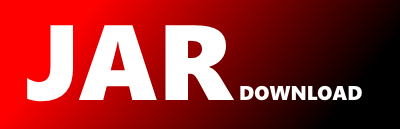
src.openwfe.org.engine.expressions.map.XmlExpressionMap Maven / Gradle / Ivy
/*
* Copyright (c) 2005, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: XmlExpressionMap.java 2385 2006-03-04 09:30:22Z jmettraux $
*/
//
// XmlExpressionMap.java
//
// [email protected]
//
// generated with
// jtmpl 1.0.04 20.11.2001 John Mettraux ([email protected])
//
package openwfe.org.engine.expressions.map;
import openwfe.org.Utils;
import openwfe.org.Parameters;
import openwfe.org.AbstractService;
import openwfe.org.ServiceException;
import openwfe.org.ApplicationContext;
import openwfe.org.xml.XmlUtils;
import openwfe.org.engine.expressions.BuildException;
import openwfe.org.engine.expressions.FlowExpression;
import openwfe.org.engine.expressions.DefineExpression;
import openwfe.org.engine.expressions.DefinitionExpression;
import openwfe.org.engine.expressions.ParticipantExpression;
import openwfe.org.engine.expressions.SubProcessRefExpression;
/**
* An expression map maps expression names to expression classes.
* For instance, the expression named 'participant' is implemented
* by the class 'openwfe.org.engine.expressions.ParticipantExpression'.
* This implementation of the interface ExpressionMap parses an XML
* document describing this mapping.
*
* CVS Info :
*
$Author: jmettraux $
*
$Date: 2006-03-04 10:30:22 +0100 (Sat, 04 Mar 2006) $
*
$Id: XmlExpressionMap.java 2385 2006-03-04 09:30:22Z jmettraux $
*
* @author [email protected]
*/
public class XmlExpressionMap
extends AbstractService
implements ExpressionMap
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(XmlExpressionMap.class.getName());
//
// CONSTANTS (definitions)
/**
* In the expression map, you need to set the RawExpression implementation
* that you use with this '__raw__' key.
*/
public final static String RAW_KEY
= "__raw__";
/**
* This parameter is used to indicate to the XmlExpressionMap where
* the XML document describing the mapping is located. The value of
* this parameter is usually a file path, but URLs are accepted.
* One could imagine a set of workflow engine sharing their expression
* map XML mapping served by one or more webservers.
*/
public final static String P_EXPRESSION_MAP_FILE
= "expressionMapFile";
//
// FIELDS
private String processDefinitionExpressionName = null;
private java.util.Map map = new java.util.HashMap();
//
// CONSTRUCTORS
public void init
(final String serviceName,
final ApplicationContext context,
final java.util.Map serviceParams)
throws
ServiceException
{
super.init(serviceName, context, serviceParams);
final String fileName = Utils.expandUrl
(context.getApplicationDirectory(),
(String)getParams().get(P_EXPRESSION_MAP_FILE));
try
{
build(XmlUtils.extractXml(fileName, false));
}
catch (final Exception e)
{
throw new ServiceException
("Failed to build XmlExpressionMap from file '"+fileName+"'",
e);
}
}
private void build (final org.jdom.Element rootElt)
{
java.util.List children = rootElt.getChildren("expression");
java.util.Iterator it = children.iterator();
while (it.hasNext())
{
org.jdom.Element child = (org.jdom.Element)it.next();
String expressionName = child.getAttributeValue("name").trim();
String className = child.getAttributeValue("class").trim();
java.util.Map params = Parameters.extractParameters(child);
try
{
final Class expressionClass = Class.forName(className);
//Entry e = new Entry(expressionName, expressionClass, params);
final Entry e = new Entry(expressionName, expressionClass);
//Definitions.getExpressionPool(getContext())
// .setVariable(null, "//"+expressionName, expressionClass);
//
// expression pool might not yet be initialized
// forget it
log.info
("loaded expression '"+expressionName+
"', class is "+className);
this.map.put(expressionName, e);
if (this.processDefinitionExpressionName == null &&
expressionClass.equals(DefineExpression.class))
{
this.processDefinitionExpressionName = expressionName;
}
}
catch (ClassNotFoundException cnfe)
{
log.info
("Class '"+className+
"' for expression '"+expressionName+
"' not found.", cnfe);
}
}
}
//
// METHODS
/**
* Since OpenWFE 1.4.8, raw expressions lies at the heart of how flows
* are built (all was done before in wfibuilder which vanished).
*/
public Class getRawExpressionClass ()
{
return getClass(RAW_KEY);
}
/**
* Instantiates an empty expression; this method is used by droflo when
* adding new expressions to a flow definition and of course by this class
* resolveExpression() method, when parsing a flow.
*/
public FlowExpression instantiateExpression (final String expressionName)
throws BuildException
{
//
// determine class
final Class expressionClass = getClass(expressionName);
if (expressionClass == null)
{
throw new BuildException
("Unmapped expression '"+expressionName+"'");
}
//
// instantiate expression
FlowExpression fe = null;
try
{
fe = (FlowExpression)expressionClass.newInstance();
}
catch (Exception e)
{
throw new BuildException
("Failed to build expression '"+
expressionName+"' with class '"+
expressionClass.getName()+"'",
e);
}
return fe;
}
/**
* Returns the class associated with the name of the expression found
* in the WFD
*/
public Class getClass (final String expressionName)
{
//log.debug("getClass() for '"+expressionName+"'");
Entry e = (Entry)this.map.get(expressionName);
//log.debug("getClass() found entry");
if (e == null) return null;
//log.debug("getClass() not null : "+e.expressionClass);
//log.debug("getClass() not null : "+e.expressionClass.getName());
return e.expressionClass;
}
/* *
* returns the params associated with a given expression name
* /
public java.util.Map getParams (final String expressionName)
{
Entry e = (Entry)this.map.get(expressionName);
if (e == null) return null;
return e.params;
}
*/
/**
* A droflo method ; it returns the short expression name associated
* with an expression class.
*/
public String getName (final Class expressionClass)
{
/*
* hardcoded tricks...
* should vanish later... (2005/01/17)
*/
if (expressionClass.equals(DefineExpression.class))
return this.processDefinitionExpressionName;
if (expressionClass.equals(ParticipantExpression.class))
return "participant";
java.util.Iterator it = this.map.keySet().iterator();
while (it.hasNext())
{
String name = (String)it.next();
Entry e = (Entry)this.map.get(name);
if (e.expressionClass.equals(expressionClass)) return name;
}
return null;
}
/**
* Returns a table of the names of the expression implementing/extending
* the given expression class.
*/
public String[] getNames (final Class expressionClass)
{
log.debug("getNames() for class "+expressionClass.getName());
final java.util.List result = new java.util.ArrayList(this.map.size());
java.util.Iterator it = this.map.keySet().iterator();
while (it.hasNext())
{
final String name = (String)it.next();
final Entry e = (Entry)this.map.get(name);
//if (e.expressionClass.equals(expressionClass)) return name;
if (expressionClass.isAssignableFrom(e.expressionClass))
{
result.add(e.shortName);
log.debug("getNames() - '"+e.shortName+"'");
}
}
final String[] sResult = new String[result.size()];
int i = 0;
it = result.iterator();
while (it.hasNext())
{
sResult[i] = (String)it.next();
i++;
}
return sResult;
}
/**
* A droflo/jgpd method : returns the keys (the set of expression
* names configured in this map)
*/
public java.util.Set keySet ()
{
return this.map.keySet();
}
//
// INNER CLASSES
private class Entry
{
public String shortName = null;
public Class expressionClass = null;
//public java.util.Map params = null;
public Entry
(final String shortName,
final Class expressionClass)
//final java.util.Map params)
{
this.shortName = shortName;
this.expressionClass = expressionClass;
//this.params = params;
}
}
}