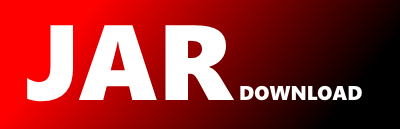
src.openwfe.org.engine.expressions.state.FrozenState Maven / Gradle / Ivy
/*
* Copyright (c) 2005, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: FrozenState.java 2426 2006-03-14 13:44:30Z jmettraux $
*/
//
// FrozenState.java
//
// [email protected]
//
// generated with
// jtmpl 1.1.01 2004/05/19 ([email protected])
//
package openwfe.org.engine.expressions.state;
import openwfe.org.engine.expool.ExpressionPool;
import openwfe.org.engine.workitem.InFlowWorkItem;
import openwfe.org.engine.expressions.FlowExpression;
import openwfe.org.engine.expressions.ApplyException;
import openwfe.org.engine.expressions.ReplyException;
/**
* A paused expression will be transparent, the flow will run trough it as
* if it was not here.
*
* CVS Info :
*
$Author: jmettraux $
*
$Id: FrozenState.java 2426 2006-03-14 13:44:30Z jmettraux $
*
* @author [email protected]
*/
public class FrozenState
extends AbstractExpressionState
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(FrozenState.class.getName());
//
// CONSTANTS & co
/**
* A constant for [Text|Sql]History : "+FRZ"
*/
public final static String EVT_FREEZE
= "+FRZ";
/**
* A constant for [Text|Sql]History : "+UFZ"
*/
public final static String EVT_UNFREEZE
= "+UFZ";
private final static String NAME = "frozen";
private final static char IDENTIFIER = 'f';
//
// FIELDS
private InFlowWorkItem appliedItem = null;
private java.util.List replyQueue = null;
//
// CONSTRUCTORS
//
// BEAN METHODS
/**
* Returns a potential applied and thus frozen workitem
*/
public InFlowWorkItem getAppliedItem () { return this.appliedItem; }
/**
* Returns the list of workitems that got successively replied to
* the frozen expression.
*/
public java.util.List getReplyQueue () { return this.replyQueue; }
public void setAppliedItem (final InFlowWorkItem wi)
{
this.appliedItem = wi;
}
public void setReplyQueue (final java.util.List rq)
{
this.replyQueue = rq;
}
//
// METHODS from ExpressionState
/**
* Will return 'frozen'.
*/
public String getName ()
{
return NAME;
}
/**
* Returns a one char identifier for the state (for example 'f' for frozen)
*/
public char getIdentifier ()
{
return IDENTIFIER;
}
public void apply (final InFlowWorkItem wi)
throws ApplyException
{
if (this.appliedItem != null)
{
throw new ApplyException
("An expression should not get applied twice.");
}
this.appliedItem = (InFlowWorkItem)wi.clone();
log.debug
("apply() applied item lastExpressionId : "+
this.appliedItem.getLastExpressionId());
this.expression.storeItself();
}
public void reply (final InFlowWorkItem wi)
throws ReplyException
{
log.debug("reply() freezing incoming wi");
if (this.replyQueue == null)
this.replyQueue = new java.util.LinkedList();
this.replyQueue.add(wi);
this.expression.storeItself();
}
public InFlowWorkItem cancel ()
throws ApplyException
{
this.expression.cancel();
return this.appliedItem;
//
// the applied item may be null, the replied items
// are not taken into account.
}
/**
* Makes this expression leave this state.
*/
public void exitState ()
throws ApplyException
{
this.expression.setState(null);
//this.expression.storeItself();
//
// should not be necessary as apply() or reply() will induce
// it somehow
if (this.appliedItem == null && this.replyQueue == null)
{
log.debug("exitState() simple unfreeze done.");
return;
}
if (this.replyQueue == null)
//
// apply
{
log.debug
("exitState() applying (exp) "+
this.expression.getId());
log.debug
("exitState() applying (itm) "+
this.appliedItem.getLastExpressionId());
this.expression.apply(this.appliedItem);
}
else
//
// reply
{
log.debug("exitState() replying to "+this.expression.getId());
final java.util.Iterator it = this.replyQueue.iterator();
while (it.hasNext())
{
final InFlowWorkItem wi = (InFlowWorkItem)it.next();
try
{
this.expression.reply(wi);
}
catch (final ReplyException re)
{
log.warn
("exitState() failure during 'reply' defreeze", re);
}
}
}
}
//
// STATIC METHODS
}