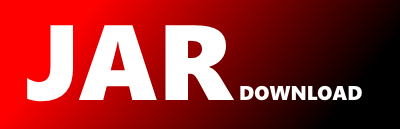
org.opentripplanner.netex.index.hierarchy.HierarchicalMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of otp Show documentation
Show all versions of otp Show documentation
The OpenTripPlanner multimodal journey planning system
package org.opentripplanner.netex.index.hierarchy;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
/**
* A concrete implementation of {@link AbstractHierarchicalMap}.
*
* @param the key type
* @param the value type
*/
public class HierarchicalMap extends AbstractHierarchicalMap {
private final Map map = new HashMap<>();
/** Create a new hierarchical map root. */
public HierarchicalMap() {
super(null);
}
/** Create new child map with the given {@code parent}. */
public HierarchicalMap(HierarchicalMap parent) {
super(parent);
}
/** Return a reference to the parent. */
@Override
public HierarchicalMap parent() {
return (HierarchicalMap) super.parent();
}
@Override
V localGet(K key) {
return map.get(key);
}
@Override
boolean localContainsKey(K key) {
return map.containsKey(key);
}
@Override
protected int localSize() {
return map.size();
}
@Override
void localRemove(K key) {
map.remove(key);
}
/**
* Add a new pair of {@code key & value} to the local map instance.
*/
public void add(K key, V value) {
map.put(key, value);
}
/**
* Add a set of {@code keys & values} to the local map instance.
*/
public void addAll(Map other) {
for (Map.Entry e : other.entrySet()) {
add(e.getKey(), e.getValue());
}
}
/** @return return all keys in the local map */
@Override
public Collection localKeys() {
return map.keySet();
}
/**
* @return a collection of all values hold in the local map, all values added to one of the
* parents are excluded from the collection.
*/
@Override
public Collection localValues() {
return map.values();
}
@Override
public boolean localIsEmpty() {
return map.isEmpty();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy