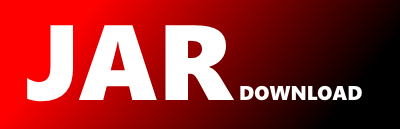
org.opentripplanner.graph_builder.module.shapefile.CompositeStreetTraversalPermissionConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of otp Show documentation
Show all versions of otp Show documentation
The OpenTripPlanner multimodal journey planning system
package org.opentripplanner.graph_builder.module.shapefile;
import java.util.ArrayList;
import java.util.Collection;
import org.opengis.feature.simple.SimpleFeature;
import org.opentripplanner.common.model.P2;
import org.opentripplanner.graph_builder.services.shapefile.SimpleFeatureConverter;
import org.opentripplanner.routing.edgetype.StreetTraversalPermission;
/**
* A converter which is a composite of other converters. It can combine them with an "and" or "or"
* strategy. The orPermissions variable controls that.
*
* @author rob
*
*/
public class CompositeStreetTraversalPermissionConverter implements SimpleFeatureConverter> {
private Collection>> converters = new ArrayList>>();
private boolean orPermissions = false;
public CompositeStreetTraversalPermissionConverter() {
}
/**
* Is the or combination strategy being used?
*
* @return whether the or combination strategy is used
*/
public boolean isOrPermissions() {
return orPermissions;
}
public void setOrPermissions(boolean orPermissions) {
this.orPermissions = orPermissions;
}
/**
* set the list of converters used to the passed in parameter
*
* @param converters
* list of converters to use
*/
public void setConverters(
Collection>> converters) {
this.converters = converters;
}
/**
* use the permission combination strategy to combine the results of the list of converters
*/
@Override
public P2 convert(SimpleFeature feature) {
P2 result = null;
for (SimpleFeatureConverter> converter : converters) {
P2 value = converter.convert(feature);
if (result == null) {
result = value;
} else {
StreetTraversalPermission first, second;
if (orPermissions) {
first = result.first.add(value.first);
second = result.second.add(value.second);
} else {
first = StreetTraversalPermission.get(result.first.code & value.first.code);
second = StreetTraversalPermission.get(result.second.code & value.second.code);
}
result = new P2(first, second);
}
}
return result;
}
/**
* add a converter to the list to be applied
* @param converter the new converter
*/
public void add(SimpleFeatureConverter> converter) {
converters.add(converter);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy