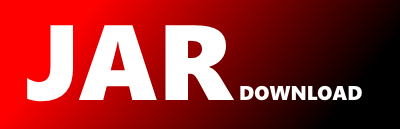
src.openwfe.org.engine.workitem.AttributeUtils Maven / Gradle / Ivy
/*
* Copyright (c) 2001-2006, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: AttributeUtils.java 2829 2006-06-15 16:46:52Z jmettraux $
*/
//
// AttributeUtils.java
//
// [email protected]
//
// generated with
// jtmpl 1.1.00 16.08.2003 John Mettraux ([email protected])
//
package openwfe.org.engine.workitem;
import java.lang.reflect.Array;
import java.lang.reflect.Method;
import openwfe.org.ReflectionUtils;
import openwfe.org.ApplicationContext;
import openwfe.org.engine.expressions.FlowExpressionId;
/**
* Some methods for turning a MapAttribute into a Map and 'vice versa'.
*
* Following an idea of Richard Jennings
*
* CVS Info :
*
$Author: jmettraux $
*
$Id: AttributeUtils.java 2829 2006-06-15 16:46:52Z jmettraux $
*
* @author [email protected]
*/
public abstract class AttributeUtils
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(AttributeUtils.class.getName());
//
// CONSTANTS
/*
* If this bean is present in a StringMapAttribute, it means that this
* map is a Bean
*/
private final static String A_BEAN_CLASSNAME
= "__bean_classname__";
/**
* When a FlowExpressionId (it implements Attribute) is passing through
* owfe2java, the result is a string which begins with this prefix.
* "fei::"
*/
public final static String FEI_PREFIX = "fei::";
//
// OWFE TO JAVA
/**
* Turns an OpenWFE attribute into a java instance
*/
public static Object owfe2java (final Attribute a)
{
if (a instanceof AtomicAttribute)
return ((AtomicAttribute)a).getValue();
if (a instanceof StringMapAttribute)
{
final StringMapAttribute sma = (StringMapAttribute)a;
final Attribute va = sma.get(A_BEAN_CLASSNAME);
if ((va != null) && (va instanceof StringAttribute))
return smap2bean(sma);
}
if (a instanceof MapAttribute)
return map2java((MapAttribute)a);
if (a instanceof ListAttribute)
return list2java((ListAttribute)a);
if (a instanceof FlowExpressionId)
return FEI_PREFIX + ((FlowExpressionId)a).toParseableString();
return "null";
}
/*
* Turns a string map attribute with the '__bean_classname__' attribute
* set into a bean.
*/
private static Object smap2bean (final StringMapAttribute sma)
{
final String beanClassName = sma.sget(A_BEAN_CLASSNAME);
final Object bean = ReflectionUtils.buildNewInstance(beanClassName);
final java.util.Map fields = map2java(sma);
final java.util.Iterator it = fields.keySet().iterator();
while (it.hasNext())
{
final String fieldName = (String)it.next();
if (log.isDebugEnabled())
log.debug("smap2bean() fieldName >"+fieldName+"<");
if (fieldName.equals(A_BEAN_CLASSNAME)) continue;
try
{
final Class fieldClass = ReflectionUtils
.getAttributeClass(bean.getClass(), fieldName);
if (log.isDebugEnabled())
{
log.debug
("smap2bean() "+
"fieldClass is >"+fieldClass.getName()+"<");
}
Object fieldValue = null;
if (fieldClass.isArray())
{
if (log.isDebugEnabled())
log.debug("smap2bean() '"+fieldName+"' is an array.");
fieldValue = toArray
(fieldClass, (java.util.List)fields.get(fieldName));
}
else
{
fieldValue = fields.get(fieldName);
}
ReflectionUtils.setAttribute(bean, fieldName, fieldValue);
}
catch (final Exception e)
{
log.warn
("smap2bean() bean of class '"+beanClassName+
"' : failed to set value for field '"+fieldName+
"'. Skipped field.");
log.warn("because of", e);
}
}
return bean;
}
/*
* Turns a list into an array
*/
private static Object toArray
(final Class arrayClass, final java.util.List l)
throws
ClassNotFoundException
{
final Class itemClass = ReflectionUtils.getItemClass(arrayClass);
if (log.isDebugEnabled())
log.debug("toArray() itemClass is "+itemClass.getName());
Object result = Array.newInstance(itemClass, l.size());
for (int i=0; i