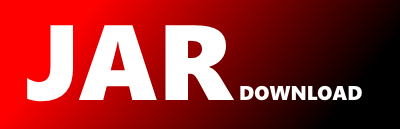
org.bimserver.models.store.User Maven / Gradle / Ivy
Show all versions of pluginbase Show documentation
/**
* Copyright (C) 2009-2014 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.bimserver.models.store;
import java.util.Date;
import org.bimserver.emf.IdEObject;
import org.bimserver.models.log.UserRelated;
import org.eclipse.emf.common.util.EList;
/**
*
* A representation of the model object 'User'.
*
*
*
* The following features are supported:
*
*
* - {@link org.bimserver.models.store.User#getName Name}
* - {@link org.bimserver.models.store.User#getPasswordHash Password Hash}
* - {@link org.bimserver.models.store.User#getPasswordSalt Password Salt}
* - {@link org.bimserver.models.store.User#getHasRightsOn Has Rights On}
* - {@link org.bimserver.models.store.User#getRevisions Revisions}
* - {@link org.bimserver.models.store.User#getState State}
* - {@link org.bimserver.models.store.User#getCreatedOn Created On}
* - {@link org.bimserver.models.store.User#getCreatedBy Created By}
* - {@link org.bimserver.models.store.User#getUserType User Type}
* - {@link org.bimserver.models.store.User#getUsername Username}
* - {@link org.bimserver.models.store.User#getLastSeen Last Seen}
* - {@link org.bimserver.models.store.User#getToken Token}
* - {@link org.bimserver.models.store.User#getValidationToken Validation Token}
* - {@link org.bimserver.models.store.User#getValidationTokenCreated Validation Token Created}
* - {@link org.bimserver.models.store.User#getUserSettings User Settings}
* - {@link org.bimserver.models.store.User#getSchemas Schemas}
* - {@link org.bimserver.models.store.User#getExtendedData Extended Data}
* - {@link org.bimserver.models.store.User#getServices Services}
* - {@link org.bimserver.models.store.User#getLogs Logs}
*
*
* @see org.bimserver.models.store.StorePackage#getUser()
* @model
* @extends IdEObject
* @generated
*/
public interface User extends IdEObject {
/**
* Returns the value of the 'Name' attribute.
*
*
* If the meaning of the 'Name' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Name' attribute.
* @see #setName(String)
* @see org.bimserver.models.store.StorePackage#getUser_Name()
* @model
* @generated
*/
String getName();
/**
* Sets the value of the '{@link org.bimserver.models.store.User#getName Name}' attribute.
*
*
* @param value the new value of the 'Name' attribute.
* @see #getName()
* @generated
*/
void setName(String value);
/**
* Returns the value of the 'Password Hash' attribute.
*
*
* If the meaning of the 'Password Hash' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Password Hash' attribute.
* @see #setPasswordHash(byte[])
* @see org.bimserver.models.store.StorePackage#getUser_PasswordHash()
* @model
* @generated
*/
byte[] getPasswordHash();
/**
* Sets the value of the '{@link org.bimserver.models.store.User#getPasswordHash Password Hash}' attribute.
*
*
* @param value the new value of the 'Password Hash' attribute.
* @see #getPasswordHash()
* @generated
*/
void setPasswordHash(byte[] value);
/**
* Returns the value of the 'Password Salt' attribute.
*
*
* If the meaning of the 'Password Salt' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Password Salt' attribute.
* @see #setPasswordSalt(byte[])
* @see org.bimserver.models.store.StorePackage#getUser_PasswordSalt()
* @model
* @generated
*/
byte[] getPasswordSalt();
/**
* Sets the value of the '{@link org.bimserver.models.store.User#getPasswordSalt Password Salt}' attribute.
*
*
* @param value the new value of the 'Password Salt' attribute.
* @see #getPasswordSalt()
* @generated
*/
void setPasswordSalt(byte[] value);
/**
* Returns the value of the 'Has Rights On' reference list.
* The list contents are of type {@link org.bimserver.models.store.Project}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.store.Project#getHasAuthorizedUsers Has Authorized Users}'.
*
*
* If the meaning of the 'Has Rights On' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Has Rights On' reference list.
* @see org.bimserver.models.store.StorePackage#getUser_HasRightsOn()
* @see org.bimserver.models.store.Project#getHasAuthorizedUsers
* @model opposite="hasAuthorizedUsers"
* @generated
*/
EList getHasRightsOn();
/**
* Returns the value of the 'Revisions' reference list.
* The list contents are of type {@link org.bimserver.models.store.Revision}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.store.Revision#getUser User}'.
*
*
* If the meaning of the 'Revisions' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Revisions' reference list.
* @see org.bimserver.models.store.StorePackage#getUser_Revisions()
* @see org.bimserver.models.store.Revision#getUser
* @model opposite="user"
* @generated
*/
EList getRevisions();
/**
* Returns the value of the 'State' attribute.
* The literals are from the enumeration {@link org.bimserver.models.store.ObjectState}.
*
*
* If the meaning of the 'State' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'State' attribute.
* @see org.bimserver.models.store.ObjectState
* @see #setState(ObjectState)
* @see org.bimserver.models.store.StorePackage#getUser_State()
* @model
* @generated
*/
ObjectState getState();
/**
* Sets the value of the '{@link org.bimserver.models.store.User#getState State}' attribute.
*
*
* @param value the new value of the 'State' attribute.
* @see org.bimserver.models.store.ObjectState
* @see #getState()
* @generated
*/
void setState(ObjectState value);
/**
* Returns the value of the 'Created On' attribute.
*
*
* If the meaning of the 'Created On' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Created On' attribute.
* @see #setCreatedOn(Date)
* @see org.bimserver.models.store.StorePackage#getUser_CreatedOn()
* @model
* @generated
*/
Date getCreatedOn();
/**
* Sets the value of the '{@link org.bimserver.models.store.User#getCreatedOn Created On}' attribute.
*
*
* @param value the new value of the 'Created On' attribute.
* @see #getCreatedOn()
* @generated
*/
void setCreatedOn(Date value);
/**
* Returns the value of the 'Created By' reference.
*
*
* If the meaning of the 'Created By' reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Created By' reference.
* @see #setCreatedBy(User)
* @see org.bimserver.models.store.StorePackage#getUser_CreatedBy()
* @model
* @generated
*/
User getCreatedBy();
/**
* Sets the value of the '{@link org.bimserver.models.store.User#getCreatedBy Created By}' reference.
*
*
* @param value the new value of the 'Created By' reference.
* @see #getCreatedBy()
* @generated
*/
void setCreatedBy(User value);
/**
* Returns the value of the 'User Type' attribute.
* The literals are from the enumeration {@link org.bimserver.models.store.UserType}.
*
*
* If the meaning of the 'User Type' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'User Type' attribute.
* @see org.bimserver.models.store.UserType
* @see #setUserType(UserType)
* @see org.bimserver.models.store.StorePackage#getUser_UserType()
* @model
* @generated
*/
UserType getUserType();
/**
* Sets the value of the '{@link org.bimserver.models.store.User#getUserType User Type}' attribute.
*
*
* @param value the new value of the 'User Type' attribute.
* @see org.bimserver.models.store.UserType
* @see #getUserType()
* @generated
*/
void setUserType(UserType value);
/**
* Returns the value of the 'Username' attribute.
*
*
* If the meaning of the 'Username' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Username' attribute.
* @see #setUsername(String)
* @see org.bimserver.models.store.StorePackage#getUser_Username()
* @model
* @generated
*/
String getUsername();
/**
* Sets the value of the '{@link org.bimserver.models.store.User#getUsername Username}' attribute.
*
*
* @param value the new value of the 'Username' attribute.
* @see #getUsername()
* @generated
*/
void setUsername(String value);
/**
* Returns the value of the 'Last Seen' attribute.
*
*
* If the meaning of the 'Last Seen' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Last Seen' attribute.
* @see #setLastSeen(Date)
* @see org.bimserver.models.store.StorePackage#getUser_LastSeen()
* @model
* @generated
*/
Date getLastSeen();
/**
* Sets the value of the '{@link org.bimserver.models.store.User#getLastSeen Last Seen}' attribute.
*
*
* @param value the new value of the 'Last Seen' attribute.
* @see #getLastSeen()
* @generated
*/
void setLastSeen(Date value);
/**
* Returns the value of the 'Token' attribute.
*
*
* If the meaning of the 'Token' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Token' attribute.
* @see #setToken(String)
* @see org.bimserver.models.store.StorePackage#getUser_Token()
* @model
* @generated
*/
String getToken();
/**
* Sets the value of the '{@link org.bimserver.models.store.User#getToken Token}' attribute.
*
*
* @param value the new value of the 'Token' attribute.
* @see #getToken()
* @generated
*/
void setToken(String value);
/**
* Returns the value of the 'Validation Token' attribute.
*
*
* If the meaning of the 'Validation Token' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Validation Token' attribute.
* @see #setValidationToken(byte[])
* @see org.bimserver.models.store.StorePackage#getUser_ValidationToken()
* @model
* @generated
*/
byte[] getValidationToken();
/**
* Sets the value of the '{@link org.bimserver.models.store.User#getValidationToken Validation Token}' attribute.
*
*
* @param value the new value of the 'Validation Token' attribute.
* @see #getValidationToken()
* @generated
*/
void setValidationToken(byte[] value);
/**
* Returns the value of the 'Validation Token Created' attribute.
*
*
* If the meaning of the 'Validation Token Created' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Validation Token Created' attribute.
* @see #setValidationTokenCreated(Date)
* @see org.bimserver.models.store.StorePackage#getUser_ValidationTokenCreated()
* @model
* @generated
*/
Date getValidationTokenCreated();
/**
* Sets the value of the '{@link org.bimserver.models.store.User#getValidationTokenCreated Validation Token Created}' attribute.
*
*
* @param value the new value of the 'Validation Token Created' attribute.
* @see #getValidationTokenCreated()
* @generated
*/
void setValidationTokenCreated(Date value);
/**
* Returns the value of the 'User Settings' reference.
*
*
* If the meaning of the 'User Settings' reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'User Settings' reference.
* @see #setUserSettings(UserSettings)
* @see org.bimserver.models.store.StorePackage#getUser_UserSettings()
* @model
* @generated
*/
UserSettings getUserSettings();
/**
* Sets the value of the '{@link org.bimserver.models.store.User#getUserSettings User Settings}' reference.
*
*
* @param value the new value of the 'User Settings' reference.
* @see #getUserSettings()
* @generated
*/
void setUserSettings(UserSettings value);
/**
* Returns the value of the 'Schemas' reference list.
* The list contents are of type {@link org.bimserver.models.store.ExtendedDataSchema}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.store.ExtendedDataSchema#getUsers Users}'.
*
*
* If the meaning of the 'Schemas' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Schemas' reference list.
* @see org.bimserver.models.store.StorePackage#getUser_Schemas()
* @see org.bimserver.models.store.ExtendedDataSchema#getUsers
* @model opposite="users"
* @generated
*/
EList getSchemas();
/**
* Returns the value of the 'Extended Data' reference list.
* The list contents are of type {@link org.bimserver.models.store.ExtendedData}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.store.ExtendedData#getUser User}'.
*
*
* If the meaning of the 'Extended Data' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Extended Data' reference list.
* @see org.bimserver.models.store.StorePackage#getUser_ExtendedData()
* @see org.bimserver.models.store.ExtendedData#getUser
* @model opposite="user"
* @generated
*/
EList getExtendedData();
/**
* Returns the value of the 'Services' reference list.
* The list contents are of type {@link org.bimserver.models.store.Service}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.store.Service#getUser User}'.
*
*
* If the meaning of the 'Services' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Services' reference list.
* @see org.bimserver.models.store.StorePackage#getUser_Services()
* @see org.bimserver.models.store.Service#getUser
* @model opposite="user"
* @generated
*/
EList getServices();
/**
* Returns the value of the 'Logs' reference list.
* The list contents are of type {@link org.bimserver.models.log.UserRelated}.
* It is bidirectional and its opposite is '{@link org.bimserver.models.log.UserRelated#getUser User}'.
*
*
* If the meaning of the 'Logs' reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Logs' reference list.
* @see org.bimserver.models.store.StorePackage#getUser_Logs()
* @see org.bimserver.models.log.UserRelated#getUser
* @model opposite="user"
* @generated
*/
EList getLogs();
} // User