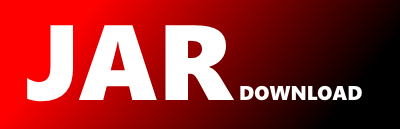
src.openwfe.org.engine.impl.participants.CompositeParticipantMap Maven / Gradle / Ivy
/*
* Copyright (c) 2001-2006, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: FileArchivingParticipant.java 2426 2006-03-14 13:44:30Z jmettraux $
*/
//
// CompositeParticipantMap.java
//
// [email protected]
//
// generated with
// jtmpl 1.1.01 2004/05/19 ([email protected])
//
package openwfe.org.engine.impl.participants;
import openwfe.org.ServiceException;
import openwfe.org.FactoryException;
import openwfe.org.ApplicationContext;
import openwfe.org.engine.participants.Participant;
import openwfe.org.engine.participants.ParticipantMapFactory;
import openwfe.org.engine.participants.AbstractParticipantMap;
/**
* A participant map implementation using one or more ParticipantMapFactory
* instances as sources.
*
* CVS Info :
*
$Author$
*
$Id$
*
* @author [email protected]
*/
public class CompositeParticipantMap
extends AbstractParticipantMap
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(CompositeParticipantMap.class.getName());
//
// CONSTANTS & co
/**
* A composite participant map enumerates its participants from
* ParticipantMapFactory instances; it should have at least one
* configured.
*/
public final static String P_FACTORY
= "factory";
//
// FIELDS
private java.util.List factoryNames = null;
//
// CONSTRUCTORS
public void init
(final String serviceName,
final ApplicationContext context,
final java.util.Map serviceParams)
throws
ServiceException
{
super.init(serviceName, context, serviceParams);
//
// enumerate factories
this.factoryNames = new java.util.ArrayList(3);
final Object o = serviceParams.get(P_FACTORY);
if (o instanceof java.util.List)
this.factoryNames.addAll((java.util.List)o);
else
this.factoryNames.add(o);
//
// reload (initial load)
reload();
}
//
// METHODS from AbstractParticipantMap
/**
* Will ask to each ParticipantMapFactory used whether its source changed
* if yes, will reload the whole participant map.
*/
protected void refreshMap ()
throws ServiceException
{
boolean shouldRefresh = false;
final java.util.Iterator it = lookupFactories().iterator();
while (it.hasNext())
{
final ParticipantMapFactory factory =
(ParticipantMapFactory)it.next();
try
{
if (factory.hasSourceChanged())
{
shouldRefresh = true;
break;
}
}
catch (final FactoryException e)
{
log.warn
("refreshMap() problem with a ParticipantMapFactory", e);
throw new ServiceException
("problem with a ParticipantMapFactory", e);
}
}
if (shouldRefresh)
{
log.debug
("refreshMap() yes, reloading participants is necessary...");
reload();
}
}
//
// METHODS from ParticipantMap
//
// METHODS
/**
* Returns the list of factories this composite participant map uses;
* only factories service names are kept in the list, not factory
* instances directly.
*/
public java.util.List getFactoryNames ()
{
return this.factoryNames;
}
//
// PROTECTED METHODS
/**
* Given a factory name, looks up its instane (service) as stored
* in the composite participant map's application context.
*/
protected ParticipantMapFactory lookupFactory (final String factoryName)
{
return (ParticipantMapFactory)getContext().lookup(factoryName);
}
/**
* Returns a list of ParticipantMapFactory instances this composite
* participant map uses.
*/
protected java.util.List lookupFactories ()
{
final java.util.List l =
new java.util.ArrayList(this.factoryNames.size());
final java.util.Iterator it = this.factoryNames.iterator();
while (it.hasNext())
{
final String factoryName = (String)it.next();
final ParticipantMapFactory factory = lookupFactory(factoryName);
if (factory == null)
{
log.warn
("lookupFactories() "+
"no ParticipantMapFactory named '"+factoryName+"' found.");
continue;
}
l.add(factory);
}
return l;
}
/**
* Reloads the participants from each participant map factory, in order.
*/
protected void reload ()
throws ServiceException
{
log.info("reload()");
this.getParticipants().clear();
final java.util.Iterator it = lookupFactories().iterator();
while (it.hasNext())
{
final ParticipantMapFactory factory =
(ParticipantMapFactory)it.next();
java.util.List participants = null;
try
{
participants = factory.createParticipants(this);
}
catch (final FactoryException e)
{
log.warn
("reload() problem creating participants", e);
throw new ServiceException
("problem creating participants", e);
}
final java.util.Iterator pit = participants.iterator();
while (pit.hasNext())
{
final Participant p = (Participant)pit.next();
this.add(p);
log.info("reload() added participant '"+p.getRegex()+"'");
}
}
}
//
// STATIC METHODS
}