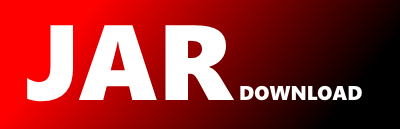
src.openwfe.org.engine.workitem.XmlAttribute Maven / Gradle / Ivy
/*
* Copyright (c) 2005, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: XmlAttribute.java 2257 2005-12-05 17:47:24Z jmettraux $
*/
//
// XmlAttribute.java
//
// [email protected]
//
// generated with
// jtmpl 1.0.04 31.10.2002 John Mettraux ([email protected])
//
package openwfe.org.engine.workitem;
import openwfe.org.ApplicationContext;
import openwfe.org.xml.XmlUtils;
import openwfe.org.engine.Definitions;
import openwfe.org.engine.impl.workitem.xml.XmlWorkItemCoder;
/**
* A XmlAttribute is either the name of a workitem field or its value.
* It's an encapsulation of a Java String.
*
* CVS Info :
*
$Author: jmettraux $
*
$Date: 2005-12-05 18:47:24 +0100 (Mon, 05 Dec 2005) $
*
$Id: XmlAttribute.java 2257 2005-12-05 17:47:24Z jmettraux $
*
* @author [email protected]
*/
public class XmlAttribute
extends AtomicAttribute
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(XmlAttribute.class.getName());
//
// FIELDS
//
// CONSTRUCTORS
/**
* Builds an empty XmlAttribute, value set to "".
*/
public XmlAttribute ()
{
super(null);
}
/* *
* The object awaited is an instance of org.jdom.Content
* /
public XmlAttribute (final Object o)
{
super(o);
}
*/
/**
* Builds a new XmlAttribute
*/
public XmlAttribute (final org.jdom.Content c)
{
super(c);
}
//
// METHODS
/**
* Returns the JDOM content instance encapsulated in this attribute.
*/
public org.jdom.Content getContent ()
{
return (org.jdom.Content)getValue();
//return (org.jdom.Content)((org.jdom.Content)getValue()).clone();
}
/**
* Simply returns the wrapped String.
*/
public String toString ()
{
if (getValue() == null) return "";
String s = XmlUtils.xmlToString(getContent());
return XmlUtils.removeHeaderLine(s);
}
/**
* Returns a copy of this XmlAttribute.
*/
public Object clone ()
{
if (getValue() == null)
return new XmlAttribute();
return new XmlAttribute((org.jdom.Content)getContent().clone());
}
/**
* Attempts to translate the XML content to an OpenWFE attribute.
* Will return null if the content is null of if the content
* doesn't decode to an attribute.
*
* @param context this method needs it to lookup for the XmlAttributeCoder
*/
public Attribute getAttributeContent (final ApplicationContext context)
{
if ( ! (getContent() instanceof org.jdom.Element))
return null;
final XmlWorkItemCoder coder = (XmlWorkItemCoder)Definitions
.getXmlCoder(context);
Attribute a = null;
try
{
a = coder.decodeAttribute((org.jdom.Element)getContent());
}
catch (final Throwable t)
{
log.debug
("getAttributeContent() no attribute in "+
XmlUtils.xmlToString(getContent()));
}
return a;
}
//
// STATIC METHODS
private static int fetchIndex (final String key)
{
int childId = -1;
try
{
childId = Integer.parseInt(key);
}
catch (final NumberFormatException nfe)
{
// ignore, childId still at -1
}
return childId;
}
private static org.jdom.Content lookupChild
(final String key, final org.jdom.Element elt)
{
final int childId = fetchIndex(key);
if (childId > -1)
{
if (childId > elt.getChildren().size()-1) return null;
return (org.jdom.Content)elt.getChildren().get(childId);
}
return elt.getChild(key);
}
private static org.jdom.Content lookupContent
(final String key, final org.jdom.Element elt)
{
final int childId = fetchIndex(key);
if (childId < 0) return null;
if (childId > elt.getContent().size()-1) return null;
return (org.jdom.Content)elt.getContent().get(childId);
}
private static org.jdom.Content lookupItem
(final String key, final org.jdom.Element elt)
{
char c0 = key.charAt(0);
Character c1 = null;
if (key.length() > 1) c1 = new Character(key.charAt(1));
if (Character.isDigit(c0))
{
return lookupContent(key, elt);
}
if (c1 == null && Character.isDigit(c0))
//
// content lookup (by index)
//
{
return lookupContent(key, elt);
}
if (c1 == null && Character.isLetter(c0))
//
// child lookup (by name)
//
{
return lookupChild(key, elt);
}
if (c0 == 'e' && Character.isDigit(c1.charValue()))
//
// child lookup (by index)
//
{
return lookupChild(key.substring(1), elt);
}
if (c0 == '@')
//
// attribute lookup
//
{
final String sValue = elt.getAttributeValue(key.substring(1));
if (sValue == null) return null;
return new org.jdom.Text(sValue);
}
//
// child lookup (by name)
return lookupChild(key, elt);
}
/**
* Returns a precise part of an XML tree
*/
public static org.jdom.Content lookupInXml
(final String key, final org.jdom.Content c)
{
if (key.equals("/") || key.equals(""))
return (org.jdom.Content)c.clone();
if ( ! (c instanceof org.jdom.Element)) return null;
final org.jdom.Element e = (org.jdom.Element)c;
final int i = key.indexOf(".");
log.debug("lookupInXml() i = "+i);
if (i < 0) return lookupItem(key, e);
//if (i >= key.length()-1) return new org.jdom.Text(e.getText());
final String scar = key.substring(0, i);
final String scdr = key.substring(i+1);
log.debug("lookupInXml() car >"+scar+"< cdr >"+scdr+"<");
final org.jdom.Content cCar = lookupItem(scar, e);
if ( ! (cCar instanceof org.jdom.Element)) return null;
final org.jdom.Element eCar = (org.jdom.Element)cCar;
if (scdr.equals(""))
return new org.jdom.Text(eCar.getText());
return lookupInXml(scdr, (org.jdom.Element)cCar);
}
private static Attribute toAttribute (final org.jdom.Content c)
{
if (c == null) return null;
if (c instanceof org.jdom.Text)
return new StringAttribute(((org.jdom.Text)c).getText());
return new XmlAttribute((org.jdom.Content)c.clone());
}
public static Attribute lookupAsAttribute
(final String key, final org.jdom.Content c)
{
return toAttribute(lookupInXml(key, c));
}
}