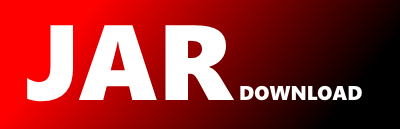
src.openwfe.org.app.XmlApplicationContextBuilder Maven / Gradle / Ivy
/*
* Copyright (c) 2001-2006, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: XmlApplicationContextBuilder.java 2925 2006-07-08 09:28:31Z jmettraux $
*/
//
// XmlApplicationContextBuilder.java
//
// [email protected]
//
// generated with
// jtmpl 1.1.01 2004/05/19 ([email protected])
//
package openwfe.org.app;
import openwfe.org.Utils;
import openwfe.org.Service;
import openwfe.org.Parameters;
import openwfe.org.ReflectionUtils;
import openwfe.org.ApplicationContext;
/**
* The classical decoder for OpenWFE application configuration files
* (like etc/engine/engine-configuration.xml).
*
* CVS Info :
*
$Author: jmettraux $
*
$Id: XmlApplicationContextBuilder.java 2925 2006-07-08 09:28:31Z jmettraux $
*
* @author [email protected]
*/
public class XmlApplicationContextBuilder
implements ApplicationContextBuilder
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(XmlApplicationContextBuilder.class.getName());
//
// CONSTANTS & co
//
// FIELDS
//
// CONSTRUCTORS
/**
* The Application will use this no-params constructor to get a fresh
* builder
*/
public XmlApplicationContextBuilder ()
{
super();
}
//
// METHODS from ApplicationContextBuilder
public ApplicationContext build
(final Object info)
throws
ApplicationBuildingException
{
return build(info, null);
}
public ApplicationContext build
(final Object info, final String applicationDirectory)
throws
ApplicationBuildingException
{
if (info == null)
{
throw new ApplicationBuildingException
("Cannot build ApplicationContext out of 'null' info.");
}
ApplicationContext result = null;
if (info instanceof String)
{
final String s = (String)info;
/*
java.net.URL url = null;
try
{
url = new java.net.URL(Utils.expandUrl(s));
}
catch (final java.net.MalformedURLException e)
{
// ignore
}
if (url != null) return build(url, applicationDirectory);
return doBuild(new java.io.File(s), applicationDirectory);
*/
try
{
return build(Utils.toUrl(s), applicationDirectory);
}
catch (final java.net.MalformedURLException e)
{
log.error
("build() "+
"cannot read ApplicationContext out of '"+s+"'",
e);
throw new ApplicationBuildingException
("cannot read ApplicationContext out of '"+s+"'",
e);
}
}
else if (info instanceof java.net.URL)
{
return doBuild
((java.net.URL)info, applicationDirectory);
}
if (result == null)
{
log.error
("build() "+
"Cannot build ApplicationContext out of instance of class "+
info.getClass());
throw new ApplicationBuildingException
("Cannot build ApplicationContext out of instance of class "+
info.getClass());
}
return result;
}
//
// METHODS
private ApplicationContext doBuild
(final java.net.URL url, final String applicationDirectory)
throws
ApplicationBuildingException
{
log.info("doBuild(u) building from '"+url+"'");
org.jdom.Document xmlConfiguration = null;
try
{
org.jdom.input.SAXBuilder builder = new org.jdom.input.SAXBuilder();
xmlConfiguration = builder.build(url);
}
catch (final Throwable t)
{
throw new ApplicationBuildingException
("Failed to interpret configuration file '"+url+"'", t);
}
long lastModified = -1;
try
{
final java.net.URLConnection con = url.openConnection();
lastModified = con.getLastModified();
}
catch (final Throwable t)
{
log.info
("doBuild() couldn't determine lastModified time "+
"for configuration file at "+url);
}
return doBuild
(lastModified,
xmlConfiguration.getRootElement(),
applicationDirectory);
}
private ApplicationContext doBuild
(final long lastModified,
final org.jdom.Element element,
final String applicationDirectory)
throws
ApplicationBuildingException
{
final ApplicationContext result = new ApplicationContext();
result.setLastModified(lastModified);
//
// if there is a name to the rootElement,
// then store the resultingApplication context is the singleton map...
String name = element.getAttributeValue("name");
if (name != null)
{
name = name.trim();
result.setApplicationName(name);
ApplicationContext.contextMap.put(name, result);
log.info("doBuild() put '"+name+"' in contextMap");
}
//
// include child configurations
java.util.Iterator it = element.getChildren("include").iterator();
while (it.hasNext())
{
final org.jdom.Element eInclude = (org.jdom.Element)it.next();
final String configFileName =
eInclude.getAttributeValue("configuration");
final ApplicationContext child = build(configFileName);
child.setParentContext(result);
result.put(child.getApplicationName(), child);
log.info
("Added sub-application under '"+
child.getApplicationName()+"'");
}
//
// extract global parameters
java.util.Map globalParams = Parameters.extractParameters(element);
result.putAll(globalParams);
if (applicationDirectory != null)
//
// setting the application directory now,
// in order to make sure to override the one found in the
// config file itself.
//
result.setApplicationDirectory(applicationDirectory);
//
// bind [system] properties
bindSystemProperties(element);
//
// extract and instantiate services
java.util.List services = element.getChildren("service");
it = services.iterator();
while (it.hasNext())
{
org.jdom.Element elt = (org.jdom.Element)it.next();
//
// gather info
String serviceName = elt.getAttributeValue("name");
String serviceClassName = elt.getAttributeValue("class");
java.util.Map serviceParams = Parameters.extractParameters(elt);
//
// instantiate service
Service service = null;
try
{
Class serviceClass = Class.forName(serviceClassName);
service = (Service)serviceClass.newInstance();
service.init(serviceName, result, serviceParams);
}
catch (Throwable t)
{
//throw new ServiceException
log.info
("Failed to instantiate service '"+serviceName+"'", t);
continue;
}
// and bind it into context
result.put(serviceName, service);
//
// service is ready.
log.info
("bound service '"+serviceName+"' ("+serviceClassName+")");
}
log.info
("Application '"+name+"' has been launched\n\n");
return result;
}
/**
* Parses 'property' children and binds results as system properties.
*/
protected void bindSystemProperties (final org.jdom.Element elt)
{
final java.util.Iterator it = elt.getChildren("property").iterator();
while (it.hasNext())
{
final org.jdom.Element e = (org.jdom.Element)it.next();
bindSystemProperty(e);
}
}
protected void bindSystemProperty (final org.jdom.Element elt)
{
//
// 1st alternative
//
//
//
// or
//
//
String name = elt.getAttributeValue("name");
String value = elt.getAttributeValue("value");
String constant = elt.getAttributeValue("constant");
if (name == null)
//
// 2nd alternative
//
//
// ...
// ...
//
{
name = elt.getChildTextTrim("name");
value = elt.getChildTextTrim("value");
constant = elt.getChildTextTrim("constant");
}
if (name == null)
//
// 3rd alternative
//
//
// ...
// ...
//
{
name = elt.getChildTextTrim("property-name");
value = elt.getChildTextTrim("property-value");
constant = elt.getChildTextTrim("property-constant");
}
if (name == null)
{
log.warn
("bindSystemProperty() "+
" without a 'name' attribute. Skipped.");
return;
}
if (constant != null)
value = ReflectionUtils.lookupConstantValue(constant);
name = name.trim();
value = value.trim();
System.getProperties().put(name, value);
log.info
("bindSystemProperty() "+
"'"+name+"' --> '"+value+"'");
}
//
// STATIC METHODS
}