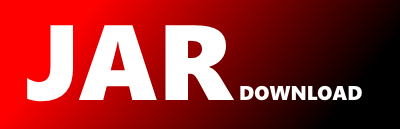
org.openurp.edu.clazz.service.internal.CourseLimitServiceImpl Maven / Gradle / Ivy
/*
* OpenURP, Agile University Resource Planning Solution.
*
* Copyright © 2014, The OpenURP Software.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful.
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package org.openurp.edu.clazz.service.internal;
import org.beangle.commons.collection.CollectUtils;
import org.beangle.commons.dao.impl.BaseServiceImpl;
import org.beangle.commons.entity.Entity;
import org.beangle.commons.lang.Strings;
import org.beangle.commons.lang.tuple.Pair;
import org.openurp.code.edu.model.EducationType;
import org.openurp.code.std.model.StdType;
import org.openurp.base.edu.model.Direction;
import org.openurp.base.edu.model.Major;
import org.openurp.base.std.model.Squad;
import org.openurp.base.std.model.Student;
import org.openurp.base.model.Department;
import org.openurp.code.edu.model.CourseTakeType;
import org.openurp.code.edu.model.EducationLevel;
import org.openurp.code.edu.model.ElectionMode;
import org.openurp.code.person.model.Gender;
import org.openurp.edu.clazz.model.*;
import org.openurp.edu.clazz.service.ClazzNameStrategy;
import org.openurp.edu.clazz.service.CourseLimitService;
import org.openurp.edu.clazz.service.RestrictionBuilder;
import java.util.*;
@SuppressWarnings("unchecked")
public class CourseLimitServiceImpl extends BaseServiceImpl implements CourseLimitService {
ClazzNameStrategy teachclassNameStrategy;
@Deprecated
public void mergeAll(Enrollment target, Enrollment source) {
// TODO
}
@Deprecated
public void merge(Long mergeType, Enrollment target, Enrollment source) {
if (ClazzRestrictionMeta.Squad.equals(mergeType)) {
Set tmp_collection = new HashSet();
List targetCollection = extractSquades(target);
if (CollectUtils.isNotEmpty(targetCollection)) {
tmp_collection.addAll(targetCollection);
}
List sourceCollection = extractSquades(source);
if (CollectUtils.isNotEmpty(sourceCollection)) {
tmp_collection.addAll(sourceCollection);
}
limitEnrollment(true, target, tmp_collection.toArray(new Squad[0]));
} else if (ClazzRestrictionMeta.Department.equals(mergeType)) {
Set tmp_collection = new HashSet();
List targetCollection = extractAttendDeparts(target);
if (CollectUtils.isNotEmpty(targetCollection)) {
tmp_collection.addAll(targetCollection);
}
List sourceCollection = extractAttendDeparts(source);
if (CollectUtils.isNotEmpty(sourceCollection)) {
tmp_collection.addAll(sourceCollection);
}
limitEnrollment(true, target, tmp_collection.toArray(new Department[0]));
} else if (ClazzRestrictionMeta.StdType.equals(mergeType)) {
Set tmp_collection = new HashSet();
List targetCollection = extractStdTypes(target);
if (CollectUtils.isNotEmpty(targetCollection)) {
tmp_collection.addAll(targetCollection);
}
List sourceCollection = extractStdTypes(source);
if (CollectUtils.isNotEmpty(sourceCollection)) {
tmp_collection.addAll(sourceCollection);
}
limitEnrollment(true, target, tmp_collection.toArray(new StdType[0]));
} else if (ClazzRestrictionMeta.Direction.equals(mergeType)) {
Set tmp_collection = new HashSet();
List targetCollection = extractDirections(target);
if (CollectUtils.isNotEmpty(targetCollection)) {
tmp_collection.addAll(targetCollection);
}
List sourceCollection = extractDirections(source);
if (CollectUtils.isNotEmpty(sourceCollection)) {
tmp_collection.addAll(sourceCollection);
}
limitEnrollment(true, target, tmp_collection.toArray(new Direction[0]));
} else if (ClazzRestrictionMeta.Gender.equals(mergeType)) {
Gender targetGender = extractGender(target);
Gender sourceGender = extractGender(source);
Gender tmp_gender = null;
if (targetGender != null && sourceGender != null) {
if (targetGender.equals(sourceGender)) {
tmp_gender = targetGender;
}
}
if (targetGender != null && sourceGender == null) {
tmp_gender = targetGender;
}
if (targetGender == null && sourceGender != null) {
tmp_gender = sourceGender;
}
if (tmp_gender != null) {
limitEnrollment(true, target, tmp_gender);
}
} else if (ClazzRestrictionMeta.Grade.equals(mergeType)) {
String tmp_grade = "";
String targetGrade = extractGrade(target);
if (Strings.isNotBlank(targetGrade)) {
tmp_grade = targetGrade;
}
String sourceGrade = extractGrade(source);
if (Strings.isNotBlank(sourceGrade)) {
tmp_grade += "," + sourceGrade;
}
String[] grades = Strings.split(tmp_grade);
if (grades.length > 0) {
limitEnrollment(true, target, grades);
}
} else if (ClazzRestrictionMeta.Major.equals(mergeType)) {
Set tmp_collection = new HashSet();
List targetCollection = extractMajors(target);
if (CollectUtils.isNotEmpty(targetCollection)) {
tmp_collection.addAll(targetCollection);
}
List sourceCollection = extractMajors(source);
if (CollectUtils.isNotEmpty(sourceCollection)) {
tmp_collection.addAll(sourceCollection);
}
limitEnrollment(true, target, tmp_collection.toArray(new Major[0]));
} else if (ClazzRestrictionMeta.Level.equals(mergeType)) {
Set tmp_collection = new HashSet();
List targetCollection = extractEducations(target);
if (CollectUtils.isNotEmpty(targetCollection)) {
tmp_collection.addAll(targetCollection);
}
List sourceCollection = extractEducations(source);
if (CollectUtils.isNotEmpty(sourceCollection)) {
tmp_collection.addAll(sourceCollection);
}
limitEnrollment(true, target, tmp_collection.toArray(new EducationLevel[0]));
} else {
throw new RuntimeException("unsupported limit meta merge");
}
}
public List extractEducations(Enrollment teachclass) {
Map>> res = xtractEducationLimit(teachclass);
List levels = CollectUtils.newArrayList();
for (Pair> tmpRes : res.values()) {
if (tmpRes._1) {
for (EducationLevel level : tmpRes._2) {
if (!levels.contains(level)) {
levels.add(level);
}
}
}
}
return levels;
}
public List extractEducations(ClazzRestriction group) {
Pair> res = xtractEducationLimit(group);
if (res._1) {
return res._2;
}
return CollectUtils.newArrayList();
}
public List extractSquades(Enrollment teachclass) {
Map>> res = xtractSquadLimit(teachclass);
List squades = CollectUtils.newArrayList();
for (Pair> tmpRes : res.values()) {
if (tmpRes._1) {
for (Squad squad : tmpRes._2) {
if (!squades.contains(squad)) {
squades.add(squad);
}
}
}
}
return squades;
}
public List extractSquades(ClazzRestriction group) {
Pair> res = xtractSquadLimit(group);
if (res._1) {
return res._2;
}
return CollectUtils.newArrayList();
}
public String extractGrade(Enrollment teachclass) {
Map>> res = xtractGradeLimit(teachclass);
Set grades = CollectUtils.newHashSet();
for (Pair> tmpRes : res.values()) {
if (tmpRes._1) {
grades.addAll(tmpRes._2);
}
}
String grade = "";
for (String str : grades) {
if (Strings.isNotEmpty(grade)) {
grade += ",";
}
grade += str;
}
return grade;
}
public String extractGrade(ClazzRestriction group) {
Pair> res = xtractGradeLimit(group);
if (res._1) {
if (CollectUtils.isNotEmpty(res._2)) {
return res._2.get(0);
}
}
return null;
}
public List extractStdTypes(Enrollment teachclass) {
Map>> res = xtractStdTypeLimit(teachclass);
List stdTypes = CollectUtils.newArrayList();
for (Pair> tmpRes : res.values()) {
if (tmpRes._1) {
for (StdType stdType : tmpRes._2) {
if (!stdTypes.contains(stdType)) {
stdTypes.add(stdType);
}
}
}
}
return stdTypes;
}
public List extractStdTypes(ClazzRestriction group) {
Pair> res = xtractStdTypeLimit(group);
if (res._1) {
return res._2;
}
return CollectUtils.newArrayList();
}
public List extractMajors(Enrollment teachclass) {
Map>> res = xtractMajorLimit(teachclass);
List majors = CollectUtils.newArrayList();
for (Pair> tmpRes : res.values()) {
if (tmpRes._1) {
for (Major major : tmpRes._2) {
if (!majors.contains(major)) {
majors.add(major);
}
}
}
}
return majors;
}
public List extractMajors(ClazzRestriction group) {
Pair> res = xtractMajorLimit(group);
if (res._1) {
return res._2;
}
return CollectUtils.newArrayList();
}
public List extractDirections(Enrollment teachclass) {
Map>> res = xtractDirectionLimit(teachclass);
List directions = CollectUtils.newArrayList();
for (Pair> tmpRes : res.values()) {
if (tmpRes._1) {
for (Direction direction : tmpRes._2) {
if (!directions.contains(direction)) {
directions.add(direction);
}
}
}
}
return directions;
}
public List extractDirections(ClazzRestriction group) {
Pair> res = xtractDirectionLimit(group);
if (res._1) {
return res._2;
}
return CollectUtils.newArrayList();
}
public List extractAttendDeparts(Enrollment teachclass) {
Map>> res = xtractAttendDepartLimit(teachclass);
List departments = CollectUtils.newArrayList();
for (Pair> tmpRes : res.values()) {
if (tmpRes._1) {
for (Department department : tmpRes._2) {
if (!departments.contains(department)) {
departments.add(department);
}
}
}
}
return departments;
}
public List extractAttendDeparts(ClazzRestriction group) {
Pair> res = xtractAttendDepartLimit(group);
if (res._1) {
return res._2;
}
return CollectUtils.newArrayList();
}
@Deprecated
public Gender extractGender(Enrollment teachclass) {
List groups = teachclass.getRestrictions();
for (ClazzRestriction group : groups) {
if (!group.isPrime()) {
continue;
}
for (ClazzRestrictionItem item : group.getItems()) {
if (ClazzRestrictionMeta.Gender.equals(item.getMeta()) && item.isIncluded()) {
return entityDao.get(Gender.class, Strings.splitToInt(item.getContents()))
.get(0);
}
}
}
return null;
}
@Deprecated
public Gender extractGender(ClazzRestriction group) {
if (group == null) {
return null;
}
for (ClazzRestrictionItem item : group.getItems()) {
if (ClazzRestrictionMeta.Gender.equals(item.getMeta()) && item.isIncluded()) {
return entityDao.get(Gender.class, Strings.splitToInt(item.getContents()))
.get(0);
}
}
return null;
}
public RestrictionBuilder builder() {
return new DefaultRestrictionBuilder();
}
private RestrictionBuilder builder(ClazzRestriction group) {
return new DefaultRestrictionBuilder(group);
}
public RestrictionBuilder builder(Enrollment teachclass) {
Enrollment clb = (Enrollment) teachclass;
ClazzRestriction group = clb.getOrCreateDefautRestriction();
return new DefaultRestrictionBuilder(group);
}
public Set extractLonelyTakers(Enrollment teachclass) {
Set takers = teachclass.getCourseTakers();
List squades = extractSquades(teachclass);
Set lonelyTakers = new HashSet();
for (CourseTaker taker : takers) {
boolean lonely = true;
for (Squad squad : squades) {
if (squad.equals(taker.getStd().getSquad())) {
lonely = false;
break;
}
}
if (lonely) {
lonelyTakers.add(taker);
}
}
return lonelyTakers;
}
public Set extractPossibleCourseTakers(Clazz clazz) {
Enrollment teachclass = clazz.getEnrollment();
// 这个会按照学号排序的
Set possibleTakers = new TreeSet(new Comparator() {
public int compare(CourseTaker o1, CourseTaker o2) {
return o1.getStd().getCode().compareTo(o2.getStd().getCode());
}
});
if (CollectUtils.isNotEmpty(teachclass.getCourseTakers())) {
possibleTakers.addAll(teachclass.getCourseTakers());
return possibleTakers;
}
List squades = extractSquades(teachclass);
ElectionMode eleMode = new ElectionMode();
eleMode.setId(ElectionMode.Assigned);
for (Squad squad : squades) {
for (Student std : squad.getStudents(clazz.getSemester().getBeginOn())) {
CourseTaker taker = new CourseTaker(clazz, std, new CourseTakeType(CourseTakeType.Normal));
taker.setCourseType(clazz.getCourseType());
taker.setElectionMode(eleMode);
possibleTakers.add(taker);
}
}
return possibleTakers;
}
public void limitEnrollment(boolean inclusive, Enrollment teachclass, String... grades) {
if (grades.length > 0) {
Enrollment clb = (Enrollment) teachclass;
ClazzRestriction group = clb.getOrCreateDefautRestriction();
RestrictionBuilder builder = builder(group);
builder.clear(ClazzRestrictionMeta.Grade);
if (inclusive) {
builder.inGrades(grades);
} else {
builder.notInGrades(grades);
}
}
}
public > void limitEnrollment(boolean inclusive, Enrollment teachclass, T... entities) {
if (entities.length > 0) {
Enrollment clb = (Enrollment) teachclass;
ClazzRestriction group = clb.getOrCreateDefautRestriction();
RestrictionBuilder builder = builder(group);
T first = entities[0];
if (first instanceof Squad) {
builder.clear(ClazzRestrictionMeta.Squad);
} else if (first instanceof StdType) {
builder.clear(ClazzRestrictionMeta.StdType);
} else if (first instanceof Major) {
builder.clear(ClazzRestrictionMeta.Major);
} else if (first instanceof Direction) {
builder.clear(ClazzRestrictionMeta.Direction);
} else if (first instanceof Department) {
builder.clear(ClazzRestrictionMeta.Department);
} else if (first instanceof EducationLevel) {
builder.clear(ClazzRestrictionMeta.Level);
} else if (first instanceof Gender) {
builder.clear(ClazzRestrictionMeta.Gender);
} else if (first instanceof EducationType) {
builder.clear(ClazzRestrictionMeta.EduType);
} else {
throw new RuntimeException("not supported limit meta class " + first.getClass().getName());
}
if (inclusive) {
builder.in(entities);
} else {
builder.notIn(entities);
}
}
}
private Pair> xtractLimitDirtyWork(ClazzRestriction group, ClazzRestrictionMeta limitMeta) {
if (group == null) {
return new Pair>(null, CollectUtils.newArrayList());
}
for (ClazzRestrictionItem item : group.getItems()) {
if (ClazzRestrictionMeta.Level.equals(limitMeta)) {
if (ClazzRestrictionMeta.Level.equals(item.getMeta())) {
return new Pair>(item.isIncluded(),
entityDao.get(EducationLevel.class, Strings.splitToInt(item.getContents())));
}
} else if (ClazzRestrictionMeta.Squad.equals(limitMeta)) {
if (ClazzRestrictionMeta.Squad
.equals(item.getMeta())) {
return new Pair>(item.isIncluded(),
entityDao.get(Squad.class, Strings.splitToLong(item.getContents())));
}
} else if (ClazzRestrictionMeta.Department.equals(limitMeta)) {
if (ClazzRestrictionMeta.Department
.equals(item.getMeta())) {
return new Pair>(item.isIncluded(),
entityDao.get(Department.class, Strings.splitToInt(item.getContents())));
}
} else if (ClazzRestrictionMeta.Major.equals(limitMeta)) {
if (ClazzRestrictionMeta.Major
.equals(item.getMeta())) {
return new Pair>(item.isIncluded(),
entityDao.get(Major.class, Strings.splitToLong(item.getContents())));
}
} else if (ClazzRestrictionMeta.Direction.equals(limitMeta)) {
if (ClazzRestrictionMeta.Direction
.equals(item.getMeta())) {
return new Pair>(item.isIncluded(),
entityDao.get(Direction.class, Strings.splitToLong(item.getContents())));
}
} else if (ClazzRestrictionMeta.StdType.equals(limitMeta)) {
if (ClazzRestrictionMeta.StdType
.equals(item.getMeta())) {
return new Pair>(item.isIncluded(),
entityDao.get(StdType.class, Strings.splitToInt(item.getContents())));
}
} else if (ClazzRestrictionMeta.Grade.equals(limitMeta)) {
if (ClazzRestrictionMeta.Grade
.equals(item.getMeta())) {
return new Pair>(item.isIncluded(),
Arrays.asList(Strings.split(item.getContents())));
}
} else if (ClazzRestrictionMeta.Gender.equals(limitMeta)) {
if (ClazzRestrictionMeta.Gender
.equals(item.getMeta())) {
return new Pair>(item.isIncluded(),
entityDao.get(Gender.class, Strings.splitToInt(item.getContents())));
}
} else if (ClazzRestrictionMeta.EduType.equals(limitMeta)) {
if (ClazzRestrictionMeta.EduType
.equals(item.getMeta())) {
return new Pair>(item.isIncluded(),
entityDao.get(EducationType.class, Strings.splitToInt(item.getContents())));
}
}
}
return new Pair>(Boolean.TRUE, CollectUtils.newArrayList());
}
private Map>> xtractLimitDirtyWork(Enrollment teachclass,
ClazzRestrictionMeta limitMeta) {
List groups = teachclass.getRestrictions();
Map>> groupDatas = CollectUtils.newHashMap();
for (ClazzRestriction group : groups) {
if (!group.isPrime()) {
continue;
}
groupDatas.put(group, xtractLimitDirtyWork(group, limitMeta));
}
return groupDatas;
}
public Map>> xtractEducationLimit(Enrollment teachclass) {
Map>> tmpRes = xtractLimitDirtyWork(teachclass,
ClazzRestrictionMeta.Level);
Map>> results = CollectUtils.newHashMap();
for (Map.Entry>> tmpEntrySet : tmpRes.entrySet()) {
Pair> tmpPair = tmpEntrySet.getValue();
results.put(tmpEntrySet.getKey(),
new Pair>(tmpPair._1, (List) tmpPair._2));
}
return results;
}
public Pair> xtractEducationLimit(ClazzRestriction group) {
Pair> tmpRes = xtractLimitDirtyWork(group, ClazzRestrictionMeta.Level);
return new Pair>(tmpRes._1, (List) tmpRes._2);
}
public Map>> xtractSquadLimit(Enrollment teachclass) {
Map>> tmpRes = xtractLimitDirtyWork(teachclass,
ClazzRestrictionMeta.Squad);
Map>> results = CollectUtils.newHashMap();
for (Map.Entry>> tmpEntrySet : tmpRes.entrySet()) {
Pair> tmpPair = tmpEntrySet.getValue();
results.put(tmpEntrySet.getKey(), new Pair<>(tmpPair._1, (List) tmpPair._2));
}
return results;
}
public Pair> xtractSquadLimit(ClazzRestriction group) {
Pair> tmpRes = xtractLimitDirtyWork(group, ClazzRestrictionMeta.Squad);
return new Pair>(tmpRes._1, (List) tmpRes._2);
}
public Map>> xtractAttendDepartLimit(Enrollment teachclass) {
Map>> tmpRes = xtractLimitDirtyWork(teachclass,
ClazzRestrictionMeta.Department);
Map>> results = CollectUtils.newHashMap();
for (Map.Entry>> tmpEntrySet : tmpRes.entrySet()) {
Pair> tmpPair = tmpEntrySet.getValue();
results.put(tmpEntrySet.getKey(),
new Pair>(tmpPair._1, (List) tmpPair._2));
}
return results;
}
public Pair> xtractAttendDepartLimit(ClazzRestriction group) {
Pair> tmpRes = xtractLimitDirtyWork(group, ClazzRestrictionMeta.Department);
return new Pair>(tmpRes._1, (List) tmpRes._2);
}
public Map>> xtractDirectionLimit(Enrollment teachclass) {
Map>> tmpRes = xtractLimitDirtyWork(teachclass,
ClazzRestrictionMeta.Direction);
Map>> results = CollectUtils.newHashMap();
for (Map.Entry>> tmpEntrySet : tmpRes.entrySet()) {
Pair> tmpPair = tmpEntrySet.getValue();
results.put(tmpEntrySet.getKey(),
new Pair>(tmpPair._1, (List) tmpPair._2));
}
return results;
}
public Pair> xtractDirectionLimit(ClazzRestriction group) {
Pair> tmpRes = xtractLimitDirtyWork(group, ClazzRestrictionMeta.Direction);
return new Pair>(tmpRes._1, (List) tmpRes._2);
}
public Map>> xtractGradeLimit(Enrollment teachclass) {
List groups = teachclass.getRestrictions();
Map>> groupDatas = CollectUtils.newHashMap();
for (ClazzRestriction group : groups) {
if (!group.isPrime()) {
continue;
}
groupDatas.put(group, xtractGradeLimit(group));
}
return groupDatas;
}
public Pair> xtractGradeLimit(ClazzRestriction group) {
for (ClazzRestrictionItem item : group.getItems()) {
if (ClazzRestrictionMeta.Grade.equals(item.getMeta())) {
return new Pair>(item.isIncluded(),
CollectUtils.newArrayList(item.getContents()));
}
}
return new Pair>(null, new ArrayList());
}
public Map>> xtractMajorLimit(Enrollment teachclass) {
Map>> tmpRes = xtractLimitDirtyWork(teachclass,
ClazzRestrictionMeta.Major);
Map>> results = CollectUtils.newHashMap();
for (Map.Entry>> tmpEntrySet : tmpRes.entrySet()) {
Pair> tmpPair = tmpEntrySet.getValue();
results.put(tmpEntrySet.getKey(), new Pair>(tmpPair._1, (List) tmpPair._2));
}
return results;
}
public Pair> xtractMajorLimit(ClazzRestriction group) {
Pair> tmpRes = xtractLimitDirtyWork(group, ClazzRestrictionMeta.Major);
return new Pair>(tmpRes._1, (List) tmpRes._2);
}
public Map>> xtractStdTypeLimit(Enrollment teachclass) {
Map>> tmpRes = xtractLimitDirtyWork(teachclass,
ClazzRestrictionMeta.StdType);
Map>> results = CollectUtils.newHashMap();
for (Map.Entry>> tmpEntrySet : tmpRes.entrySet()) {
Pair> tmpPair = tmpEntrySet.getValue();
results.put(tmpEntrySet.getKey(),
new Pair>(tmpPair._1, (List) tmpPair._2));
}
return results;
}
public Pair> xtractStdTypeLimit(ClazzRestriction group) {
Pair> tmpRes = xtractLimitDirtyWork(group, ClazzRestrictionMeta.StdType);
return new Pair>(tmpRes._1, (List) tmpRes._2);
}
public boolean isAutoName(Clazz clazz) {
// 检查是否需要自动命名
boolean isAutoName = true;
if (clazz.getId() != null) {
String teachclassName = clazz.getClazzName();
String autoName = teachclassNameStrategy.genName(clazz);
if (teachclassName != null && !teachclassName.equals(autoName)) {
isAutoName = false;
}
}
return isAutoName;
}
public RestrictionPair xtractLimitGroup(ClazzRestriction group) {
RestrictionPair pair = new RestrictionPair(group);
Pair> gradeLimit = xtractLimitDirtyWork(group, ClazzRestrictionMeta.Grade);
if (gradeLimit._1 != null) pair.setGradeLimit(gradeLimit);
Pair> stdTypeLimit = xtractLimitDirtyWork(group, ClazzRestrictionMeta.StdType);
if (stdTypeLimit._1 != null) pair.setStdTypeLimit(stdTypeLimit);
Pair> genderLimit = xtractLimitDirtyWork(group, ClazzRestrictionMeta.Gender);
if (genderLimit._1 != null) pair.setGenderLimit(genderLimit);
Pair> departLimit = xtractLimitDirtyWork(group, ClazzRestrictionMeta.Department);
if (departLimit._1 != null) pair.setDepartmentLimit(departLimit);
Pair> majorLimit = xtractLimitDirtyWork(group, ClazzRestrictionMeta.Major);
if (majorLimit._1 != null) pair.setMajorLimit(majorLimit);
Pair> directionLimit = xtractLimitDirtyWork(group, ClazzRestrictionMeta.Direction);
if (directionLimit._1 != null) pair.setDirectionLimit(directionLimit);
Pair> squadLimit = xtractLimitDirtyWork(group, ClazzRestrictionMeta.Squad);
if (squadLimit._1 != null) pair.setSquadLimit(squadLimit);
Pair> levelLimit = xtractLimitDirtyWork(group, ClazzRestrictionMeta.Level);
if (levelLimit._1 != null) pair.setLevelLimit(levelLimit);
Pair> eduTypeLimit = xtractLimitDirtyWork(group, ClazzRestrictionMeta.EduType);
if (levelLimit._1 != null) pair.setEduTypeLimit(eduTypeLimit);
return pair;
}
public void setEnrollmentNameStrategy(ClazzNameStrategy teachclassNameStrategy) {
this.teachclassNameStrategy = teachclassNameStrategy;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy