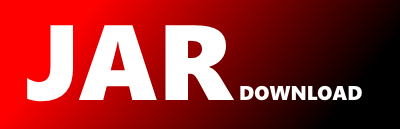
org.opentripplanner.netex.loader.NetexXmlParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of otp Show documentation
Show all versions of otp Show documentation
The OpenTripPlanner multimodal journey planning system
package org.opentripplanner.netex.loader;
import java.io.InputStream;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Unmarshaller;
import org.rutebanken.netex.model.PublicationDeliveryStructure;
/** Simple wrapper to perform typesafe xml parsing and simple error handling. */
public class NetexXmlParser {
/** used to parse the XML. */
private final Unmarshaller unmarshaller;
public NetexXmlParser() {
this.unmarshaller = createUnmarshaller();
}
/**
* Parse an input stream and return the root document type for the given xml file (stream).
*/
public PublicationDeliveryStructure parseXmlDoc(InputStream stream) throws JAXBException {
JAXBElement root;
//noinspection unchecked
root = (JAXBElement) unmarshaller.unmarshal(stream);
return root.getValue();
}
/** factory method for unmarshaller */
private static Unmarshaller createUnmarshaller() {
try {
return JAXBContext.newInstance(PublicationDeliveryStructure.class).createUnmarshaller();
} catch (JAXBException e) {
// This is a programming error - not expected!
// We abort early and also allow for this to happen in the constructor;
// Which in other cases would be considered bad practice.
throw new RuntimeException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy