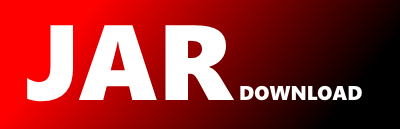
src.openwfe.org.engine.expressions.DoExpression Maven / Gradle / Ivy
/*
* Copyright (c) 2005, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: DoExpression.java 2447 2006-03-19 12:42:42Z jmettraux $
*/
//
// DoExpression.java
//
// [email protected]
//
// generated with
// jtmpl 1.1.01 2004/05/19 ([email protected])
//
package openwfe.org.engine.expressions;
import openwfe.org.engine.expool.PoolException;
import openwfe.org.engine.workitem.InFlowWorkItem;
import openwfe.org.engine.expressions.raw.RawExpression;
/**
* A 'do' may get 'undo'ne or 'redo'ne.
*
* CVS Info :
*
$Author: jmettraux $
*
$Id: DoExpression.java 2447 2006-03-19 12:42:42Z jmettraux $
*
* @author [email protected]
*/
public class DoExpression
extends OneRawChildExpression
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(DoExpression.class.getName());
//
// CONSTANTS & co
/**
* This constant is used by do/undo/redo to set/localize a 'do' in
* the variables of an expression tree.
*/
public final static String V_DO
= "__do__";
/**
* You can name a do with the 'name' attribute, then refer to it
* directly from your 'undo' or 'redo' with their 'ref' attribute.
*/
public final static String A_NAME
= "name";
/**
* Undo events will appear in the history with a '+UDO' code.
*/
public final static String EVT_UNDO = "+UDO";
/**
* Redo events will appear in the history with a '+RDO' code.
*/
public final static String EVT_REDO = "+RDO";
//
// FIELDS
//private RawExpression rawChild = null;
private InFlowWorkItem appliedItem = null;
private boolean inAction = false;
private String doName = null;
//
// CONSTRUCTORS
//
// BEAN METHODS
//public RawExpression getRawChild () { return this.rawChild; }
public InFlowWorkItem getAppliedItem () { return this.appliedItem; }
//public void setRawChild (final RawExpression re) { this.rawChild = re; }
public void setAppliedItem (final InFlowWorkItem wi) { this.appliedItem = wi; }
public boolean isInAction () { return this.inAction; }
public void setInAction (final boolean b) { this.inAction = b; }
public String getDoName () { return this.doName; }
public void setDoName (final String s) { this.doName = s; }
//
// METHODS
private String theDoName ()
{
if (this.doName != null) return this.doName;
return "";
}
/**
* As its name implies.
*/
public void undo ()
throws ApplyException
{
changeActionMode(true);
log.debug("undo() willing to cancel "+this.getChildExpressionId());
getExpressionPool().cancel(this.getChildExpressionId());
changeActionMode(false);
this.historyLog
(this.appliedItem,
EVT_UNDO,
null,
"undid '"+theDoName()+"'");
applyToParent(this.appliedItem);
}
/**
* Takes care of switching the action mode and then
* do a storeItself().
*/
protected void changeActionMode (final boolean b)
{
this.inAction = b;
this.storeItself();
}
/**
* As its name implies.
*/
public void redo ()
throws ApplyException
{
changeActionMode(true);
log.debug("redo() cancelling child "+this.getChildExpressionId());
getExpressionPool().cancel(this.getChildExpressionId());
log.debug("redo() cancel done.");
final RawExpression child = (RawExpression)getRawChild().clone();
try
{
getExpressionPool().add(child);
}
catch (final PoolException e)
{
throw new ApplyException
("Failed to add rawChild to expression pool", e);
}
log.debug("redo() applying "+child.getId());
this.historyLog
(this.appliedItem,
EVT_REDO,
null,
"redoing '"+theDoName()+"'");
getExpressionPool().apply(child.getId(), this.appliedItem);
changeActionMode(false);
}
//
// METHODS from FlowExpression
public void apply (final InFlowWorkItem wi)
throws ApplyException
{
if (this.getChildExpressionId() == null) return;
//
// prepare self
this.appliedItem = (InFlowWorkItem)wi.clone();
fetchRawChild();
//
// bind self as a variable
this.doName = lookupAttribute(A_NAME, wi);
String variableName = V_DO;
if (doName != null) variableName += doName;
this.bindVariable(variableName, this.getId());
this.storeItself();
//
// apply child
getExpressionPool().apply(this.getChildExpressionId(), wi);
}
public void reply (final InFlowWorkItem wi)
throws ReplyException
{
log.debug("reply()");
if (this.inAction) return;
super.reply(wi);
}
//
// STATIC METHODS
}