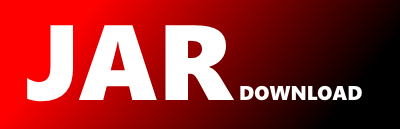
org.bimserver.models.store.IfcHeader Maven / Gradle / Ivy
Show all versions of pluginbase Show documentation
/**
* Copyright (C) 2009-2014 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.bimserver.models.store;
import java.util.Date;
import org.bimserver.emf.IdEObject;
import org.eclipse.emf.common.util.EList;
/**
*
* A representation of the model object 'Ifc Header'.
*
*
*
* The following features are supported:
*
*
* - {@link org.bimserver.models.store.IfcHeader#getDescription Description}
* - {@link org.bimserver.models.store.IfcHeader#getImplementationLevel Implementation Level}
* - {@link org.bimserver.models.store.IfcHeader#getFilename Filename}
* - {@link org.bimserver.models.store.IfcHeader#getTimeStamp Time Stamp}
* - {@link org.bimserver.models.store.IfcHeader#getAuthor Author}
* - {@link org.bimserver.models.store.IfcHeader#getOrganization Organization}
* - {@link org.bimserver.models.store.IfcHeader#getPreProcessorVersion Pre Processor Version}
* - {@link org.bimserver.models.store.IfcHeader#getOriginatingSystem Originating System}
* - {@link org.bimserver.models.store.IfcHeader#getIfcSchemaVersion Ifc Schema Version}
* - {@link org.bimserver.models.store.IfcHeader#getAuthorization Authorization}
*
*
* @see org.bimserver.models.store.StorePackage#getIfcHeader()
* @model
* @extends IdEObject
* @generated
*/
public interface IfcHeader extends IdEObject {
/**
* Returns the value of the 'Description' attribute list.
* The list contents are of type {@link java.lang.String}.
*
*
* If the meaning of the 'Description' attribute list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Description' attribute list.
* @see org.bimserver.models.store.StorePackage#getIfcHeader_Description()
* @model unique="false"
* @generated
*/
EList getDescription();
/**
* Returns the value of the 'Implementation Level' attribute.
*
*
* If the meaning of the 'Implementation Level' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Implementation Level' attribute.
* @see #setImplementationLevel(String)
* @see org.bimserver.models.store.StorePackage#getIfcHeader_ImplementationLevel()
* @model
* @generated
*/
String getImplementationLevel();
/**
* Sets the value of the '{@link org.bimserver.models.store.IfcHeader#getImplementationLevel Implementation Level}' attribute.
*
*
* @param value the new value of the 'Implementation Level' attribute.
* @see #getImplementationLevel()
* @generated
*/
void setImplementationLevel(String value);
/**
* Returns the value of the 'Filename' attribute.
*
*
* If the meaning of the 'Filename' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Filename' attribute.
* @see #setFilename(String)
* @see org.bimserver.models.store.StorePackage#getIfcHeader_Filename()
* @model
* @generated
*/
String getFilename();
/**
* Sets the value of the '{@link org.bimserver.models.store.IfcHeader#getFilename Filename}' attribute.
*
*
* @param value the new value of the 'Filename' attribute.
* @see #getFilename()
* @generated
*/
void setFilename(String value);
/**
* Returns the value of the 'Time Stamp' attribute.
*
*
* If the meaning of the 'Time Stamp' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Time Stamp' attribute.
* @see #setTimeStamp(Date)
* @see org.bimserver.models.store.StorePackage#getIfcHeader_TimeStamp()
* @model
* @generated
*/
Date getTimeStamp();
/**
* Sets the value of the '{@link org.bimserver.models.store.IfcHeader#getTimeStamp Time Stamp}' attribute.
*
*
* @param value the new value of the 'Time Stamp' attribute.
* @see #getTimeStamp()
* @generated
*/
void setTimeStamp(Date value);
/**
* Returns the value of the 'Author' attribute list.
* The list contents are of type {@link java.lang.String}.
*
*
* If the meaning of the 'Author' attribute list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Author' attribute list.
* @see org.bimserver.models.store.StorePackage#getIfcHeader_Author()
* @model unique="false"
* @generated
*/
EList getAuthor();
/**
* Returns the value of the 'Organization' attribute list.
* The list contents are of type {@link java.lang.String}.
*
*
* If the meaning of the 'Organization' attribute list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Organization' attribute list.
* @see org.bimserver.models.store.StorePackage#getIfcHeader_Organization()
* @model unique="false"
* @generated
*/
EList getOrganization();
/**
* Returns the value of the 'Pre Processor Version' attribute.
*
*
* If the meaning of the 'Pre Processor Version' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Pre Processor Version' attribute.
* @see #setPreProcessorVersion(String)
* @see org.bimserver.models.store.StorePackage#getIfcHeader_PreProcessorVersion()
* @model
* @generated
*/
String getPreProcessorVersion();
/**
* Sets the value of the '{@link org.bimserver.models.store.IfcHeader#getPreProcessorVersion Pre Processor Version}' attribute.
*
*
* @param value the new value of the 'Pre Processor Version' attribute.
* @see #getPreProcessorVersion()
* @generated
*/
void setPreProcessorVersion(String value);
/**
* Returns the value of the 'Originating System' attribute.
*
*
* If the meaning of the 'Originating System' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Originating System' attribute.
* @see #setOriginatingSystem(String)
* @see org.bimserver.models.store.StorePackage#getIfcHeader_OriginatingSystem()
* @model
* @generated
*/
String getOriginatingSystem();
/**
* Sets the value of the '{@link org.bimserver.models.store.IfcHeader#getOriginatingSystem Originating System}' attribute.
*
*
* @param value the new value of the 'Originating System' attribute.
* @see #getOriginatingSystem()
* @generated
*/
void setOriginatingSystem(String value);
/**
* Returns the value of the 'Ifc Schema Version' attribute.
*
*
* If the meaning of the 'Ifc Schema Version' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Ifc Schema Version' attribute.
* @see #setIfcSchemaVersion(String)
* @see org.bimserver.models.store.StorePackage#getIfcHeader_IfcSchemaVersion()
* @model
* @generated
*/
String getIfcSchemaVersion();
/**
* Sets the value of the '{@link org.bimserver.models.store.IfcHeader#getIfcSchemaVersion Ifc Schema Version}' attribute.
*
*
* @param value the new value of the 'Ifc Schema Version' attribute.
* @see #getIfcSchemaVersion()
* @generated
*/
void setIfcSchemaVersion(String value);
/**
* Returns the value of the 'Authorization' attribute.
*
*
* If the meaning of the 'Authorization' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Authorization' attribute.
* @see #setAuthorization(String)
* @see org.bimserver.models.store.StorePackage#getIfcHeader_Authorization()
* @model
* @generated
*/
String getAuthorization();
/**
* Sets the value of the '{@link org.bimserver.models.store.IfcHeader#getAuthorization Authorization}' attribute.
*
*
* @param value the new value of the 'Authorization' attribute.
* @see #getAuthorization()
* @generated
*/
void setAuthorization(String value);
} // IfcHeader