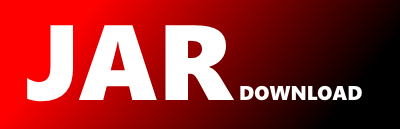
org.openstreetmap.atlas.utilities.collections.Maps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of atlas Show documentation
Show all versions of atlas Show documentation
"Library to load OSM data into an Atlas format"
package org.openstreetmap.atlas.utilities.collections;
import java.util.HashMap;
import java.util.Map;
import org.openstreetmap.atlas.exception.CoreException;
/**
* @author matthieun
*/
public final class Maps
{
/**
* Return a {@link HashMap} from a even number of keys and values of the same type
*
* @param items
* a even number of keys and values of the same type
* @param
* The type for the map
* @return A {@link HashMap} translated from the keys and values in items
*/
@SafeVarargs
public static Map hashMap(final T... items)
{
if (items.length % 2 != 0)
{
throw new CoreException("Needs to have an even number of arguments");
}
final Map result = new HashMap<>();
for (int i = 0; i < items.length; i += 2)
{
result.put(items[i], items[i + 1]);
}
return result;
}
public static Map stringMap(final String... items)
{
return hashMap(items);
}
@SafeVarargs
public static Map withMaps(final boolean rejectCollisions,
final Map... items)
{
if (items.length == 0)
{
return new HashMap<>();
}
if (items.length == 1)
{
return items[0];
}
final Map result = new HashMap<>();
for (final Map item : items)
{
for (final Map.Entry entry : item.entrySet())
{
if (rejectCollisions && result.containsKey(entry.getKey()))
{
throw new CoreException("Cannot merge maps! Collision on key.");
}
result.put(entry.getKey(), entry.getValue());
}
}
return result;
}
@SafeVarargs
public static Map withMaps(final Map... items)
{
return withMaps(true, items);
}
private Maps()
{
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy