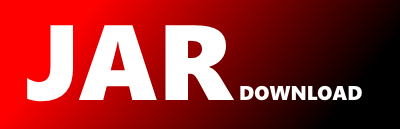
org.bimserver.models.store.Trigger Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pluginbase Show documentation
Show all versions of pluginbase Show documentation
Base project for BIMserver plugin development. Some plugins mights also need the Shared library
The newest version!
/**
* Copyright (C) 2009-2014 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.bimserver.models.store;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import org.eclipse.emf.common.util.Enumerator;
/**
*
* A representation of the literals of the enumeration 'Trigger',
* and utility methods for working with them.
*
* @see org.bimserver.models.store.StorePackage#getTrigger()
* @model
* @generated
*/
public enum Trigger implements Enumerator {
/**
* The 'NEW REVISION' literal object.
*
*
* @see #NEW_REVISION_VALUE
* @generated
* @ordered
*/
NEW_REVISION(0, "NEW_REVISION", "NEW_REVISION"),
/**
* The 'NEW PROJECT' literal object.
*
*
* @see #NEW_PROJECT_VALUE
* @generated
* @ordered
*/
NEW_PROJECT(1, "NEW_PROJECT", "NEW_PROJECT"),
/**
* The 'NEW EXTENDED DATA' literal object.
*
*
* @see #NEW_EXTENDED_DATA_VALUE
* @generated
* @ordered
*/
NEW_EXTENDED_DATA(2, "NEW_EXTENDED_DATA", "NEW_EXTENDED_DATA");
/**
* The 'NEW REVISION' literal value.
*
*
* If the meaning of 'NEW REVISION' literal object isn't clear,
* there really should be more of a description here...
*
*
* @see #NEW_REVISION
* @model
* @generated
* @ordered
*/
public static final int NEW_REVISION_VALUE = 0;
/**
* The 'NEW PROJECT' literal value.
*
*
* If the meaning of 'NEW PROJECT' literal object isn't clear,
* there really should be more of a description here...
*
*
* @see #NEW_PROJECT
* @model
* @generated
* @ordered
*/
public static final int NEW_PROJECT_VALUE = 1;
/**
* The 'NEW EXTENDED DATA' literal value.
*
*
* If the meaning of 'NEW EXTENDED DATA' literal object isn't clear,
* there really should be more of a description here...
*
*
* @see #NEW_EXTENDED_DATA
* @model
* @generated
* @ordered
*/
public static final int NEW_EXTENDED_DATA_VALUE = 2;
/**
* An array of all the 'Trigger' enumerators.
*
*
* @generated
*/
private static final Trigger[] VALUES_ARRAY = new Trigger[] { NEW_REVISION, NEW_PROJECT, NEW_EXTENDED_DATA, };
/**
* A public read-only list of all the 'Trigger' enumerators.
*
*
* @generated
*/
public static final List VALUES = Collections.unmodifiableList(Arrays.asList(VALUES_ARRAY));
/**
* Returns the 'Trigger' literal with the specified literal value.
*
*
* @param literal the literal.
* @return the matching enumerator or null
.
* @generated
*/
public static Trigger get(String literal) {
for (int i = 0; i < VALUES_ARRAY.length; ++i) {
Trigger result = VALUES_ARRAY[i];
if (result.toString().equals(literal)) {
return result;
}
}
return null;
}
/**
* Returns the 'Trigger' literal with the specified name.
*
*
* @param name the name.
* @return the matching enumerator or null
.
* @generated
*/
public static Trigger getByName(String name) {
for (int i = 0; i < VALUES_ARRAY.length; ++i) {
Trigger result = VALUES_ARRAY[i];
if (result.getName().equals(name)) {
return result;
}
}
return null;
}
/**
* Returns the 'Trigger' literal with the specified integer value.
*
*
* @param value the integer value.
* @return the matching enumerator or null
.
* @generated
*/
public static Trigger get(int value) {
switch (value) {
case NEW_REVISION_VALUE:
return NEW_REVISION;
case NEW_PROJECT_VALUE:
return NEW_PROJECT;
case NEW_EXTENDED_DATA_VALUE:
return NEW_EXTENDED_DATA;
}
return null;
}
/**
*
*
* @generated
*/
private final int value;
/**
*
*
* @generated
*/
private final String name;
/**
*
*
* @generated
*/
private final String literal;
/**
* Only this class can construct instances.
*
*
* @generated
*/
private Trigger(int value, String name, String literal) {
this.value = value;
this.name = name;
this.literal = literal;
}
/**
*
*
* @generated
*/
public int getValue() {
return value;
}
/**
*
*
* @generated
*/
public String getName() {
return name;
}
/**
*
*
* @generated
*/
public String getLiteral() {
return literal;
}
/**
* Returns the literal value of the enumerator, which is its string representation.
*
*
* @generated
*/
@Override
public String toString() {
return literal;
}
} //Trigger
© 2015 - 2025 Weber Informatics LLC | Privacy Policy