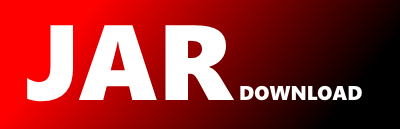
org.bimserver.database.queries.om.QueryPart Maven / Gradle / Ivy
package org.bimserver.database.queries.om;
/******************************************************************************
* Copyright (C) 2009-2016 BIMserver.org
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see {@literal }.
*****************************************************************************/
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.bimserver.emf.PackageMetaData;
import org.eclipse.emf.ecore.EClass;
public class QueryPart extends PartOfQuery implements CanInclude {
private List types;
private List oids;
private Set guids;
private Map properties;
private PackageMetaData packageMetaData;
private InBoundingBox inBoundingBox;
private List includes;
private boolean includeAllFields;
public QueryPart(PackageMetaData packageMetaData) {
this.packageMetaData = packageMetaData;
}
public void addType(EClass type, boolean includeAllSubTypes) {
if (types == null) {
types = new ArrayList<>();
}
types.add(type);
if (includeAllSubTypes) {
types.addAll(packageMetaData.getAllSubClasses(type));
}
}
public void addOid(long oid) {
if (oids == null) {
oids = new ArrayList<>();
}
oids.add(oid);
}
public void addGuid(String guid) {
if (guids == null) {
guids = new HashSet<>();
}
guids.add(guid);
}
public List getTypes() {
return types;
}
public List getOids() {
return oids;
}
public Set getGuids() {
return guids;
}
public Map getProperties() {
return properties;
}
public void addProperty(String key, boolean value) {
if (this.properties == null) {
this.properties = new HashMap();
}
this.properties.put(key, value);
}
public void setInBoundingBox(InBoundingBox inBoundingBox) {
this.inBoundingBox = inBoundingBox;
}
public InBoundingBox getInBoundingBox() {
return inBoundingBox;
}
public boolean isIncludeAllFields() {
return includeAllFields;
}
public void setIncludeAllFields(boolean includeAllFields) {
this.includeAllFields = includeAllFields;
}
@Override
public void addInclude(Include include) {
if (includes == null) {
includes = new ArrayList();
}
includes.add(include);
}
public List getIncludes() {
return includes;
}
public boolean hasIncludes() {
return includes != null;
}
public boolean hasOids() {
return oids != null;
}
public boolean hasTypes() {
return types != null;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
return sb.toString();
}
public void dump(int indent, StringBuilder sb) {
if (indent > 10) {
sb.append("..trimmed\n");
return;
}
if (hasTypes()) {
sb.append(indent(indent) + "types\n");
for (EClass type : getTypes()) {
sb.append(indent(indent + 1) + type.getName() + "\n");
}
}
if (hasOids()) {
sb.append(indent(indent) + "oids\n");
for (long oid : getOids()) {
sb.append(indent(indent + 1) + oid + "\n");
}
}
if (hasIncludes()) {
sb.append(indent(indent) + "includes\n");
for (Include include : getIncludes()) {
include.dump(indent + 1, sb);
}
}
}
public Include createInclude() {
Include include = new Include(packageMetaData);
addInclude(include);
return include;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy