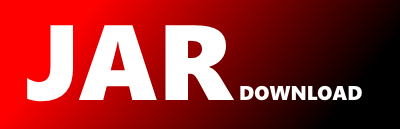
org.jhotdraw.samples.mini.SelectionToolSample Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jhotdraw Show documentation
Show all versions of jhotdraw Show documentation
JHotDraw 7 with openTCS-specific modifications
The newest version!
/*
* @(#)SelectionToolSample.java
*
* Copyright (c) 1996-2010 by the original authors of JHotDraw and all its
* contributors. All rights reserved.
*
* You may not use, copy or modify this file, except in compliance with the
* license agreement you entered into with the copyright holders. For details
* see accompanying license terms.
*/
package org.jhotdraw.samples.mini;
import org.jhotdraw.draw.tool.SelectionTool;
import java.awt.geom.*;
import javax.swing.*;
import org.jhotdraw.draw.*;
/**
* SelectionToolSample demonstrates how the SelectionTool
works.
*
* Internally, the SelectionTool
uses three smaller tools
* (named as 'Tracker') to fulfill its task. If the user presses the mouse
* button over an empty area of a drawing, the SelectAreaTracker
* comes into action. If the user presses the mouse button over a figure, the
* DragTracker
comes into action. If the user presses the mouse
* button over a handle, the HandleTracker
comes into action.
*
* You need to edit the source code as marked below.
*
* With this program you can:
*
* - See how the
SelectionTool
interacts with a
* LineFigure
.
* - See how the
SelectAreaTracker
interacts with a
* LineFigure
.
* - See how the
DragTracker
interacts with a
* LineFigure
.
* - See how the
HandleTracker
interacts with a
* LineFigure
.
*
*
*
* @author Pondus
* @version $Id: SelectionToolSample.java 718 2010-11-21 17:49:53Z rawcoder $
*/
public class SelectionToolSample {
/**
* Creates a new instance of SelectionToolSample
*/
public SelectionToolSample() {
LineFigure lf = new LineFigure();
lf.setBounds(new Point2D.Double(40, 40), new Point2D.Double(200,
40));
// Add all figures to a drawing
Drawing drawing = new DefaultDrawing();
drawing.add(lf);
// Show the drawing
JFrame f = new JFrame("UltraMini");
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.setSize(600, 300);
DrawingView view = new DefaultDrawingView();
view.setDrawing(drawing);
f.getContentPane().add(view.getComponent());
// set up the drawing editor
DrawingEditor editor = new DefaultDrawingEditor();
editor.add(view);
// Activate the following line to see the SelectionTool in full
// action.
editor.setTool(new SelectionTool());
// Activate the following line to only see the SelectAreaTracker in
// action.
//editor.setTool(new SelectAreaTracker());
// Activate the following line to only see the DragTracker in
// action.
//editor.setTool(new DragTracker(lf));
// Activate the following lines to only see the HandleTracker in
// action.
//view.selectAll();
//editor.setTool(new HandleTracker(view.findHandle(view.drawingToView(lf.getStartPoint()))));
f.setVisible(true);
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
new SelectionToolSample();
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy