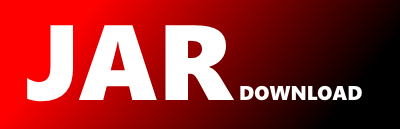
src.openwfe.org.MapUtils Maven / Gradle / Ivy
/*
* Copyright (c) 2001-2006, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: MapUtils.java 2673 2006-05-26 21:08:46Z jmettraux $
*/
//
// MapUtils.java
//
// [email protected]
//
// generated with
// jtmpl 1.1.00 16.08.2003 John Mettraux ([email protected])
//
package openwfe.org;
import openwfe.org.time.Time;
/**
* Methods for extracting values out of maps
*
* CVS Info :
*
$Author: jmettraux $
*
$Id: MapUtils.java 2673 2006-05-26 21:08:46Z jmettraux $
*
* @author [email protected]
*/
public abstract class MapUtils
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(MapUtils.class.getName());
//
// CONSTRUCTORS
/*
* Preventing further extensions of that class.
*/
private MapUtils ()
{
super();
}
//
// METHODS
/**
* Returns the String value behind the given key.
* If the key is not present, null will be returned.
*/
public static String getAsString
(final java.util.Map m,
final String key)
{
Object value = m.get(key);
if (value == null) return null;
return value.toString();
}
/**
* Returns the String value behind the given key.
* If the key is not present, the given default value will be
* returned.
*/
public static String getAsString
(final java.util.Map m,
final String key,
final String defaultValue)
{
String value = getAsString(m, key);
if (value == null) return defaultValue;
return value;
}
/**
* If the key is not present in the map, this method will
* throw an IllegalArgumentException.
*/
public static String getMandatoryString
(final java.util.Map m,
final String key)
{
final String result = getAsString(m, key);
if (result == null)
{
throw new IllegalArgumentException
("mandatory parameter '"+key+"' is missing.");
}
return result;
}
/**
* Tries to map to an integer the value of parameter given by its key.
* Will throw an IllegalArgumentException if it can't.
*/
public static int getMandatoryInt
(final java.util.Map m, final String key)
{
final String sValue = getAsString(m, key);
if (sValue == null)
{
throw new IllegalArgumentException
("mandatory int parameter '"+key+"' is missing.");
}
try
{
return Integer.parseInt(sValue);
}
catch (final NumberFormatException e)
{
throw new IllegalArgumentException
("mandatory int parameter '"+key+
"' has value '"+sValue+
"' which cannot be mapped to an integer");
}
}
/**
* Returns a Long and a String : the count in milliseconds described as a
* 'time string' in the String, a 'time string' is something like
* "3h4m30s".
*/
public static Object[] getMandatoryTimeAndString
(final java.util.Map m, final String key)
{
final String sValue = getMandatoryString(m, key);
try
{
final long tValue = Time.parseTimeString(sValue);
return new Object[] { new Long(tValue), sValue };
}
catch (final NumberFormatException e)
{
throw new IllegalArgumentException
("Failed to turn '"+sValue+"' into a count of milliseconds.");
}
}
/**
* Returns a duration in milliseconds, the expected param value is
* something like "3d4h10m".
*
* @see openwfe.org.time.Time
*/
public static long getAsTime
(final java.util.Map m,
final String key)
throws
NumberFormatException
{
String sValue = getAsString(m, key);
if (sValue == null)
{
throw new NumberFormatException
("No assigned value for '"+key+"'");
}
return Time.parseTimeString(sValue);
}
/**
* Returns a duration in milliseconds, the expected param value is
* something like "3d4h10m".
* A default long duration is given.
*
* @see openwfe.org.time.Time
*/
public static long getAsTime
(final java.util.Map m, final String key, long defaultValue)
throws
NumberFormatException
{
String sValue = getAsString(m, key);
if (sValue == null) return defaultValue;
return Time.parseTimeString(sValue);
}
/**
* Returns a duration in milliseconds, the expected param value is
* something like "3d4h10m".
* A default duration is given, and it's also expressed as a 'time string'.
*
* @see openwfe.org.time.Time
*/
public static long getAsTime
(final java.util.Map m, final String key, final String defaultValue)
throws
NumberFormatException
{
String sValue = getAsString(m, key);
if (sValue == null) return Time.parseTimeString(defaultValue);
return Time.parseTimeString(sValue);
}
/**
* Excepts a parameter whose value may be returned as a long value.
*/
public static long getAsLong
(final java.util.Map m, final String key)
throws
NumberFormatException
{
String sValue = getAsString(m, key);
if (sValue == null)
{
throw new NumberFormatException
("No assigned value for '"+key+"'");
}
return Long.parseLong(sValue);
}
/**
* Excepts a parameter whose value may be returned as a long value.
*/
public static long getAsLong
(final java.util.Map m, final String key, final long defaultValue)
{
String sValue = getAsString(m, key);
if (sValue == null) return defaultValue;
try
{
return Long.parseLong(sValue);
}
catch (NumberFormatException nfe)
{
log.info
("'"+sValue+"' isn't a long value. Returning default "+
defaultValue);
return defaultValue;
}
}
/**
* Excepts a parameter whose value may be returned as an integer value.
*/
public static int getAsInt
(final java.util.Map m, final String key, final int defaultValue)
{
String sValue = getAsString(m, key);
if (sValue == null) return defaultValue;
try
{
return Integer.parseInt(sValue);
}
catch (NumberFormatException nfe)
{
log.info
("'"+sValue+"' isn't an int value. Returning default "+
defaultValue);
return defaultValue;
}
}
/**
* Excepts a parameter whose value may be returned as boolean value.
*/
public static boolean getAsBoolean
(final java.util.Map m, final String key, final boolean defaultValue)
{
final Object o = m.get(key);
if (o == null) return defaultValue;
if (o instanceof Boolean) return ((Boolean)o).booleanValue();
String sValue = o.toString();
return sValue.equalsIgnoreCase("true");
}
/**
* Excepts a parameter whose value may be returned as double [precision]
* value.
*/
public static double getAsDouble
(final java.util.Map m, final String key)
throws
NumberFormatException
{
String sValue = getAsString(m, key);
if (sValue == null)
{
throw new NumberFormatException
("No assigned value for '"+key+"'");
}
return Double.parseDouble(sValue);
}
/**
* Returns a float value for the given parameter name.
*/
public static float getAsFloat
(final java.util.Map m,
final String paramName,
final float defaultValue)
{
try
{
return Float.parseFloat(getAsString(m, paramName));
}
catch (final Throwable t)
{
}
return defaultValue;
}
/**
* The parameter value is supposed to be comma separated list of
* values, this method will return this list of trimmed strings or
* null if the parameter is not present.
* If the parameter is present but has an empty value, an empty
* list will be returned.
*/
public static java.util.List getAsList
(final java.util.Map m,
final String paramName)
{
String s = (String)m.get(paramName);
if (s == null) return null;
s = s.trim();
final String[] ss = s.split(",\\s*");
final java.util.ArrayList l = new java.util.ArrayList(ss.length);
for (int i=0; i