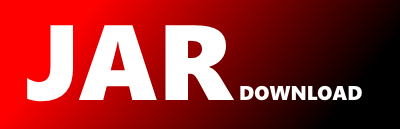
src.openwfe.org.engine.control.auth.ControlPermission Maven / Gradle / Ivy
/*
* Copyright (c) 2005, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: ControlPermission.java 1882 2005-05-17 16:41:07Z jmettraux $
*/
//
// ControlPermission.java
//
// [email protected]
//
// generated with
// jtmpl 1.0.04 31.10.2002 John Mettraux ([email protected])
//
package openwfe.org.engine.control.auth;
import java.security.PermissionCollection;
import openwfe.org.auth.Permission;
import openwfe.org.auth.AuthException;
/**
* A permission for a workitem store
*
* CVS Info :
*
$Author: jmettraux $
*
$Date: 2005-05-17 18:41:07 +0200 (Tue, 17 May 2005) $
*
$Id: ControlPermission.java 1882 2005-05-17 16:41:07Z jmettraux $
*
* @author [email protected]
*/
public class ControlPermission
extends Permission
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(ControlPermission.class.getName());
//
// CONSTANTS (definitions)
public final static String P_RIGHTS
= "rights";
public final static String P_ACTIONS
= "actions";
private final static int NONE = 0x0;
private final static int READ = 0x1;
private final static int FREEZE = 0x2;
private final static int CANCEL = 0x4;
private final static int ALL = READ | FREEZE | CANCEL;
private final static String S_READ = "read";
private final static String S_FREEZE = "freeze";
private final static String S_CANCEL = "cancel";
//
// FIELDS
private int mask = NONE;
private transient String action = null;
//
// CONSTRUCTORS
public ControlPermission (final java.util.Map params)
{
super(params);
String actions = (String)params.get(P_RIGHTS);
if (actions == null) actions = (String)params.get(P_ACTIONS);
this.mask = getMask(actions);
this.action = null; // so that it gets recomputed
//log.debug("ControlPermission() : "+this.toString());
}
/**
* a helper method
*/
public static ControlPermission newControlPermission
(final String name, final String actions)
{
final java.util.Map params = new java.util.HashMap(2);
params.put(NAME, name);
params.put(P_RIGHTS, actions);
return new ControlPermission(params);
}
//
// METHODS
public int getMask ()
{
return this.mask;
}
//
// METHODS from Permission
public int hashCode ()
{
return (this.getName().hashCode() ^ this.mask);
}
public boolean equals (Object o)
{
if ( ! (o instanceof ControlPermission)) return false;
ControlPermission other = (ControlPermission)o;
return (this.mask == other.mask);
//
// control permissions do not care about names
//
//return
// (getName().equals(other.getName()) &&
// this.mask == other.mask);
}
public boolean implies (java.security.Permission p)
{
if (p == null || ! (p instanceof ControlPermission)) return false;
//log.debug("does "+this+" implies "+p+" ?");
//
// control permissions do not care about names
//
//if ( ! namesMatch(this.getName(), p.getName())) return false;
//
// check the actions
ControlPermission sp = (ControlPermission)p;
//log.debug("result is "+((this.mask & sp.mask) == sp.mask));
return ((this.mask & sp.mask) == sp.mask);
}
public PermissionCollection newPermissionCollection ()
{
return new ControlPermissionCollection();
}
public String toString ()
{
StringBuffer sb = new StringBuffer();
sb.append(" ");
return sb.toString();
}
//
// some static METHODS
protected static String removeWildcard (String s)
{
if (s.length() < 1) return ".";
if ( ! s.endsWith("*")) return (s + '!');
return s.substring(0, s.length()-1);
}
public static boolean namesMatch (String n1, String n2)
{
n1 = removeWildcard(n1);
n2 = removeWildcard(n2);
return n2.startsWith(n1);
}
public static int getMask (String actions)
{
int mask = NONE;
if (actions == null) return mask;
//log.debug("getMask() actions >"+actions+"<");
final String[] actionArray = actions.toLowerCase().split(",");
for (int i=0; i < actionArray.length; i++)
{
String action = actionArray[i].trim();
//log.debug("getMask() parsed action >"+action+"<");
if (action.equals(S_READ))
{
mask |= READ;
}
else if (action.equals(S_FREEZE))
{
mask |= FREEZE;
}
else if (action.equals(S_CANCEL))
{
mask |= CANCEL;
}
}
//log.debug("getMask() mask is "+mask);
return mask;
}
public String getActions ()
{
if (this.action == null)
{
StringBuffer result = new StringBuffer();
if ((this.mask & READ) == READ)
result.append(S_READ);
if ((this.mask & FREEZE) == FREEZE)
{
if (result.length() > 0) result.append(",");
result.append(S_FREEZE);
}
if ((this.mask & CANCEL) == CANCEL)
{
if (result.length() > 0) result.append(",");
result.append(S_CANCEL);
}
this.action = result.toString();
}
return this.action;
}
}
//
// ANOTHER CLASS
//
// ControlPermissionCollection.java
//
// [email protected]
//
// generated with
// jtmpl 1.0.04 31.10.2002 John Mettraux ([email protected])
//
/**
* no comment
*
*
CVS Info :
*
$Author: jmettraux $
*
$Date: 2005-05-17 18:41:07 +0200 (Tue, 17 May 2005) $
*
$Id: ControlPermission.java 1882 2005-05-17 16:41:07Z jmettraux $
*
* @author [email protected]
*/
final class ControlPermissionCollection
extends PermissionCollection
implements java.io.Serializable
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(ControlPermissionCollection.class.getName());
//
// FIELDS
private java.util.Vector permissions = new java.util.Vector();
//
// CONSTRUCTORS
//
// METHODS
public void add (java.security.Permission p)
{
if (p == null || ! (p instanceof ControlPermission))
{
throw new IllegalArgumentException
("null permission or permission not of class '"+
ControlPermission.class.getName()+"'");
}
if (isReadOnly())
{
throw new SecurityException
("attempt to add a Permission to a readonly "+
"PermissionCollection");
}
permissions.add(p);
}
public boolean implies (java.security.Permission p)
{
if (p == null || ! (p instanceof ControlPermission)) return false;
//log.debug("implies() does collection implies "+p.toString());
ControlPermission sp = (ControlPermission)p;
int desiredMask = sp.getMask();
int effectiveMask = 0;
int neededMask = desiredMask;
java.util.Enumeration en = elements();
while(en.hasMoreElements())
{
ControlPermission esp = (ControlPermission)en.nextElement();
//log.debug("implies() does "+esp+" implies "+p+" ?");
//if (((neededMask & esp.getMask()) != 0) &&
// ControlPermission.namesMatch(p.getName(), esp.getName()))
if ((neededMask & esp.getMask()) != 0)
{
effectiveMask |= esp.getMask();
if ((effectiveMask & desiredMask) == desiredMask)
{
//log.debug("implies() true!");
return true;
}
neededMask = (desiredMask ^ effectiveMask);
}
}
//log.debug("implies() false!");
return false;
}
public java.util.Enumeration elements ()
{
return permissions.elements();
}
}