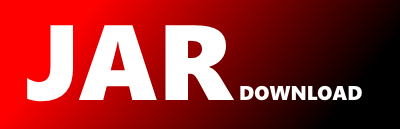
src.openwfe.org.engine.impl.functions.DateTimeFunctions Maven / Gradle / Ivy
/*
* Copyright (c) 2001-2006, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: DateTimeFunctions.java 2838 2006-06-18 20:28:08Z jmettraux $
*/
//
// DateTimeFunctions.java
//
// [email protected]
//
// generated with
// jtmpl 1.1.01 2004/05/19 ([email protected])
//
package openwfe.org.engine.impl.functions;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import openwfe.org.Utils;
import openwfe.org.time.Time;
import openwfe.org.engine.Definitions;
import openwfe.org.engine.expool.ExpressionPool;
import openwfe.org.engine.workitem.InFlowWorkItem;
import openwfe.org.engine.expressions.FlowExpression;
/**
* Functions about date and time.
*
* CVS Info :
*
$Author: jmettraux $
*
$Id: DateTimeFunctions.java 2838 2006-06-18 20:28:08Z jmettraux $
*
* @author [email protected]
*/
public abstract class DateTimeFunctions
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(DateTimeFunctions.class.getName());
//
// CONSTANTS & co
private static SimpleDateFormat ts_shortSdf =
new SimpleDateFormat("yyyyMMdd");
private static SimpleDateFormat ts_longSdf =
new SimpleDateFormat("yyyyMMddhhmmss");
private static SimpleDateFormat ts_veryLongSdf =
new SimpleDateFormat("yyyyMMddhhmmssSSS");
//
// static methods (FUNCTIONS)
/**
* This function will return the current time as an ISO full date.
*/
public static String now
(final FlowExpression fe, final InFlowWorkItem wi, final String[] args)
{
return Time.toIsoDate();
}
/**
* This function will return the current date as an ISO date.
*/
public static String today
(final FlowExpression fe, final InFlowWorkItem wi, final String[] args)
{
final String sNow = Time.toIsoDate();
final int i = sNow.indexOf(" ");
return sNow.substring(0, i);
}
/**
* Attempts to parse a date in any standard format and turn it into
* a ISO (8601) formatted date.
* Such a date is then readable by expressions like
* <sleep until="ddd" />.
*/
public static String toIsoDate
(final FlowExpression fe, final InFlowWorkItem wi, final String[] args)
{
if (args.length < 1)
//
// return now()
//
return Time.toIsoDate();
if (args.length > 1)
{
try
{
final SimpleDateFormat sdf = new SimpleDateFormat(args[1]);
final java.util.Date d = sdf.parse(args[0]);
return Time.toIsoDate(d.getTime());
}
catch (final Throwable t)
{
return
"cannot parse date '"+args[0]+
"' with format '"+args[1]+"'";
}
}
log.debug("toIsoDate() attempting to parse \""+args[0]+"\"");
final java.util.Date d = Time.parseDate(args[0]);
if (d == null) return "";
return Time.toIsoDate(d.getTime());
}
/**
* An alias for the function 'toIsoDate'.
*/
public static String date
(final FlowExpression fe, final InFlowWorkItem wi, final String[] args)
{
return toIsoDate(fe, wi, args);
}
/**
* An alias for timeAdd.
*/
public static String tadd
(final FlowExpression fe, final InFlowWorkItem wi, final String[] args)
{
return timeAdd(fe, wi, args);
}
/**
* This function takes two arguments : an iso date and a time duration,
* it then returns the date + the time duration.
*/
public static String timeAdd
(final FlowExpression fe, final InFlowWorkItem wi, final String[] args)
{
if (args.length < 2)
{
throw new IllegalArgumentException
("timeAdd() awaits 2 args.");
}
final String sDate = args[0];
String sDuration = args[1];
int dmod = +1;
if (sDuration.startsWith("-"))
{
sDuration = sDuration.substring(1);
dmod = -1;
}
long date = 0;
try
{
date = Time.fromIsoDate(sDate);
}
catch (final ParseException pe)
{
throw new IllegalArgumentException
("Failed to parse date '"+sDate+"'");
}
final long duration = dmod * Time.parseTimeString(sDuration);
return Time.toIsoDate(date + duration);
}
/**
* Short time stamp : outputs the timestamp in the
* form "yyyyMMdd".
*/
public static String ststamp
(final FlowExpression fe, final InFlowWorkItem wi, final String[] args)
{
return ts_shortSdf.format(new java.util.Date());
}
/**
* Long time stamp : outputs the timestamp in the
* form "yyyyMMddhhmmss".
*/
public static String ltstamp
(final FlowExpression fe, final InFlowWorkItem wi, final String[] args)
{
return ts_longSdf.format(new java.util.Date());
}
/**
* An alias for 'vltstamp'.
*/
public static String timestamp
(final FlowExpression fe, final InFlowWorkItem wi, final String[] args)
{
return vltstamp(fe, wi, args);
}
/**
* Very long time stamp : outputs the timestamp in the
* form "yyyyMMddhhmmssSSS".
*/
public static String vltstamp
(final FlowExpression fe, final InFlowWorkItem wi, final String[] args)
{
return ts_veryLongSdf.format(new java.util.Date());
}
}