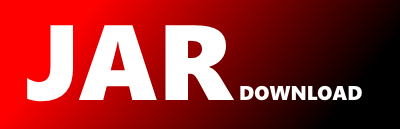
org.opentripplanner.gtfs.mapping.StopTimeMapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of otp Show documentation
Show all versions of otp Show documentation
The OpenTripPlanner multimodal journey planning system
package org.opentripplanner.gtfs.mapping;
import org.opentripplanner.model.StopTime;
import org.opentripplanner.util.MapUtils;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
/**
* Responsible for mapping GTFS StopTime into the OTP Transit model.
*/
class StopTimeMapper {
private final StopMapper stopMapper;
private final TripMapper tripMapper;
private Map mappedStopTimes = new HashMap<>();
StopTimeMapper(StopMapper stopMapper, TripMapper tripMapper) {
this.stopMapper = stopMapper;
this.tripMapper = tripMapper;
}
Collection map(Collection times) {
return MapUtils.mapToList(times, this::map);
}
/** Map from GTFS to OTP model, {@code null} safe. */
StopTime map(org.onebusaway.gtfs.model.StopTime orginal) {
return orginal == null ? null : mappedStopTimes.computeIfAbsent(orginal, this::doMap);
}
private StopTime doMap(org.onebusaway.gtfs.model.StopTime rhs) {
StopTime lhs = new StopTime();
lhs.setTrip(tripMapper.map(rhs.getTrip()));
lhs.setStop(stopMapper.map(rhs.getStop()));
lhs.setArrivalTime(rhs.getArrivalTime());
lhs.setDepartureTime(rhs.getDepartureTime());
lhs.setTimepoint(rhs.getTimepoint());
lhs.setStopSequence(rhs.getStopSequence());
lhs.setStopHeadsign(rhs.getStopHeadsign());
lhs.setRouteShortName(rhs.getRouteShortName());
lhs.setPickupType(rhs.getPickupType());
lhs.setDropOffType(rhs.getDropOffType());
lhs.setShapeDistTraveled(rhs.getShapeDistTraveled());
lhs.setFarePeriodId(rhs.getFarePeriodId());
// Skip mapping of proxy
// private transient StopTimeProxy proxy;
if (rhs.getProxy() != null) {
throw new IllegalStateException("Did not expect proxy to be set!");
}
return lhs;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy