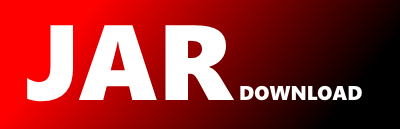
org.opentcs.guing.common.components.dialogs.StandardContentDialog Maven / Gradle / Ivy
/**
* Copyright (c) The openTCS Authors.
*
* This program is free software and subject to the MIT license. (For details,
* see the licensing information (LICENSE.txt) you should have received with
* this copy of the software.)
*/
package org.opentcs.guing.common.components.dialogs;
import java.awt.BorderLayout;
import java.awt.Component;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JComponent;
import javax.swing.JOptionPane;
import javax.swing.border.EmptyBorder;
/**
* A standard dialog with an OK and a cancel button.
*/
public class StandardContentDialog
extends
javax.swing.JDialog
implements
InputValidationListener {
/**
* A return status code - returned if Cancel button has been pressed.
*/
public static final int RET_CANCEL = 0;
/**
* A return status code - returned if OK button has been pressed.
*/
public static final int RET_OK = 1;
/**
* Button configuration for an OK and a cancel button.
*/
public static final int OK_CANCEL = 10;
/**
* Button configuration for an OK, cancel and apply button.
*/
public static final int OK_CANCEL_APPLY = 11;
/**
* Button configuration for a close button.
*/
public static final int CLOSE = 12;
/**
* Button configuration user-defined.
*/
public static final int USER_DEFINED = 13;
/**
* Content for this dialog.
*/
protected DialogContent fContent;
/**
* The return status.
*/
private int returnStatus = RET_CANCEL;
/**
* Creates new instance.
*/
public StandardContentDialog(Component parent, DialogContent content) {
this(parent, content, true, OK_CANCEL);
}
/**
* Creates new form StandardDialog.
*
* @param parent The parent component on which this dialog is centered.
* @param content The content.
* @param modal whether or not this dialog is modal.
* @param options Which user interface options to use.
*/
@SuppressWarnings("this-escape")
public StandardContentDialog(
Component parent,
DialogContent content,
boolean modal,
int options
) {
super(JOptionPane.getFrameForComponent(parent), modal);
initComponents();
initButtons(options);
JComponent component = content.getComponent();
if (component.getBorder() == null) {
component.setBorder(new EmptyBorder(4, 4, 4, 4));
}
getContentPane().add(component, BorderLayout.CENTER);
setTitle(content.getDialogTitle());
content.initFields();
pack();
setLocationRelativeTo(parent);
fContent = content;
getRootPane().setDefaultButton(okButton);
}
@Override
public void inputValidationSuccessful(boolean success) {
this.okButton.setEnabled(success);
}
/**
* Returns the returns status code.
*
* @return the return status of this dialog - one of RET_OK or RET_CANCEL
*/
public int getReturnStatus() {
return returnStatus;
}
/**
* Adds a user-defined button.
*
* @param text The text for the button.
* @param returnStatus The return value when the button is pressed.
*/
public void addUserDefinedButton(String text, final int returnStatus) {
JButton button = new JButton(text);
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent evt) {
doClose(returnStatus);
}
});
buttonPanel.add(button);
}
/**
* Event of the dialog content that the dialog can be closed.
*/
public void requestClose() {
doClose(RET_CANCEL);
}
protected final void initButtons(int options) {
switch (options) {
case OK_CANCEL:
applyButton.setVisible(false);
closeButton.setVisible(false);
break;
case OK_CANCEL_APPLY:
closeButton.setVisible(false);
break;
case CLOSE:
okButton.setVisible(false);
cancelButton.setVisible(false);
applyButton.setVisible(false);
break;
case USER_DEFINED:
okButton.setVisible(false);
cancelButton.setVisible(false);
applyButton.setVisible(false);
closeButton.setVisible(false);
break;
default:
}
}
// FORMATTER:OFF
// CHECKSTYLE:OFF
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
// //GEN-BEGIN:initComponents
private void initComponents() {
buttonPanel = new javax.swing.JPanel();
okButton = new javax.swing.JButton();
cancelButton = new CancelButton();
applyButton = new javax.swing.JButton();
closeButton = new javax.swing.JButton();
addWindowListener(new java.awt.event.WindowAdapter() {
public void windowClosing(java.awt.event.WindowEvent evt) {
closeDialog(evt);
}
});
buttonPanel.setBorder(javax.swing.BorderFactory.createEmptyBorder(0, 0, 0, 5));
buttonPanel.setLayout(new java.awt.FlowLayout(java.awt.FlowLayout.CENTER, 10, 5));
okButton.setFont(okButton.getFont().deriveFont(okButton.getFont().getStyle() | java.awt.Font.BOLD));
java.util.ResourceBundle bundle = java.util.ResourceBundle.getBundle("i18n/org/opentcs/plantoverview/system"); // NOI18N
okButton.setText(bundle.getString("standardContentDialog.button_ok.text")); // NOI18N
okButton.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
okButtonActionPerformed(evt);
}
});
buttonPanel.add(okButton);
cancelButton.setFont(cancelButton.getFont());
cancelButton.setText(bundle.getString("standardContentDialog.button_cancel.text")); // NOI18N
cancelButton.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
cancelButtonActionPerformed(evt);
}
});
buttonPanel.add(cancelButton);
applyButton.setFont(applyButton.getFont());
applyButton.setText(bundle.getString("standardContentDialog.button_apply.text")); // NOI18N
applyButton.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
applyButtonActionPerformed(evt);
}
});
buttonPanel.add(applyButton);
closeButton.setFont(closeButton.getFont());
closeButton.setText(bundle.getString("standardContentDialog.button_close.text")); // NOI18N
closeButton.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
closeButtonActionPerformed(evt);
}
});
buttonPanel.add(closeButton);
getContentPane().add(buttonPanel, java.awt.BorderLayout.SOUTH);
pack();
}// //GEN-END:initComponents
// CHECKSTYLE:ON
// FORMATTER:ON
/**
* Button "close" pressed.
*
* @param evt The action event.
*/
private void closeButtonActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_closeButtonActionPerformed
doClose(RET_CANCEL);
}//GEN-LAST:event_closeButtonActionPerformed
/**
* Button "apply" pressed.
*
* @param evt The action event.
*/
private void applyButtonActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_applyButtonActionPerformed
fContent.update();
}//GEN-LAST:event_applyButtonActionPerformed
/**
* Button "Ok" pressed.
*
* @param evt The action event.
*/
private void okButtonActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_okButtonActionPerformed
fContent.update();
if (!fContent.updateFailed()) {
doClose(RET_OK);
}
}//GEN-LAST:event_okButtonActionPerformed
/**
* Button "cancel" pressed.
*
* @param evt The action event.
*/
private void cancelButtonActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_cancelButtonActionPerformed
doClose(RET_CANCEL);
}//GEN-LAST:event_cancelButtonActionPerformed
/**
* Closes the dialog
*/
private void closeDialog(java.awt.event.WindowEvent evt) {//GEN-FIRST:event_closeDialog
doClose(RET_CANCEL);
}//GEN-LAST:event_closeDialog
/**
* Closes the dialog.
*
* @param retStatus The return status code.
*/
private void doClose(int retStatus) {
returnStatus = retStatus;
setVisible(false);
dispose();
}
// FORMATTER:OFF
// CHECKSTYLE:OFF
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton applyButton;
private javax.swing.JPanel buttonPanel;
private javax.swing.JButton cancelButton;
private javax.swing.JButton closeButton;
private javax.swing.JButton okButton;
// End of variables declaration//GEN-END:variables
// CHECKSTYLE:ON
// FORMATTER:ON
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy