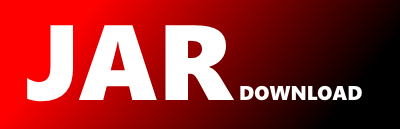
src.openwfe.org.engine.tools.PoolGrapher Maven / Gradle / Ivy
/*
* Copyright (c) 2006, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: Definitions.java,v 1.101.2.1 2006/03/27 22:16:50 jmettraux Exp $
*/
//
// PoolGrapher.java
//
// [email protected]
//
// generated with
// jtmpl 1.1.01 2004/05/19 ([email protected])
//
package openwfe.org.engine.tools;
import openwfe.org.Service;
import openwfe.org.ApplicationContext;
import openwfe.org.time.Time;
import openwfe.org.engine.Definitions;
import openwfe.org.engine.expool.ExpressionStore;
import openwfe.org.engine.expressions.Environment;
import openwfe.org.engine.expressions.FlowExpression;
import openwfe.org.engine.expressions.FlowExpressionId;
import openwfe.org.engine.expressions.DefineExpression;
import openwfe.org.engine.expressions.OneChildExpression;
import openwfe.org.engine.expressions.CompositeFlowExpression;
import openwfe.org.engine.expressions.ParticipantExpression;
import openwfe.org.engine.expressions.state.ExpressionState;
import openwfe.org.engine.impl.expool.XmlExpressionStore;
import openwfe.org.engine.impl.expool.SimpleExpressionPool;
/**
* This tool is meant to list the expressions in a pool and show the links
* between them, the use case being identifying mis-linked expressions.
*
* CVS Info :
*
$Author$
*
$Id$
*
* @author [email protected]
*/
public class PoolGrapher
{
/*
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(PoolGrapher.class.getName());
*/
//
// CONSTANTS & co
private static ApplicationContext applicationContext =
new ApplicationContext();
private static java.util.Map pool =
new java.util.HashMap();
private static boolean printOwfeVersion = false;
//
// STATIC METHODS
private static ExpressionStore getExpressionStore
(final String storeClassName, final java.util.Map storeParams)
throws
Exception
{
final Class storeClass = Class.forName(storeClassName);
final ExpressionStore store = (ExpressionStore)storeClass.newInstance();
if (storeClass.equals(XmlExpressionStore.class) &&
storeParams.size() < 1)
{
storeParams.put
(XmlExpressionStore.P_WORK_DIRECTORY, "./work/engine/pool");
}
((Service)store).init
("expressionStore", applicationContext, storeParams);
return store;
}
private static void printUsage (final String cmd)
{
System.out.println();
System.out.println("Usage :");
System.out.println();
System.out.println(" "+cmd+" [-s storeClassName] {-paramName paramValue}* {wfid}*");
System.out.println();
System.out.println(" will print out a list of the expression in the pool an how they are linked");
System.out.println();
System.out.println(" -s storeClassName");
System.out.println(" sets the store class to use (by default, it's XmlExpressionStore");
System.out.println(" -V");
System.out.println(" prints the OpenWFE version for each expression");
System.out.println(" -paramName paramValue");
System.out.println(" passes parameters to the store");
System.out.println();
System.out.println(" -h");
System.out.println(" prints this usage and exits");
System.out.println();
System.out.println(" ("+Definitions.OPENWFE_VERSION+")");
System.out.println();
System.exit(-1);
}
private static void putExpression
(final String wfids, final FlowExpression fe)
{
final Long wfid = new Long(fe.getId().getParentWorkflowInstanceId());
final String sWfid = ""+wfid;
if (wfids != null &&
wfids.indexOf(sWfid) < 0)
{
return;
}
java.util.Map flowInstance = (java.util.Map)pool.get(wfid);
if (flowInstance == null)
{
flowInstance = new java.util.HashMap();
pool.put(wfid, flowInstance);
}
flowInstance.put(fe.getId(), fe);
}
/*
private static FlowExpression fetchExpression (final FlowExpressionId fei)
{
final Long wfid = new Long(fei.getParentWorkflowInstanceId());
java.util.Map flowInstance = (java.util.Map)pool.get(wfid);
if (flowInstance == null) return null;
return (FlowExpression)flowInstance.get(fei);
}
*/
private static void renderPool ()
{
System.out.println("digraph OpenWFE_expression_pool {");
//System.out.println(" rankdir=TB;");
System.out.println(" rankdir=LR;");
//System.out.println(" size=\"8,11\";");
//System.out.println(" node [shape = circle];");
System.out.println(" node [shape = ellipse, color = gray85 ];");
//System.out.println(" node [shape = ellipse, style = \"dotted\" ];");
final java.util.Iterator it = pool.keySet().iterator();
while (it.hasNext())
{
final java.util.Map flowInstance =
(java.util.Map)pool.get(it.next());
renderFlowInstance(flowInstance);
}
System.out.println("}");
}
private static void renderFlowInstance (final java.util.Map flowInstance)
{
final java.util.Map m = new java.util.HashMap();
java.util.Iterator it = flowInstance.keySet().iterator();
while (it.hasNext())
{
final FlowExpressionId fei = (FlowExpressionId)it.next();
//renderExpression((FlowExpression)flowInstance.get(fei));
final String sortKey =
fei.getWorkflowInstanceId()+"_"+fei.getExpressionId();
m.put(sortKey, fei);
}
final java.util.List l = new java.util.ArrayList(m.keySet());
java.util.Collections.sort(l);
it = l.iterator();
while (it.hasNext())
{
final String sortKey = (String)it.next();
final FlowExpressionId fei = (FlowExpressionId)m.get(sortKey);
renderExpression((FlowExpression)flowInstance.get(fei));
}
}
private static String id (final FlowExpressionId fei)
{
final StringBuffer sb = new StringBuffer();
sb
.append(fei.getWorkflowInstanceId())
.append("_")
.append(fei.getExpressionName())
.append("_")
.append(fei.getExpressionId());
sb
.append("\\n");
sb
.append(fei.getWorkflowDefinitionName())
.append(" ")
.append(fei.getWorkflowDefinitionRevision());
if (printOwfeVersion)
{
sb
.append(" (")
.append(fei.getOwfeVersion())
.append(")");
}
return sb.toString();
}
private static void printLink
(final FlowExpressionId from,
final FlowExpressionId to,
final String label)
{
if (from == null || to == null) return;
System.out.print(" ");
System.out.print("\"");
System.out.print(id(from));
System.out.print("\"");
System.out.print(" -> ");
System.out.print("\"");
System.out.print(id(to));
System.out.print("\"");
if (label.equals("env"))
System.out.print(" [ constraint = false ];");
else
System.out.print(" [ label = \""+label+"\" ];");
System.out.println();
}
private static void printLabel
(final FlowExpression fe)
{
final StringBuffer sb = new StringBuffer();
sb.append(id(fe.getId()));
// output class
sb
.append("\\n")
.append(fe.getClass().getName());
// output attributes
final java.util.List atts =
new java.util.ArrayList(fe.getAttributes().keySet());
java.util.Collections.sort(atts);
final java.util.Iterator it = atts.iterator();
while (it.hasNext())
{
final String key = (String)it.next();
final String value = (String)fe.getAttributes().get(key);
sb
.append("\\n")
.append(key)
.append(" = ")
.append("'")
.append(value)
.append("'");
}
// participant output
boolean applied = false;
if (fe instanceof ParticipantExpression)
{
final ParticipantExpression pe = (ParticipantExpression)fe;
if (pe.getAppliedWorkitem() != null)
{
applied = true;
sb
.append("\\n")
.append("*applied*");
}
}
// output state
if (fe.getState() != null)
{
final String since = Time.toIsoDate(fe.getState().getSince());
sb
.append("\\n")
.append(fe.getState().getName())
.append(" since ")
.append(since);
}
System.out.print(" ");
System.out.print("\"");
System.out.print(id(fe.getId()));
System.out.print("\"");
System.out.print(" [ label = \""+sb.toString()+"\"");
if (fe instanceof Environment)
System.out.print(" shape = rect ");
if (applied)
System.out.print(" color = red ");
else
System.out.print(" color = black ");
System.out.print("];");
System.out.println();
}
private static void renderExpression (final FlowExpression fe)
{
final ExpressionState state = SimpleExpressionPool.getState(fe);
final String since = Time.toIsoDate(state.getSince());
final FlowExpressionId fei = fe.getId();
printLabel(fe);
printLink(fei, fe.getParent(), "parent");
//printLink(fei, fe.getEnvironmentId(), "env");
if (Environment.ownsEnvironment(fe))
printLink(fei, fe.getEnvironmentId(), "env");
if (fe instanceof CompositeFlowExpression)
{
final java.util.Iterator it =
((CompositeFlowExpression)fe).getChildren().iterator();
//final StringBuffer sbRank = new StringBuffer();
//sbRank.append(" {rank = same;");
while (it.hasNext())
{
final FlowExpressionId childId = (FlowExpressionId)it.next();
printLink(fei, childId, "child");
//sbRank
// .append("\"")
// .append(id(childId))
// .append("\";");
}
//sbRank.append("}");
//System.out.println(sbRank.toString());
}
else if (fe instanceof OneChildExpression)
{
final FlowExpressionId childId =
((OneChildExpression)fe).getChildExpressionId();
printLink(fei, childId, "child");
}
}
/*
private static void renderPool ()
{
final java.util.Iterator it = pool.keySet().iterator();
while (it.hasNext())
{
final java.util.Map flowInstance =
(java.util.Map)pool.get(it.next());
renderFlowInstance(flowInstance);
}
}
private static void renderFlowInstance (final java.util.Map flowInstance)
{
final java.util.Iterator it = flowInstance.keySet().iterator();
while (it.hasNext())
{
final FlowExpressionId fei = (FlowExpressionId)it.next();
renderExpression((FlowExpression)flowInstance.get(fei));
}
}
private static void renderExpression (final FlowExpression fe)
{
final ExpressionState state = SimpleExpressionPool.getState(fe);
final String since = Time.toIsoDate(state.getSince());
System.out.println();
System.out.println(" +-- parent "+renderLink(fe.getParent()));
//System.out.println(" |");
System.out.println(" V");
System.out.println(" * "+fe.getId());
System.out.println(" | (state '"+state.getName()+"' since "+since+")");
System.out.println(" +--- env "+renderLink(fe.getEnvironmentId()));
System.out.println(" +--- behalf "+renderLink(fe.getBehalf()));
System.out.println(" +--> next "+renderLink(fe.getNext()));
System.out.println(" <-- previous "+renderLink(fe.getPrevious()));
if (fe instanceof CompositeFlowExpression)
{
final java.util.Iterator it =
((CompositeFlowExpression)fe).getChildren().iterator();
while (it.hasNext())
renderChild((FlowExpressionId)it.next());
}
else if (fe instanceof OneChildExpression)
{
final FlowExpressionId childId =
((OneChildExpression)fe).getChildExpressionId();
renderChild(childId);
}
}
private static void renderChild (final FlowExpressionId fei)
{
System.out.println(" o child "+renderLink(fei));
}
private static String renderLink (final FlowExpressionId fei)
{
if (fei == null) return "";
String s = fei.toString();
if (fetchExpression(fei) == null) s += " // MISSING //";
return s;
}
*/
/**
* The main method.
*/
public static void main (final String[] args)
throws Exception
{
String cmd = "java "+PoolGrapher.class.getName();
int index = 0;
// extract $0
if (args.length < 1) printUsage(cmd);
if ( ! args[0].startsWith("-"))
{
cmd = args[0];
index = 1;
}
// extract $*
//boolean verbose = false;
String storeClassName = XmlExpressionStore.class.getName();
final java.util.Map storeParams = new java.util.HashMap();
while (index < args.length &&
args[index].startsWith("-"))
//
// gather options
{
final String arg = args[index];
if (arg.equals("-h"))
{
printUsage(cmd);
}
if (arg.equals("-s"))
{
storeClassName = args[index+1];
}
else if (arg.equals("-V"))
{
printOwfeVersion = true;
}
else
{
storeParams.put(args[index].substring(1), args[index+1]);
}
index += 2;
}
String wfids = null;
while (index < args.length)
{
if (wfids == null) wfids = "";
wfids += args[index];
wfids += " ";
index++;
}
//
// do the job
applicationContext.setApplicationName
("tool:"+PoolGrapher.class.getName());
// set up expression store
final ExpressionStore es =
getExpressionStore(storeClassName, storeParams);
// iterate to load pool
final java.util.Iterator it = es.contentIterator(null);
while (it.hasNext())
{
final FlowExpression fe = (FlowExpression)it.next();
putExpression(wfids, fe);
}
renderPool();
}
}