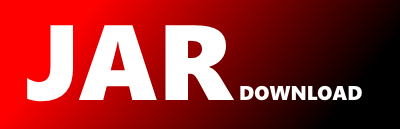
src.openwfe.org.engine.expressions.Iterator Maven / Gradle / Ivy
/*
* Copyright (c) 2005, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: Iterator.java 2444 2006-03-18 12:37:14Z jmettraux $
*/
//
// Iterator.java
//
// [email protected]
//
// generated with
// jtmpl 1.1.00 16.08.2003 John Mettraux ([email protected])
//
package openwfe.org.engine.expressions;
import openwfe.org.OpenWfeException;
import openwfe.org.engine.Definitions;
import openwfe.org.engine.launch.Launcher;
import openwfe.org.engine.workitem.InFlowWorkItem;
import openwfe.org.engine.workitem.MapAttribute;
import openwfe.org.engine.workitem.ListAttribute;
import openwfe.org.engine.workitem.StringAttribute;
import openwfe.org.engine.expressions.raw.RawExpression;
import openwfe.org.engine.expressions.sync.SynchableExpression;
/**
* An iterator will iterate for IteratorExpression and
* ConcurrentIteratorExpression, it does the job of keeping track
* of the loops for them.
*
* CVS Info :
*
$Author: jmettraux $
*
$Id: Iterator.java 2444 2006-03-18 12:37:14Z jmettraux $
*
* @author [email protected]
*/
public class Iterator
implements java.io.Serializable
{
static final long serialVersionUID = -2532616767097005828L;
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(Iterator.class.getName());
//
// CONSTANTS & co
/**
* You may determine on what to iterate with
*
* - on-value
* - on-field-value
* - on-variable-value
*
*
* That's why we have this prefix.
*/
public final static String VALUE_PREFIX
= "on-";
/**
* if no TO_FIELD and no TO_VARIABLE is provided, the current iteration
* value will be stored in the variable named here.
*/
public final static String DEFAULT_TO_VARIABLE
= "__iteration_value__";
/**
* Use this parameter to set the regex used to split the value string into
* the different values.
* By default it is set to ", *"
*/
public final static String VALUE_SEPARATOR
= "value-separator";
/**
* As already stated, this default separator is ", *"
*/
public final static String DEFAULT_VALUE_SEPARATOR
= ", *";
//
// FIELDS
private int iterationPosition = -1;
private java.util.List iterationList = null;
private FlowExpressionId templateId = null;
//
// CONSTRUCTORS
/**
* This constructor is necessary in order to xml-deserialize
* the Iterator out of the pool (it is embedded in an IteratorExpression
* though)
*/
public Iterator ()
{
super();
}
public Iterator
(final FlowExpression iteratingExpression,
final InFlowWorkItem wi)
throws
ApplyException
{
super();
prepareIterationList(iteratingExpression, wi);
}
//
// BEAN METHODS
public int getIterationPosition () { return this.iterationPosition; }
public java.util.List getIterationList () { return this.iterationList; }
public FlowExpressionId getTemplateId () { return this.templateId; }
public void setIterationPosition (int i) { this.iterationPosition = i; }
public void setIterationList (java.util.List l) { this.iterationList = l; }
public void setTemplateId (FlowExpressionId fei) { this.templateId = fei; }
//
// METHODS
/**
* Returns the current value for the iteration.
*/
public Object currentIterationValue ()
{
try
{
return this.iterationList.get(this.iterationPosition);
}
catch (final ArrayIndexOutOfBoundsException e)
{
}
return null;
}
/**
* Will return true if a next iteration step is possible.
*/
public boolean hasNext ()
{
if (this.iterationList == null || this.iterationList.size() < 1)
return false;
boolean result =
(this.iterationPosition < this.iterationList.size()-1);
log.debug("hasNext() ? "+result);
return result;
}
/**
* For the given iteratingExpression and workitem, will step to
* the next iteration.
*/
public void next
(final OneChildExpression iteratingExpression,
final InFlowWorkItem wi)
throws
OpenWfeException
{
if (this.templateId == null)
this.templateId = iteratingExpression.getChildExpressionId();
iteratingExpression.tag(wi);
log.debug("next() tagged workitem");
log.debug("next() iteratingExpression is "+iteratingExpression.getId());
this.iterationPosition++;
log.debug("next() iterationPosition : "+this.iterationPosition);
log.debug("next() currentIterationValue >"+currentIterationValue()+"<");
//
// launch scope
try
{
//
// prepare workitem (set field or output variable if needed)
//
final java.util.Map vars = ValueUtils.determineAndSetTarget
("to-", // 'to-field' or 'to-variable'
iteratingExpression,
wi,
currentIterationValue());
final Launcher launcher = Definitions
.getLauncher(iteratingExpression.context());
final FlowExpressionId launchedId = launcher.launchSub
(wi,
iteratingExpression.getId(),
//null, // no 'on behalf of'
iteratingExpression.getChildExpressionId(),
vars,
true); // async launch
addChild(iteratingExpression, launchedId);
}
catch (final OpenWfeException owe)
{
throw new ApplyException
("Failed to apply next scope", owe);
}
//
// if last iteration, remove template
if ( ! this.hasNext())
{
iteratingExpression.getExpressionPool()
.removeExpression(this.templateId);
log.debug("next() removed template "+this.templateId);
}
}
//
// other METHODS
/**
* This method adds the child id to the parent only if this parent
* is an instance of SynchableExpression.
*/
protected void addChild
(final FlowExpression parent, final FlowExpressionId childId)
{
if (parent == null) log.info("addChildToParent() parent is null");
if ( ! (parent instanceof SynchableExpression)) return;
//log.debug
// ("addChildToParent() sync expression is "+
// ((SynchableExpression)parent).getSyncExpression());
((SynchableExpression)parent).getSyncExpression()
.addChild(childId);
parent.storeItself();
log.debug("addChildToParent() added "+childId+" to "+parent.getId());
}
protected void prepareIterationList
(final FlowExpression iteratingExpression,
final InFlowWorkItem wi)
throws
ApplyException
{
//
// determine on what we will iterate
Object val = null;
try
{
val = ValueUtils
.determineValue(VALUE_PREFIX, iteratingExpression, wi);
}
catch (ValueException ve)
{
throw new ApplyException
("Failed to determine value to iterate on", ve);
}
//
// determine value separator regex
String separatorRegex = (String)iteratingExpression.getAttributes()
.get(VALUE_SEPARATOR);
if (separatorRegex == null)
separatorRegex = DEFAULT_VALUE_SEPARATOR;
//
// turn the value into a list
if (val == null)
{
this.iterationList = null;
}
else if (val instanceof java.util.List)
{
this.iterationList = (java.util.List)val;
}
else if (val instanceof String ||
val instanceof StringAttribute)
{
String[] ss = val.toString().split(separatorRegex);
this.iterationList = new java.util.ArrayList(ss.length);
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy