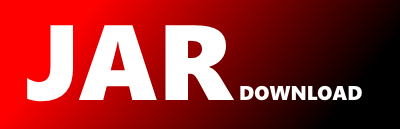
src.openwfe.org.engine.dispatch.AbstractWorkItemDispatcher Maven / Gradle / Ivy
/*
* Copyright (c) 2001-2006, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: AbstractWorkItemDispatcher.java 2575 2006-05-08 11:52:39Z jmettraux $
*/
//
// AbstractWorkItemDispatcher.java
//
// [email protected]
//
// generated with
// jtmpl 1.0.04 31.10.2002 John Mettraux ([email protected])
//
package openwfe.org.engine.dispatch;
import openwfe.org.MapUtils;
import openwfe.org.AbstractService;
import openwfe.org.ServiceException;
import openwfe.org.ApplicationContext;
import openwfe.org.engine.Definitions;
import openwfe.org.engine.listen.reply.OkReply;
import openwfe.org.engine.listen.reply.FatalReply;
import openwfe.org.engine.listen.reply.WarningReply;
import openwfe.org.engine.listen.reply.ListenerReply;
import openwfe.org.engine.workitem.WorkItemCoder;
import openwfe.org.engine.workitem.WorkItemCoderLoader;
/**
* Half an implementation of a WorkItemDispatcher
*
* CVS Info :
*
$Author: jmettraux $
*
$Date: 2006-05-08 13:52:39 +0200 (Mon, 08 May 2006) $
*
$Id: AbstractWorkItemDispatcher.java 2575 2006-05-08 11:52:39Z jmettraux $
*
* @author [email protected]
*/
public abstract class AbstractWorkItemDispatcher
extends AbstractService
implements WorkItemDispatcher
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(AbstractWorkItemDispatcher.class.getName());
//
// CONSTANTS (definitions)
/**
* This init param (named 'workItemCoder') is used to set the workitem
* coder used by this workitem dispatcher.
*/
public final static String WORKITEM_CODER
= "workItemCoder";
//
// FIELDS
private String workItemCoderName = null;
//
// CONSTRUCTORS
/**
* The required initialized method of a service.
*/
public void init
(final String serviceName,
final ApplicationContext context,
final java.util.Map serviceParams)
throws
ServiceException
{
super.init(serviceName, context, serviceParams);
//
// get workItemCoder
this.workItemCoderName = MapUtils.getAsString
(getParams(), WORKITEM_CODER);
// check it
final WorkItemCoderLoader loader =
Definitions.getWorkItemCoderLoader(getContext());
WorkItemCoder coder = loader.getCoder(this.workItemCoderName);
if (coder == null)
{
coder = loader.getDefaultCoder();
log.info("using default coder");
}
this.workItemCoderName = coder.getName();
//
// that's all folks
log.info
("Dispatcher '"+getName()+
"' using coder '"+this.workItemCoderName+"'");
}
//
// METHODS
/**
* Returns the name of workitem coder service that this dispatcher
* has to use. If null is returned, it means that this service will
* use the default workitem coder (as found in
* etc/engine/coder-configuratin.xml).
*/
public String getWorkItemCoderName ()
{
return this.workItemCoderName;
}
/**
* Internal usage : returns the workitem coder for usage.
*/
protected WorkItemCoder instantiateEncoder ()
throws DispatchingException
{
WorkItemCoderLoader coderLoader = Definitions
.getWorkItemCoderLoader(getContext());
WorkItemCoder coder = coderLoader.getDefaultCoder();
if (this.workItemCoderName != null)
coder = coderLoader.getCoder(this.workItemCoderName);
return coder;
}
/**
* This method is used by dispatcher implementations.
* It will throw a dispatching exception if and only if the
* reply is a fatal one.
*/
protected Object handleReply (ListenerReply reply)
throws DispatchingException
{
if (reply == null)
{
throw new DispatchingException("No reply from listener");
}
if (reply instanceof FatalReply)
{
throw new FatalDispatchingException((FatalReply)reply);
}
if (reply instanceof OkReply)
{
log.debug("Listener replied 'ok'");
return ((OkReply)reply).getConsumerResult();
}
WarningReply warn = (WarningReply)reply;
StringBuffer sb = new StringBuffer();
sb.append("listener warned :\n");
sb.append(warn.getMessage()); sb.append('\n');
sb.append(warn.getExceptionMessage()); sb.append('\n');
sb.append(warn.getExceptionStackTrace());
log.warn(sb.toString());
return null;
}
}