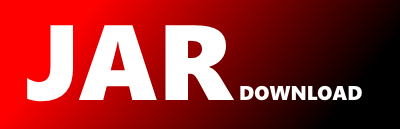
src.openwfe.org.mail.MailUtils Maven / Gradle / Ivy
/*
* Copyright (c) 2001-2006, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: MailUtils.java 2713 2006-06-01 14:38:45Z jmettraux $
*/
//
// MailUtils.java
//
// [email protected]
//
// generated with
// jtmpl 1.1.01 2004/05/19 ([email protected])
//
package openwfe.org.mail;
import javax.mail.Message;
import javax.mail.Message.RecipientType;
import javax.mail.Session;
import javax.mail.MessagingException;
import javax.mail.internet.MimeMessage;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.AddressException;
import openwfe.org.MapUtils;
/**
* Some static methods for Smtp / Pop / Imap...
*
* CVS Info :
*
$Author: jmettraux $
*
$Id: MailUtils.java 2713 2006-06-01 14:38:45Z jmettraux $
*
* @author [email protected]
*/
public abstract class MailUtils
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(MailUtils.class.getName());
//
// CONSTANTS & CO
/**
* Use this parameter ('smtp-host') to tell the participant which
* mail server it should use to send the notification email
*/
public final static String P_SMTP_SERVER
= "smtp-server";
/**
* Use this parameter ('smtp-port') to tell the participant which port
* of the mail server it should use to send the notification email
*/
public final static String P_SMTP_PORT
= "smtp-port";
/**
* Use this parameter ('mail-from') to indicate who should be the
* apparent author of the notification email
*/
public final static String P_MAIL_FROM
= "mail-from";
/* *
* Use this participant parameter named 'mail-encoding' to set the
* encoding to use for generating emails.
* By default, iso-8859-1 is used.
* /
public final static String P_MAIL_ENCODING
= "mail-encoding";
/*
* By default, emails are encoded using iso-8859-1
* /
private final static String DEFAULT_MAIL_ENCODING
= "iso-8859-1";
*/
//
// METHODS
/**
* Gets a mail session with the given [OpenWFE service] params.
*/
public static Session getMailSession (final java.util.Map params)
{
final java.util.Properties props = new java.util.Properties();
props.put
("mail.smtp.host",
MapUtils.getAsString(params, P_SMTP_SERVER, "127.0.0.1"));
props.put
("mail.smtp.port",
MapUtils.getAsString(params, P_SMTP_PORT, "25"));
if (log.isDebugEnabled())
{
log.debug
("getMailSession() smtp : "+
props.get("mail.smtp.host")+":"+props.get("mail.smtp.port"));
}
return Session.getDefaultInstance(props, null);
}
/* *
* create a MimeMessage instance with the given [OpenWFE service] params
* for configuring the mail session.
* /
public static MimeMessage createMessage (final java.util.Map params)
throws MessagingException
{
final MimeMessage m = new MimeMessage(getMailSession(params));
return m;
}
*/
/**
* Extracts from the params the 'mail-from' parameter.
* Returns null if this parameter is not present.
*/
public static InternetAddress getMailFrom (final java.util.Map params)
throws MessagingException
{
final String mailFrom = MapUtils.getAsString(params, P_MAIL_FROM);
if (mailFrom == null) return null;
return new InternetAddress(mailFrom);
}
/**
* Given a message and the params, will look in the params for 'mail-from'
* and if present, will set it as the 'from' of the message.
*/
public static void setMailFrom
(final Message m, final java.util.Map params)
throws
MessagingException
{
final InternetAddress a = getMailFrom(params);
if (a != null) m.setFrom(a);
}
/**
* Given a recipient list (email addresses separated by commas), adds them
* to the message.
*/
public static void addRecipients
(final Message m,
final RecipientType recipientType,
final String recipientList)
throws
MessagingException
{
if (recipientList == null) return;
final String[] ss = recipientList.split(", *");
for (int i=0; i