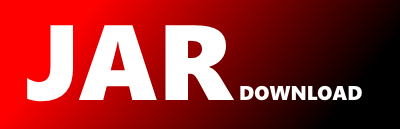
src.openwfe.org.engine.expressions.FlowExpressionId Maven / Gradle / Ivy
/*
* Copyright (c) 2001-2006, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: FlowExpressionId.java 2902 2006-07-01 13:31:15Z jmettraux $
*/
//
// FlowExpressionId.java
//
// [email protected]
//
// generated with
// jtmpl 1.0.04 20.11.2001 John Mettraux ([email protected])
//
package openwfe.org.engine.expressions;
import openwfe.org.Utils;
//import openwfe.org.ApplicationContext;
//import openwfe.org.time.Scheduler;
//import openwfe.org.time.Schedulable;
//import openwfe.org.engine.Definitions;
import openwfe.org.engine.workitem.Attribute;
/**
* A unique identifier for an expression
*
* CVS Info :
*
$Author: jmettraux $
*
$Date: 2006-07-01 15:31:15 +0200 (Sat, 01 Jul 2006) $
*
$Id: FlowExpressionId.java 2902 2006-07-01 13:31:15Z jmettraux $
*
* @author [email protected]
*/
public class FlowExpressionId
//implements java.io.Serializable, Cloneable
//implements Attribute, Schedulable
implements Attribute
{
static final long serialVersionUID = -6456469702585696792L;
/*
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(FlowExpressionId.class.getName());
*/
//
// CONSTANTS and co
//
// FIELDS
private String engineId = null;
private String initialEngineId = null;
private String workflowDefinitionUrl = null;
private String workflowDefinitionName = null;
private String workflowDefinitionRevision = null;
private String workflowInstanceId = null;
private String expressionName = null;
private String expressionId = null;
private String owfeVersion = null;
//
// CONSTRUCTORS
//
// BEAN METHODS
/**
* Returns the identifier of an engine whom the expression/workitem belongs
* to. This id corresponds to an entry in the participant map
* (etc/engine/participant-map.xml)
*/
public String getEngineId () { return this.engineId; }
/**
* Returns the engineId (participant name) of the engine on which the
* expression got created).
* This id corresponds to an entry in the participant map
* (etc/engine/participant-map.xml)
*/
public String getInitialEngineId () { return this.initialEngineId; }
public String getWorkflowDefinitionUrl () { return this.workflowDefinitionUrl; }
public String getWorkflowDefinitionName () { return this.workflowDefinitionName; }
public String getWorkflowDefinitionRevision () { return this.workflowDefinitionRevision; }
public String getWorkflowInstanceId () { return this.workflowInstanceId; }
public String getExpressionName () { return this.expressionName; }
public String getExpressionId () { return this.expressionId; }
public String getOwfeVersion () { return this.owfeVersion; }
//
// setters
public void setEngineId (String s) { this.engineId = s; }
public void setInitialEngineId (String s) { this.initialEngineId = s; }
public void setWorkflowDefinitionUrl (String s) { this.workflowDefinitionUrl = s; }
public void setWorkflowDefinitionName (String s) { this.workflowDefinitionName = s; }
public void setWorkflowDefinitionRevision (String s) { this.workflowDefinitionRevision = s; }
public void setWorkflowInstanceId (String s) { this.workflowInstanceId = s; }
public void setExpressionName (String s) { this.expressionName = s; }
public void setExpressionId (String s) { this.expressionId = s; }
public void setOwfeVersion (String s) { this.owfeVersion = s; }
//
// METHODS from Schedulable
/* *
* This method is called by the Scheduler instance to wake up and
* /
public void trigger (final Object[] params)
{
final ApplicationContext ac = (ApplicationContext)params[0];
Definitions.getExpressionPool(ac).trigger(new Object[] { this });
}
/* *
* Never called, not implemented thus.
* /
public Long reschedule (final Scheduler s)
{
return null;
}
*/
//
// METHODS
/**
* Returns the workflowInstanceId without any subflow ids
*/
public String getParentWorkflowInstanceId ()
{
return extractParentWorkflowInstanceId(getWorkflowInstanceId());
}
/**
* A static method for removing the subflow ids in a workflowInstanceId,
* turning "1232132.0.4" into "12321322" for example.
*/
public static String extractParentWorkflowInstanceId
(final String workflowInstanceId)
{
final int i = workflowInstanceId.indexOf(".");
if (i < 0) return workflowInstanceId;
return workflowInstanceId.substring(0, i);
}
public boolean equals (final Object o)
{
if ( ! (o instanceof FlowExpressionId)) return false;
FlowExpressionId fei = (FlowExpressionId)o;
return
Utils.stringEquals(this.engineId, fei.engineId) &&
Utils.stringEquals(this.initialEngineId, fei.initialEngineId) &&
Utils.stringEquals(this.workflowInstanceId, fei.workflowInstanceId) &&
Utils.stringEquals(this.expressionId, fei.expressionId) &&
Utils.stringEquals(this.expressionName, fei.expressionName) &&
Utils.stringEquals(this.workflowDefinitionName, fei.workflowDefinitionName) &&
Utils.stringEquals(this.workflowDefinitionRevision, fei.workflowDefinitionRevision) &&
Utils.stringEquals(this.owfeVersion, fei.owfeVersion);
}
/**
* Returns true if this expression id points to an expression that is part
* of a subflow.
*/
public boolean isInSubFlow ()
{
if (this.workflowInstanceId == null) return false;
return (this.workflowInstanceId.indexOf(".") > -1);
}
public int hashCode ()
{
return this.toString().hashCode();
}
public Object clone ()
{
FlowExpressionId result = new FlowExpressionId();
result.engineId =
Utils.copyString(this.engineId);
result.initialEngineId =
Utils.copyString(this.initialEngineId);
result.workflowDefinitionUrl =
Utils.copyString(this.workflowDefinitionUrl);
result.workflowDefinitionName =
Utils.copyString(this.workflowDefinitionName);
result.workflowDefinitionRevision =
Utils.copyString(this.workflowDefinitionRevision);
result.workflowInstanceId =
Utils.copyString(this.workflowInstanceId);
result.expressionName =
Utils.copyString(this.expressionName);
result.expressionId =
Utils.copyString(this.expressionId);
result.owfeVersion =
Utils.copyString(this.owfeVersion);
return result;
}
/**
* A shortcut for clone, without the casting need.
*/
public FlowExpressionId copy ()
{
return (FlowExpressionId)this.clone();
}
/**
* The classical toString() method : returns a human readable version of
* this flowExpressionId.
*/
public String toString ()
{
StringBuffer sb = new StringBuffer();
sb
.append("( fei ")
.append(this.owfeVersion)
.append(" ")
.append(this.engineId)
.append("/")
.append(this.initialEngineId)
.append(" ")
.append(this.workflowDefinitionUrl)
.append(" ")
.append(this.workflowDefinitionName)
.append(" ")
.append(this.workflowDefinitionRevision)
.append(" ")
.append(this.workflowInstanceId)
.append(" ")
.append(this.expressionName)
.append(" ")
.append(this.expressionId)
.append(" )");
return sb.toString();
}
/**
* Produces a 'summarized' string id of this flow expression id, it's used
* in rest sessions to lighten the info transmitted.
*/
public String asStringId ()
{
final StringBuffer sb = new StringBuffer();
sb.append(getEngineId());
sb.append("_");
sb.append(getWorkflowInstanceId());
sb.append("_");
sb.append(getExpressionName());
sb.append("_");
sb.append(getExpressionId());
return sb.toString();
}
/**
* Turns this expression id into a parseable string
*/
public String toParseableString ()
{
final StringBuffer sb = new StringBuffer();
sb
.append(normalize(this.owfeVersion))
.append("|")
.append(normalize(this.engineId))
.append("|")
.append(normalize(this.initialEngineId))
.append("|")
.append(normalize(this.workflowDefinitionUrl))
.append("|")
.append(normalize(this.workflowDefinitionName))
.append("|")
.append(normalize(this.workflowDefinitionRevision))
.append("|")
.append(normalize(this.workflowInstanceId))
.append("|")
.append(normalize(this.expressionName))
.append("|")
.append(normalize(this.expressionId));
return sb.toString();
}
private String normalize (final String in)
{
if (in == null) return "";
return in;
}
/**
* Parses a string generated with toParseableString() into
* its corresponding FlowExpressionId.
*/
public static FlowExpressionId fromParseableString (String s)
{
if (s == null) return null;
if (s.endsWith("|")) s = s + " ";
final String[] ss = s.split("\\|");
if (ss.length != 8 && ss.length != 9)
{
throw new IllegalArgumentException
("Cannot parse FlowExpressionId out of >"+s+
"< (length is "+ss.length+")");
}
final FlowExpressionId fei = new FlowExpressionId();
int i = 0;
if (ss.length == 9)
fei.owfeVersion = ss[i++].trim();
else
fei.owfeVersion = "unknown";
fei.engineId = ss[i++].trim();
fei.initialEngineId = ss[i++].trim();
fei.workflowDefinitionUrl = ss[i++].trim();
fei.workflowDefinitionName = ss[i++].trim();
fei.workflowDefinitionRevision = ss[i++].trim();
fei.workflowInstanceId = ss[i++].trim();
fei.expressionName = ss[i++].trim();
fei.expressionId = ss[i++].trim();
return fei;
}
//
// STATIC METHODS
}