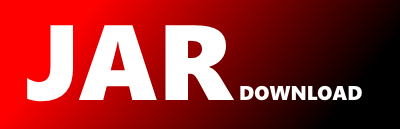
src.openwfe.org.sql.ds.DB2DataSource Maven / Gradle / Ivy
/*
* Copyright (c) 2001-2006, Jay Lawrence, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: DB2DataSource.java 2713 2006-06-01 14:38:45Z jmettraux $
*/
//
// DB2DataSource.java
//
// [email protected]
//
// based on MysqlDataSource.java - [email protected]
//
package openwfe.org.sql.ds;
import openwfe.org.MapUtils;
import openwfe.org.ServiceException;
import openwfe.org.ApplicationContext;
import com.ibm.db2.jcc.DB2SimpleDataSource;
import com.ibm.db2.jcc.DB2Diagnosable;
import com.ibm.db2.jcc.DB2Sqlca;
import java.sql.SQLException;
/**
* An OpenWFE data source for DB2
*
* CVS Info :
*
$Author: jmettraux $
*
$Date: 2006-06-01 16:38:45 +0200 (Thu, 01 Jun 2006) $
*
$Id: DB2DataSource.java 2713 2006-06-01 14:38:45Z jmettraux $
*
* @author [email protected]
* @author [email protected]
*/
public class DB2DataSource
extends OwfeDataSource
{
/*
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(DB2DataSource.class.getName());
*/
//
// CONSTANTS (definitions)
//public final static String P_URL = "url";
public final static String P_HOST = "db2Host";
public final static String P_PORT = "db2Port";
public final static int P_PORT_DEFAULT = 50000;
public final static String P_USER = "db2User";
public final static String P_PASS = "db2Pass";
public final static String P_DBNAME = "db2Database";
public final static String P_SCHEMA = "db2Schema";
public final static String P_TYPE = "db2Type";
public final static int P_TYPE_DEFAULT = 4;
private final static String CON_VALIDITY_SQL_CODE
= "select 1 from syscat.servers";
//
// FIELDS
//
// CONSTRUCTORS
public void init
(final String serviceName,
final ApplicationContext context,
final java.util.Map serviceParams)
throws
ServiceException
{
super.init(serviceName, context, serviceParams);
// final String url = MapUtils.getMandatoryString(this.serviceParams, P_URL);
final DB2SimpleDataSource ds = new DB2SimpleDataSource();
ds.setDatabaseName( MapUtils.getMandatoryString(serviceParams, P_DBNAME) );
ds.setCurrentSchema( MapUtils.getMandatoryString(serviceParams, P_SCHEMA) );
ds.setServerName( MapUtils.getMandatoryString(serviceParams, P_HOST) );
ds.setPortNumber( MapUtils.getAsInt(serviceParams, P_PORT, P_PORT_DEFAULT ) );
ds.setUser( MapUtils.getMandatoryString(serviceParams, P_USER) );
ds.setPassword( MapUtils.getMandatoryString(serviceParams, P_PASS) );
ds.setDriverType( MapUtils.getAsInt(serviceParams, P_TYPE, P_TYPE_DEFAULT ) );
this.setDataSource(ds);
}
//
// METHODS from Service
//
// METHODS
/*
* The new version of the driver (3.0.11) doesn't allow for concurrent
* connections, this releaseConnection() implementation kills the
* connection.
*
public void releaseConnection ()
{
try
{
stop();
}
catch (Exception e)
{
}
}
*
*/
public void logSQLException( String funcName, org.apache.log4j.Logger logger, SQLException e ) {
while ( e != null ) {
logger.error( funcName + "() SQLException : " + e.toString() );
if ( e instanceof DB2Diagnosable ) {
DB2Sqlca sqlca = ((DB2Diagnosable)e).getSqlca();
if ( sqlca != null ) {
int sqlCode = sqlca.getSqlCode(); // Get the SQL error code
String sqlErrmc = sqlca.getSqlErrmc();
// Get the entire SQLERRMC
String[] sqlErrmcTokens = sqlca.getSqlErrmcTokens();
// You can also retrieve the
// individual SQLERRMC tokens
String sqlErrp = sqlca.getSqlErrp();
// Get the SQLERRP
int[] sqlErrd = sqlca.getSqlErrd();
// Get SQLERRD fields
char[] sqlWarn = sqlca.getSqlWarn();
// Get SQLWARN fields
String sqlState = sqlca.getSqlState();
// Get SQLSTATE
String errMessage = "";
// ignore any errors in trying to get this "errMessage"
try { errMessage = sqlca.getMessage(); } catch ( Exception ignore ) { }
// Get error message
logger.error("Server error message: " + errMessage);
logger.error("--------------- SQLCA ---------------");
logger.error("Error code: " + sqlCode);
logger.error("SQLERRMC: " + sqlErrmc);
for (int i=0; i< sqlErrmcTokens.length; i++) {
logger.error(" token " + i + ": " + sqlErrmcTokens[i]);
}
logger.error( "SQLERRP: " + sqlErrp );
logger.error(
"SQLERRD(1): " + sqlErrd[0] + "\n" +
"SQLERRD(2): " + sqlErrd[1] + "\n" +
"SQLERRD(3): " + sqlErrd[2] + "\n" +
"SQLERRD(4): " + sqlErrd[3] + "\n" +
"SQLERRD(5): " + sqlErrd[4] + "\n" +
"SQLERRD(6): " + sqlErrd[5] );
logger.error(
"SQLWARN1: " + sqlWarn[0] + "\n" +
"SQLWARN2: " + sqlWarn[1] + "\n" +
"SQLWARN3: " + sqlWarn[2] + "\n" +
"SQLWARN4: " + sqlWarn[3] + "\n" +
"SQLWARN5: " + sqlWarn[4] + "\n" +
"SQLWARN6: " + sqlWarn[5] + "\n" +
"SQLWARN7: " + sqlWarn[6] + "\n" +
"SQLWARN8: " + sqlWarn[7] + "\n" +
"SQLWARN9: " + sqlWarn[8] + "\n" +
"SQLWARNA: " + sqlWarn[9] );
logger.error("SQLSTATE: " + sqlState);
// portion of SQLException
}
}
// Get next exception
e = (java.sql.SQLException)e.getNextException();
}
}
/*
* DB2 seems to return a null statement when the connection is
* invalid, might be a much quicker test than the SQL select test.
*
protected boolean isConnectionInvalid ()
{
try
{
final java.sql.Statement st = this.sqlConnection.createStatement();
if (st == null)
{
log.debug("isConnectionInvalid() Yes : statement is null");
return true;
}
SqlUtils.closeStatement(st);
}
catch (final Throwable t)
{
log.debug("isConnectionInvalid() Yes : ", t);
return true;
}
return false;
}
*
*/
//
// METHODS from OwfeDataSource
/**
* This method when implemented has to return a piece of SQL code
* that will be run against any database for connection
* validity checking.
*/
public String getConnectionValiditySqlCode ()
{
return CON_VALIDITY_SQL_CODE;
}
}