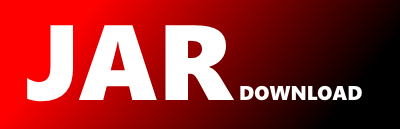
org.opentripplanner.gtfs.mapping.RouteMapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of otp Show documentation
Show all versions of otp Show documentation
The OpenTripPlanner multimodal journey planning system
package org.opentripplanner.gtfs.mapping;
import org.opentripplanner.model.Route;
import org.opentripplanner.util.MapUtils;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
/** Responsible for mapping GTFS Route into the OTP model. */
class RouteMapper {
private final AgencyMapper agencyMapper;
private final Map mappedRoutes = new HashMap<>();
RouteMapper(AgencyMapper agencyMapper) {
this.agencyMapper = agencyMapper;
}
Collection map(Collection agencies) {
return MapUtils.mapToList(agencies, this::map);
}
/** Map from GTFS to OTP model, {@code null} safe. */
Route map(org.onebusaway.gtfs.model.Route orginal) {
return orginal == null ? null : mappedRoutes.computeIfAbsent(orginal, this::doMap);
}
private Route doMap(org.onebusaway.gtfs.model.Route rhs) {
Route lhs = new Route();
lhs.setId(AgencyAndIdMapper.mapAgencyAndId(rhs.getId()));
lhs.setAgency(agencyMapper.map(rhs.getAgency()));
lhs.setShortName(rhs.getShortName());
lhs.setLongName(rhs.getLongName());
lhs.setType(rhs.getType());
lhs.setMode(TransitModeMapper.mapMode(rhs.getType()));
lhs.setDesc(rhs.getDesc());
lhs.setUrl(rhs.getUrl());
lhs.setColor(rhs.getColor());
lhs.setTextColor(rhs.getTextColor());
lhs.setRouteBikesAllowed(rhs.getRouteBikesAllowed());
lhs.setBikesAllowed(rhs.getBikesAllowed());
lhs.setSortOrder(rhs.getSortOrder());
lhs.setBrandingUrl(rhs.getBrandingUrl());
return lhs;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy