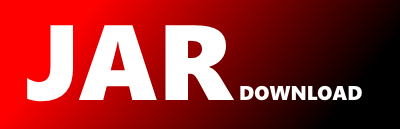
org.opentcs.kernelcontrolcenter.AboutDialog Maven / Gradle / Ivy
The newest version!
// SPDX-FileCopyrightText: The openTCS Authors
// SPDX-License-Identifier: MIT
package org.opentcs.kernelcontrolcenter;
import java.awt.Frame;
import javax.swing.JDialog;
import org.opentcs.util.Environment;
/**
* An about dialog.
*/
public class AboutDialog
extends
JDialog {
/**
* Creates new AboutDialog.
*
* @param parent The parent frame.
* @param modal Whether the dialog blocks user input to other top-level windows when shown.
*/
@SuppressWarnings("this-escape")
public AboutDialog(Frame parent, boolean modal) {
super(parent, modal);
initComponents();
}
// FORMATTER:OFF
// CHECKSTYLE:OFF
/**
* This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
// //GEN-BEGIN:initComponents
private void initComponents() {
java.awt.GridBagConstraints gridBagConstraints;
logoPanel = new javax.swing.JPanel();
opentcsLogoLbl = new javax.swing.JLabel();
contactPanel = new javax.swing.JPanel();
opentcsContactPanel = new javax.swing.JPanel();
opentcsLbl = new javax.swing.JLabel();
versionLbl = new javax.swing.JLabel();
versionTxtLbl = new javax.swing.JLabel();
customVersionLbl = new javax.swing.JLabel();
customVersionTxtLbl = new javax.swing.JLabel();
homepageLbl = new javax.swing.JLabel();
homepageTxtLbl = new javax.swing.JLabel();
emailLbl = new javax.swing.JLabel();
emailTxtLbl = new javax.swing.JLabel();
imlPanel = new javax.swing.JPanel();
fraunhoferImlLbl = new javax.swing.JLabel();
homepageImlLbl = new javax.swing.JLabel();
homepageImlTxtLbl = new javax.swing.JLabel();
fillingLbl = new javax.swing.JLabel();
closeButton = new javax.swing.JButton();
fillingLbl2 = new javax.swing.JLabel();
setDefaultCloseOperation(javax.swing.WindowConstants.DISPOSE_ON_CLOSE);
java.util.ResourceBundle bundle = java.util.ResourceBundle.getBundle("i18n/org/opentcs/kernelcontrolcenter/Bundle"); // NOI18N
setTitle(bundle.getString("aboutDialog.title")); // NOI18N
setResizable(false);
getContentPane().setLayout(new java.awt.GridBagLayout());
logoPanel.setBackground(new java.awt.Color(255, 255, 255));
logoPanel.setLayout(new java.awt.BorderLayout());
opentcsLogoLbl.setHorizontalAlignment(javax.swing.SwingConstants.CENTER);
opentcsLogoLbl.setIcon(new javax.swing.ImageIcon(getClass().getResource("/org/opentcs/kernelcontrolcenter/res/logos/opentcs.gif"))); // NOI18N
logoPanel.add(opentcsLogoLbl, java.awt.BorderLayout.CENTER);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.ipadx = 6;
gridBagConstraints.ipady = 6;
getContentPane().add(logoPanel, gridBagConstraints);
contactPanel.setLayout(new javax.swing.BoxLayout(contactPanel, javax.swing.BoxLayout.Y_AXIS));
opentcsContactPanel.setLayout(new java.awt.GridBagLayout());
opentcsLbl.setFont(opentcsLbl.getFont().deriveFont(opentcsLbl.getFont().getStyle() | java.awt.Font.BOLD));
opentcsLbl.setText("open Transportation Control System");
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridwidth = 2;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.anchor = java.awt.GridBagConstraints.WEST;
gridBagConstraints.insets = new java.awt.Insets(6, 0, 0, 0);
opentcsContactPanel.add(opentcsLbl, gridBagConstraints);
versionLbl.setText(bundle.getString("aboutDialog.label_baselineVersion.text")); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 1;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
opentcsContactPanel.add(versionLbl, gridBagConstraints);
versionTxtLbl.setText(Environment.getBaselineVersion());
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 1;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.insets = new java.awt.Insets(0, 6, 0, 0);
opentcsContactPanel.add(versionTxtLbl, gridBagConstraints);
customVersionLbl.setText(bundle.getString("aboutDialog.label_customVersion.text")); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 2;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
opentcsContactPanel.add(customVersionLbl, gridBagConstraints);
customVersionTxtLbl.setText(Environment.getCustomizationName() + " " + Environment.getCustomizationVersion());
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 2;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.insets = new java.awt.Insets(0, 6, 0, 0);
opentcsContactPanel.add(customVersionTxtLbl, gridBagConstraints);
homepageLbl.setText(bundle.getString("aboutDialog.label_homepage.text")); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 3;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
opentcsContactPanel.add(homepageLbl, gridBagConstraints);
homepageTxtLbl.setText("https://www.opentcs.org/");
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 3;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.insets = new java.awt.Insets(0, 6, 0, 0);
opentcsContactPanel.add(homepageTxtLbl, gridBagConstraints);
emailLbl.setText(bundle.getString("aboutDialog.label_email.text")); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 4;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
opentcsContactPanel.add(emailLbl, gridBagConstraints);
emailTxtLbl.setText("[email protected]");
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 4;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.insets = new java.awt.Insets(0, 6, 0, 0);
opentcsContactPanel.add(emailTxtLbl, gridBagConstraints);
contactPanel.add(opentcsContactPanel);
imlPanel.setLayout(new java.awt.GridBagLayout());
fraunhoferImlLbl.setFont(fraunhoferImlLbl.getFont().deriveFont(fraunhoferImlLbl.getFont().getStyle() | java.awt.Font.BOLD));
fraunhoferImlLbl.setText(bundle.getString("aboutDialog.label_fraunhoferIml.text")); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 0;
gridBagConstraints.gridwidth = 2;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.anchor = java.awt.GridBagConstraints.WEST;
gridBagConstraints.insets = new java.awt.Insets(6, 0, 0, 0);
imlPanel.add(fraunhoferImlLbl, gridBagConstraints);
homepageImlLbl.setText(bundle.getString("aboutDialog.label_homepage.text")); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 1;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
imlPanel.add(homepageImlLbl, gridBagConstraints);
homepageImlTxtLbl.setText("http://www.iml.fraunhofer.de/");
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 1;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.insets = new java.awt.Insets(0, 6, 0, 0);
imlPanel.add(homepageImlTxtLbl, gridBagConstraints);
contactPanel.add(imlPanel);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 1;
gridBagConstraints.fill = java.awt.GridBagConstraints.BOTH;
getContentPane().add(contactPanel, gridBagConstraints);
fillingLbl.setText(" ");
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 2;
gridBagConstraints.fill = java.awt.GridBagConstraints.BOTH;
gridBagConstraints.weighty = 1.0;
getContentPane().add(fillingLbl, gridBagConstraints);
closeButton.setText(bundle.getString("aboutDialog.button_close.text")); // NOI18N
closeButton.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
closeButtonActionPerformed(evt);
}
});
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 3;
getContentPane().add(closeButton, gridBagConstraints);
fillingLbl2.setText(" ");
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 4;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.weighty = 1.0;
getContentPane().add(fillingLbl2, gridBagConstraints);
pack();
}// //GEN-END:initComponents
// CHECKSTYLE:ON
// FORMATTER:ON
private void closeButtonActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_closeButtonActionPerformed
this.setVisible(false);
}//GEN-LAST:event_closeButtonActionPerformed
// FORMATTER:OFF
// CHECKSTYLE:OFF
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton closeButton;
private javax.swing.JPanel contactPanel;
private javax.swing.JLabel customVersionLbl;
private javax.swing.JLabel customVersionTxtLbl;
private javax.swing.JLabel emailLbl;
private javax.swing.JLabel emailTxtLbl;
private javax.swing.JLabel fillingLbl;
private javax.swing.JLabel fillingLbl2;
private javax.swing.JLabel fraunhoferImlLbl;
private javax.swing.JLabel homepageImlLbl;
private javax.swing.JLabel homepageImlTxtLbl;
private javax.swing.JLabel homepageLbl;
private javax.swing.JLabel homepageTxtLbl;
private javax.swing.JPanel imlPanel;
private javax.swing.JPanel logoPanel;
private javax.swing.JPanel opentcsContactPanel;
private javax.swing.JLabel opentcsLbl;
private javax.swing.JLabel opentcsLogoLbl;
private javax.swing.JLabel versionLbl;
private javax.swing.JLabel versionTxtLbl;
// End of variables declaration//GEN-END:variables
// CHECKSTYLE:ON
// FORMATTER:ON
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy