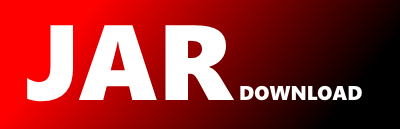
openwfe.org.rest.RestSession Maven / Gradle / Ivy
/*
* Copyright (c) 2001-2006, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: RestSession.java 2713 2006-06-01 14:38:45Z jmettraux $
*/
//
// RestSession.java
//
// [email protected]
//
// generated with
// jtmpl 1.0.04 31.10.2002 John Mettraux ([email protected])
//
package openwfe.org.rest;
import java.nio.channels.SelectionKey;
import java.nio.channels.SocketChannel;
import java.nio.channels.ReadableByteChannel;
import openwfe.org.Utils;
import openwfe.org.ExceptionUtils;
import openwfe.org.ServiceException;
import openwfe.org.OpenWfeException;
import openwfe.org.ApplicationContext;
import openwfe.org.net.NetUtils;
import openwfe.org.net.ChannelInputStream;
/**
* The skeleton of a REST session, implement it to handle your REST actions.
*
* CVS Info :
*
$Author: jmettraux $
*
$Date: 2006-06-01 16:38:45 +0200 (Thu, 01 Jun 2006) $
*
$Id: RestSession.java 2713 2006-06-01 14:38:45Z jmettraux $
*
* @author [email protected]
*/
public abstract class RestSession
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(RestSession.class.getName());
//
// CONSTANTS and DEFINITIONS
public final static String ACTION_END_WORK_SESSION
= "endworksession";
//
// FIELDS
private RestService service = null;
private ApplicationContext applicationContext = null;
private java.util.Map serviceParams = null;
private Long sessionId = null;
private long lastUsed = -1;
//
// CONSTRUCTORS
public void init
(final RestService service,
final Long sessionId,
final String username,
final String password)
throws
ServiceException
{
this.service = service;
this.sessionId = sessionId;
touch();
authentify(username, password);
}
//
// GETTERS and SETTERS
public RestService getService () { return this.service; }
//
// ABSTRACT METHODS
/**
* Implement this method so that it sets up the RMI or whatever
* back session.
*/
protected abstract boolean authentify
(String userName, String password)
throws
ServiceException;
/**
* Implement for determining what to do with every REST action
*/
protected boolean evalRequest
(final String action,
final SelectionKey key,
final String[] headers)
throws
Exception
{
final String methodName = "do_"+action.toLowerCase();
final java.lang.reflect.Method method = this.getClass()
.getMethod
(methodName,
new Class[]
{
SelectionKey.class,
String[].class
});
final Object result = method.invoke
(this, new Object[] { key, headers });
if (result == null) return true;
return ((Boolean)result).booleanValue();
}
//
// METHODS
public long getLastUsed ()
{
return this.lastUsed;
}
/**
* Handles the incoming REST request
*/
//public void handle (final SelectionKey key, final byte[] request)
public void handle
(final SelectionKey key,
final String[] headers)
{
touch();
final String firstLine = headers[0];
//
// which action is requested ?
String action = null;
Exception exception = null;
try
{
//firstLine = RestService.createBufferedReader(request).readLine();
if (log.isDebugEnabled())
log.debug("handle() firstLine is >"+firstLine+"<");
action = RestUtils.extractFromLine(firstLine, "action");
}
catch (java.io.IOException ie)
{
log.info("Failed to parse 'action' from http request", ie);
exception = ie;
}
if (action == null)
{
Object body = exception;
if (body == null) body = "No specific action requested";
NetUtils.httpReply
(key,
400,
"Bad Request",
this.service.getServerName(),
null,
"text/plain",
body);
return;
}
action = action.toLowerCase();
//
// point to action handling
if (action.equals(ACTION_END_WORK_SESSION))
{
if (this.service != null)
this.service.removeSession(this.sessionId);
log.info("Session "+this.sessionId+" ended.");
NetUtils.httpReply
(key,
200,
"OK",
this.service.getServerName(),
null,
"text/xml",
new org.jdom.Element("bye"));
return;
}
try
{
try
{
if (evalRequest(action, key, headers)) return;
}
catch (final java.lang.reflect.InvocationTargetException ite)
{
throw ite.getCause();
}
}
catch (final java.net.MalformedURLException mue)
{
log.info("Malformed URL Exception : ", mue);
NetUtils.httpReply
(key,
400,
"Bad Request",
this.service.getServerName(),
null,
"text/plain",
mue);
return;
}
catch (final HttpException he)
{
log.info("HTTP error : ", he);
NetUtils.httpReply
(key,
he.getErrorCode(),
he.getMessage(),
this.service.getServerName(),
null,
"text/plain",
he);
return;
}
catch (final Throwable t)
{
log.info("Internal Server error : ", t);
final Throwable rootCause = ExceptionUtils.getRootCause(t);
NetUtils.httpReply
(key,
500,
rootCause.toString()+" : "+rootCause.getMessage(),
this.service.getServerName(),
null,
"text/plain",
t);
return;
}
NetUtils.httpReply
(key,
400,
"Bad Request",
this.service.getServerName(),
null,
"text/plain",
"Action '"+action+"' has no implementation.");
}
/**
* This method simply updates the lastUsed field.
*/
public void touch ()
{
this.lastUsed = System.currentTimeMillis();
}
protected void reply
(final SelectionKey key, final org.jdom.Element elt)
{
NetUtils.httpReply
(key,
200,
"OK",
this.service.getServerName(),
null,
"text/xml",
elt);
//log.debug("reply() :\n"+elt);
}
//
// STATIC METHODS
public static String printSessions (final java.util.Map sessions)
{
StringBuffer sb = new StringBuffer();
sb.append("\n[\n ");
java.util.Iterator it = sessions.keySet().iterator();
while (it.hasNext())
{
Long sessionId = (Long)it.next();
RestSession session = (RestSession)sessions.get(sessionId);
sb.append(" ");
sb.append(sessionId);
sb.append(" : ");
sb.append(new java.util.Date(session.getLastUsed()));
if (it.hasNext())
{
sb.append(",\n");
}
}
sb.append("\n]\n");
return sb.toString();
}
public static org.jdom.Element parseBody
(final SelectionKey key, final String[] headers)
throws
Exception
{
log.debug("parseBody()");
final int bytesToRead = RestService.determineBytesToRead(headers);
//Thread.yield();
//
// build XML doc
final SocketChannel channel = (SocketChannel)key.channel();
final java.io.InputStream is = new java.io.BufferedInputStream
(new ChannelInputStream(channel, bytesToRead, 100));
final org.jdom.input.SAXBuilder builder =
new org.jdom.input.SAXBuilder();
final org.jdom.Document doc = builder.build(is);
//Utils.dump
// ("rest_session_", openwfe.org.xml.XmlUtils.toByteArray(doc));
return doc.getRootElement();
}
/**
* This method is used to turn a simple exception into an HttpError,
* very useful in final implementations of this class.
*/
public static void rethrowAsHttpException
(final int httpErrorCode, final Exception e)
throws
HttpException
{
throw new HttpException(httpErrorCode, e.getMessage());
}
}