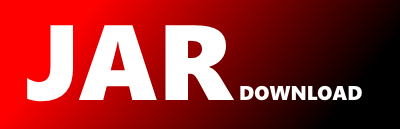
src.openwfe.org.engine.functions.AbstractFunctionMap Maven / Gradle / Ivy
/*
* Copyright (c) 2005, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: AbstractFunctionMap.java 2838 2006-06-18 20:28:08Z jmettraux $
*/
//
// AbstractFunctionMap.java
//
// [email protected]
//
// generated with
// jtmpl 1.1.01 2004/05/19 ([email protected])
//
package openwfe.org.engine.functions;
import java.lang.reflect.Method;
import openwfe.org.AbstractService;
import openwfe.org.ReflectionUtils;
import openwfe.org.ServiceException;
import openwfe.org.ApplicationContext;
import openwfe.org.engine.workitem.InFlowWorkItem;
import openwfe.org.engine.workitem.StringMapAttribute;
import openwfe.org.engine.expressions.FlowExpression;
/**
* The beginning of the implementation of a FunctionMap, it implements
* the eval job, but not the parsing of the function map itself, may it
* be XML or whatever.
*
* CVS Info :
*
$Author: jmettraux $
*
$Id: AbstractFunctionMap.java 2838 2006-06-18 20:28:08Z jmettraux $
*
* @author [email protected]
*/
public abstract class AbstractFunctionMap
extends AbstractService
implements FunctionMap
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(AbstractFunctionMap.class.getName());
//
// CONSTANTS & co
private final static Class[] FUNCTION_ARG_TYPES =
new Class[]
{
FlowExpression.class,
InFlowWorkItem.class,
String[].class
};
//
// FIELDS
private java.util.List classes = null;
private java.util.Map functionMap = null;
//
// CONSTRUCTORS
/**
* Builds this service (this is called by ApplicationContext upon
* parsing a configuration file)
*/
public void init
(final String serviceName,
final ApplicationContext context,
final java.util.Map serviceParams)
throws
ServiceException
{
super.init(serviceName, context, serviceParams);
buildMap();
}
//
// METHODS from FunctionMap
protected java.util.List getClasses () { return this.classes; }
protected java.util.Map getFunctionMap () { return this.functionMap; }
protected void setClasses (java.util.List l) { this.classes = l; }
protected void setFunctionMap (java.util.Map m) { this.functionMap = m; }
/*
* Iterates through the classes registered in this map to find a given
* function by its name.
*/
private Class findClass (final String functionName)
{
final java.util.Iterator it = this.classes.iterator();
while (it.hasNext())
{
final Class clazz = (Class)it.next();
try
{
final Method m = clazz
.getMethod(functionName, FUNCTION_ARG_TYPES);
return clazz;
}
catch (final Throwable t)
{
log.debug
("findClass() did not find class for function named '"+
functionName+"' in class '"+clazz.getName()+"'");
// continue;
}
}
return null;
}
/**
* This is the main method of a FunctionMap, given a functionName, a
* workitem and an array of args.
* Basic functions like "today()" do not care about args or the workitem.
*/
public String eval
(final String functionName,
final FlowExpression fe,
final InFlowWorkItem wi,
final String[] args)
{
Class clazz = (Class)this.functionMap.get(functionName);
if (clazz == null)
{
clazz = findClass(functionName);
if (clazz == null)
{
log.info
("eval() did not find any class for function '"+
functionName+"'");
//return "";
return "(unknown function '"+functionName+"')";
}
//log.debug("eval() class holding function is "+clazz.getName());
this.functionMap.put(functionName, clazz);
// cache
}
Throwable t = null;
try
{
return (String)ReflectionUtils.invokeStatic
(clazz,
functionName,
FUNCTION_ARG_TYPES,
new Object[] { fe, wi, args });
}
catch (final java.lang.reflect.InvocationTargetException ite)
{
t = ite.getCause();
}
catch (final Throwable tt)
{
t = tt;
}
log.warn("eval() failed for '"+functionName+"' at "+fe.getId(), t);
wi.getAttributes().puts(F_FUNCTION_ERROR, t.toString());
return "";
}
//
// METHODS
//
// METHODS from Service
//
// ABSTRACT METHODS
/**
* This method has to be implemented in order to have a full featured
* FunctionMap, it tells how to look for classes containing function
* implementations.
*/
protected abstract void buildMap () throws ServiceException;
//
// STATIC METHODS
}