*
*
* @param value the new value of the 'Number Of Users' attribute.
* @see #getNumberOfUsers()
* @generated
*/
void setNumberOfUsers(Integer value);
/**
* Returns the value of the 'Number Of Revisions' attribute.
*
*
* If the meaning of the 'Number Of Revisions' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Number Of Revisions' attribute.
* @see #setNumberOfRevisions(Integer)
* @see org.bimserver.models.store.StorePackage#getDatabaseInformation_NumberOfRevisions()
* @model
* @generated
*/
Integer getNumberOfRevisions();
/**
* Sets the value of the '{@link org.bimserver.models.store.DatabaseInformation#getNumberOfRevisions Number Of Revisions}' attribute.
*
*
* @param value the new value of the 'Number Of Revisions' attribute.
* @see #getNumberOfRevisions()
* @generated
*/
void setNumberOfRevisions(Integer value);
/**
* Returns the value of the 'Number Of Checkouts' attribute.
*
*
* If the meaning of the 'Number Of Checkouts' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Number Of Checkouts' attribute.
* @see #setNumberOfCheckouts(Integer)
* @see org.bimserver.models.store.StorePackage#getDatabaseInformation_NumberOfCheckouts()
* @model
* @generated
*/
Integer getNumberOfCheckouts();
/**
* Sets the value of the '{@link org.bimserver.models.store.DatabaseInformation#getNumberOfCheckouts Number Of Checkouts}' attribute.
*
*
* @param value the new value of the 'Number Of Checkouts' attribute.
* @see #getNumberOfCheckouts()
* @generated
*/
void setNumberOfCheckouts(Integer value);
/**
* Returns the value of the 'Database Size In Bytes' attribute.
*
*
* If the meaning of the 'Database Size In Bytes' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Database Size In Bytes' attribute.
* @see #setDatabaseSizeInBytes(Long)
* @see org.bimserver.models.store.StorePackage#getDatabaseInformation_DatabaseSizeInBytes()
* @model
* @generated
*/
Long getDatabaseSizeInBytes();
/**
* Sets the value of the '{@link org.bimserver.models.store.DatabaseInformation#getDatabaseSizeInBytes Database Size In Bytes}' attribute.
*
*
* @param value the new value of the 'Database Size In Bytes' attribute.
* @see #getDatabaseSizeInBytes()
* @generated
*/
void setDatabaseSizeInBytes(Long value);
/**
* Returns the value of the 'Type' attribute.
*
*
* If the meaning of the 'Type' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Type' attribute.
* @see #setType(String)
* @see org.bimserver.models.store.StorePackage#getDatabaseInformation_Type()
* @model
* @generated
*/
String getType();
/**
* Sets the value of the '{@link org.bimserver.models.store.DatabaseInformation#getType Type}' attribute.
*
*
* @param value the new value of the 'Type' attribute.
* @see #getType()
* @generated
*/
void setType(String value);
/**
* Returns the value of the 'Created' attribute.
*
*
* If the meaning of the 'Created' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Created' attribute.
* @see #setCreated(Date)
* @see org.bimserver.models.store.StorePackage#getDatabaseInformation_Created()
* @model
* @generated
*/
Date getCreated();
/**
* Sets the value of the '{@link org.bimserver.models.store.DatabaseInformation#getCreated Created}' attribute.
*
*
* @param value the new value of the 'Created' attribute.
* @see #getCreated()
* @generated
*/
void setCreated(Date value);
/**
* Returns the value of the 'Location' attribute.
*
*
* If the meaning of the 'Location' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Location' attribute.
* @see #setLocation(String)
* @see org.bimserver.models.store.StorePackage#getDatabaseInformation_Location()
* @model
* @generated
*/
String getLocation();
/**
* Sets the value of the '{@link org.bimserver.models.store.DatabaseInformation#getLocation Location}' attribute.
*
*
* @param value the new value of the 'Location' attribute.
* @see #getLocation()
* @generated
*/
void setLocation(String value);
/**
* Returns the value of the 'Schema Version' attribute.
*
*
* If the meaning of the 'Schema Version' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Schema Version' attribute.
* @see #setSchemaVersion(Integer)
* @see org.bimserver.models.store.StorePackage#getDatabaseInformation_SchemaVersion()
* @model
* @generated
*/
Integer getSchemaVersion();
/**
* Sets the value of the '{@link org.bimserver.models.store.DatabaseInformation#getSchemaVersion Schema Version}' attribute.
*
*
* @param value the new value of the 'Schema Version' attribute.
* @see #getSchemaVersion()
* @generated
*/
void setSchemaVersion(Integer value);
/**
* Returns the value of the 'Categories' reference list.
* The list contents are of type {@link org.bimserver.models.store.DatabaseInformationCategory}.
*
*
* If the meaning of the 'Categories' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Categories' reference list.
* @see org.bimserver.models.store.StorePackage#getDatabaseInformation_Categories()
* @model
* @generated
*/
EList getCategories();
} // DatabaseInformation