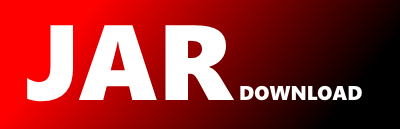
org.opentripplanner.util.MapUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of otp Show documentation
Show all versions of otp Show documentation
The OpenTripPlanner multimodal journey planning system
package org.opentripplanner.util;
import gnu.trove.map.TLongObjectMap;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.Function;
import java.util.stream.Collectors;
public class MapUtils {
public static final void addToMapSet(TLongObjectMap> mapSet, long key, U value) {
Set set = mapSet.get(key);
if (set == null) {
set = new HashSet();
mapSet.put(key, set);
}
set.add(value);
}
public static final void addToMapList(Map> mapList, T key, U value) {
List list = mapList.get(key);
if (list == null) {
list = new ArrayList();
mapList.put(key, list);
}
list.add(value);
}
public static final boolean addToMaxMap(Map map, T key, double value) {
Double oldValue = map.get(key);
if (oldValue == null || value > oldValue) {
map.put(key, value);
return true;
}
return false;
}
public static void addToMapListUnique(Map> mapList,
T key, List values) {
List list = mapList.get(key);
if (list == null) {
list = new ArrayList(values.size());
mapList.put(key, list);
}
for (U value : values) {
if (!list.contains(value)) {
list.add(value);
}
}
}
public static > void mergeInUnique(Map mapList,
Map from) {
for (Map.Entry entry : from.entrySet()) {
T key = entry.getKey();
V value = entry.getValue();
V originalValue = mapList.get(key);
if (originalValue != null) {
HashSet originalSet = new HashSet(originalValue);
for (U item : value) {
if (!originalSet.contains(item))
originalValue.add(item);
}
} else {
mapList.put(key, value);
}
}
}
/**
* Map a collection of objects of type S to a list of type T using the
* provided mapping function.
*
* Nullsafe: if entities is null
, then null
is returned.
*/
public static List mapToList(Collection entities, Function mapper) {
return entities == null ? null : entities.stream().map(mapper).collect(Collectors.toList());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy