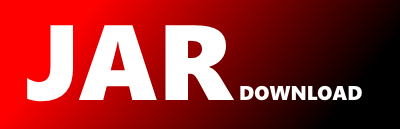
src.openwfe.org.ReflectionUtils Maven / Gradle / Ivy
/*
* Copyright (c) 2001-2006, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: ReflectionUtils.java 2673 2006-05-26 21:08:46Z jmettraux $
*/
//
// ReflectionUtils.java
//
// [email protected]
//
// generated with
// jtmpl 1.1.01 2004/05/19 ([email protected])
//
package openwfe.org;
import java.lang.reflect.Array;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
/**
* Methods for instatiating classes on the fly...
*
* CVS Info :
*
$Author: jmettraux $
*
$Id: ReflectionUtils.java 2673 2006-05-26 21:08:46Z jmettraux $
*
* @author [email protected]
*/
public abstract class ReflectionUtils
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(ReflectionUtils.class.getName());
/**
* contains simply 'class', this is supposed to be the name of the parameter
* holding the classname for the initObject(java.util.Map params) method.
*/
public final static String P_CLASS
= "class";
private final static String IS = "is";
private final static String GET = "get";
private final static String SET = "set";
//
// caching the pair of get/set methods for each bean class
private static java.util.Map fieldCache = new java.util.HashMap();
//
// METHODS
/**
* finds the init(java.util.Map initParameters) method in an object
* and invokes it
*/
public static Object initObject
(Object o, java.util.Map initParameters)
throws
NoSuchMethodException,
IllegalAccessException,
java.lang.reflect.InvocationTargetException
{
//
// not a permission
Class clazz = o.getClass();
java.lang.reflect.Method initMethod =
clazz.getMethod("init", new Class[] { java.util.Map.class });
//log.debug("------ found the init() method");
initMethod.invoke(o, new Object[] { initParameters });
//log.debug("------ init() method invocation seems successful");
return o;
}
/**
* This method is intended for classes with constructor that takes no
* parameter and a init(java.util.Map initParameters) method.
* By using this method, you can build an instance of such a class very
* easily.
*/
public static Object initObjectOfClass
(final String className, final java.util.Map initParameters)
throws
Exception
{
Class clazz = Class.forName(className);
Object instance = clazz.newInstance();
return initObject(instance, initParameters);
}
/**
* init an object whose class is contained in a P_CLASS named parameter
* (usually 'class')
*/
public static Object initObject
(final java.util.Map initParameters)
throws
Exception
{
final String className = (String)initParameters.get(P_CLASS);
if (className == null)
{
throw new IllegalArgumentException
("There is no parameter '"+P_CLASS+
"' ("+ReflectionUtils.class.getName()+
".P_CLASS). Cannot init instance.");
}
return initObjectOfClass(className, initParameters);
}
/**
* A reflective method.
*
* Customer c = new Customer("john");
* Object o = Utils.getAttribute(c, "name");
* ...
* o will yield the value "john" (because "john".equals(c.getName()))
* ...
*/
public static Object getAttribute
(Object o, String attributeName)
throws
NoSuchMethodException,
IllegalAccessException,
java.lang.reflect.InvocationTargetException
{
Class clazz = o.getClass();
String methodName =
"get" +
attributeName.substring(0, 1).toUpperCase() +
attributeName.substring(1);
java.lang.reflect.Method getMethod =
clazz.getMethod(methodName, new Class[] {});
return getMethod.invoke(o, new Object[] {});
}
/**
* Given an instance, the name of one of its attribute (property) sets a
* new value for it.
*/
public static Object setAttribute
(Object o, String attributeName, Object value)
throws
Exception
{
Class clazz = o.getClass();
String methodName =
"set" +
attributeName.substring(0, 1).toUpperCase() +
attributeName.substring(1);
java.lang.reflect.Method method = null;
Class parameterClass = null;
java.lang.reflect.Method[] methods = clazz.getMethods();
for (int i=0; i 2)
{
final String itemClassName =
arrayClassName.substring(2, arrayClassName.length()-1);
return Class.forName(itemClassName);
}
final char c = arrayClassName.charAt(1);
if (c == 'I') return Integer.class;
if (c == 'J') return Long.class;
if (c == 'F') return Float.class;
if (c == 'D') return Double.class;
if (c == 'B') return Byte.class;
if (c == 'C') return Character.class;
if (c == 'S') return Short.class;
if (c == 'Z') return Boolean.class;
return null;
}
/**
* Returns true if the given class represents an array of primitives
* like int[] or boolean[].
*/
public static boolean isPrimitiveArray (final Class clazz)
{
if ( ! clazz.isArray()) return false;
final String s = clazz.getName();
if (s.length() != 2) return false;
if (s.charAt(0) != '[') return false;
final char c = s.charAt(1);
return
(c == 'I' ||
c == 'J' ||
c == 'F' ||
c == 'D' ||
c == 'B' ||
c == 'C' ||
c == 'S' ||
c == 'Z');
}
/**
* Returns a list of attribute (field) names for a given object.
*/
public static java.util.List listAttributes (final Object o)
{
final Class clazz = o.getClass();
final java.lang.reflect.Method[] methods = clazz.getMethods();
final java.util.List attributeNames =
new java.util.ArrayList(methods.length);
for (int i=0; i"+getMethodName+"<");
if (returnType == null) continue;
if (getMethod.getParameterTypes().length > 1) continue;
//log.debug("listReadWriteFields() return and params ok");
if (( ! getMethodName.startsWith(GET)) &&
( ! getMethodName.startsWith(IS)))
{
continue;
}
//log.debug("listReadWriteFields() prefix ok");
String rawFieldName = getMethodName.substring(3);
if (getMethodName.startsWith(IS))
rawFieldName = getMethodName.substring(2);
Method setMethod = null;
try
{
setMethod = clazz.getMethod
(SET + rawFieldName, new Class[] { returnType });
final String sReturnType = ""+setMethod.getReturnType();
if (( ! sReturnType.equals("void")) &&
( ! sReturnType.equals("null")))
{
continue;
}
}
catch (final NoSuchMethodException nsme)
{
continue;
}
//log.debug("listReadWriteFields() adding >"+rawFieldName+"<");
result.add(new Method[] { getMethod, setMethod });
}
//
// cache result
fieldCache.put(instance.getClass(), result);
//
// that's all folks
return result;
}
/**
* Given a path like "openwfe.org.engine.Definitions.OPENWFE_VERSION"
* returns the value of the constant (as a String).
*/
public static String lookupConstantValue (final String constantPath)
{
try
{
final int i = constantPath.lastIndexOf(".");
final String className = constantPath.substring(0, i);
final String constantName = constantPath.substring(i+1);
//log.debug("extractConstant() className >"+className+"<");
//log.debug("extractConstant() constantName >"+constantName+"<");
final Class clazz = Class.forName(className);
final Field fConstant = clazz.getField(constantName);
return ""+fConstant.get(null);
}
catch (final Throwable t)
{
log.warn("extractConstant() failed to determine value", t);
return null;
}
}
}