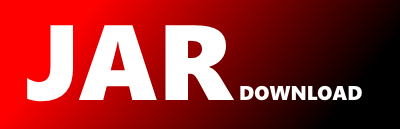
org.opentcs.drivers.peripherals.management.PeripheralAttachmentInformation Maven / Gradle / Ivy
/**
* Copyright (c) The openTCS Authors.
*
* This program is free software and subject to the MIT license. (For details,
* see the licensing information (LICENSE.txt) you should have received with
* this copy of the software.)
*/
package org.opentcs.drivers.peripherals.management;
import static java.util.Objects.requireNonNull;
import jakarta.annotation.Nonnull;
import java.io.Serializable;
import java.util.List;
import org.opentcs.data.model.Location;
import org.opentcs.data.model.TCSResourceReference;
import org.opentcs.drivers.peripherals.PeripheralCommAdapterDescription;
/**
* Describes which communication adapter a location is currently associated with.
*/
public class PeripheralAttachmentInformation
implements
Serializable {
/**
* The location this attachment information belongs to.
*/
private final TCSResourceReference locationReference;
/**
* The list of comm adapters available to be attached to the referenced location.
*/
private final List availableCommAdapters;
/**
* The comm adapter attached to the referenced location.
*/
private final PeripheralCommAdapterDescription attachedCommAdapter;
/**
* Creates a new instance.
*
* @param locationReference The location this attachment information belongs to.
* @param availableCommAdapters The list of comm adapters available to be attached to the
* referenced location.
* @param attachedCommAdapter The comm adapter attached to the referenced location.
*/
public PeripheralAttachmentInformation(
@Nonnull
TCSResourceReference locationReference,
@Nonnull
List availableCommAdapters,
@Nonnull
PeripheralCommAdapterDescription attachedCommAdapter
) {
this.locationReference = requireNonNull(locationReference, "locationReference");
this.availableCommAdapters = requireNonNull(availableCommAdapters, "availableCommAdapters");
this.attachedCommAdapter = requireNonNull(attachedCommAdapter, "attachedCommAdapter");
}
/**
* Returns the location this attachment information belongs to.
*
* @return The location this attachment information belongs to.
*/
@Nonnull
public TCSResourceReference getLocationReference() {
return locationReference;
}
/**
* Creates a copy of this object with the given location reference.
*
* @param locationReference The new location reference.
* @return A copy of this object, differing in the given location reference.
*/
public PeripheralAttachmentInformation withLocationReference(
TCSResourceReference locationReference
) {
return new PeripheralAttachmentInformation(
locationReference,
availableCommAdapters,
attachedCommAdapter
);
}
/**
* Returns the list of comm adapters available to be attached to the referenced location.
*
* @return The list of comm adapters available to be attached to the referenced location.
*/
@Nonnull
public List getAvailableCommAdapters() {
return availableCommAdapters;
}
/**
* Creates a copy of this object with the given available comm adapters.
*
* @param availableCommAdapters The new available comm adapters.
* @return A copy of this object, differing in the given available comm adapters.
*/
public PeripheralAttachmentInformation withAvailableCommAdapters(
@Nonnull
List availableCommAdapters
) {
return new PeripheralAttachmentInformation(
locationReference,
availableCommAdapters,
attachedCommAdapter
);
}
/**
* Returns the comm adapter attached to the referenced location.
*
* @return The comm adapter attached to the referenced location.
*/
@Nonnull
public PeripheralCommAdapterDescription getAttachedCommAdapter() {
return attachedCommAdapter;
}
/**
* Creates a copy of this object with the given attached comm adapter.
*
* @param attachedCommAdapter The new attached comm adapter.
* @return A copy of this object, differing in the given attached comm adapter.
*/
public PeripheralAttachmentInformation withAttachedCommAdapter(
@Nonnull
PeripheralCommAdapterDescription attachedCommAdapter
) {
return new PeripheralAttachmentInformation(
locationReference,
availableCommAdapters,
attachedCommAdapter
);
}
@Override
public String toString() {
return "PeripheralAttachmentInformation{"
+ "locationReference=" + locationReference
+ ", availableCommAdapters=" + availableCommAdapters
+ ", attachedCommAdapter=" + attachedCommAdapter
+ '}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy