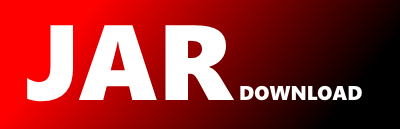
src.openwfe.org.engine.workitem.StringMapAttribute Maven / Gradle / Ivy
/*
* Copyright (c) 2001-2006, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: StringMapAttribute.java 2854 2006-06-22 20:37:30Z jmettraux $
*/
//
// StringMapAttribute.java
//
// [email protected]
//
// generated with
// jtmpl 1.0.04 31.10.2002 John Mettraux ([email protected])
//
package openwfe.org.engine.workitem;
/**
* A dictionary of attributes whose key must be StringAttribute instances.
* Important thing : each InFlowWorkitem has as root attribute an instance of
* StringMapAttribute.
* This class extends MapAttribute. It introduces a lot of shortcut methods for
* working with strings.
* You should also see 'AttributeUtils' in the same package to find method
* helping you to work with attributes.
*
* CVS Info :
*
$Author: jmettraux $
*
$Date: 2006-06-22 22:37:30 +0200 (Thu, 22 Jun 2006) $
*
$Id: StringMapAttribute.java 2854 2006-06-22 20:37:30Z jmettraux $
*
* @author [email protected]
*/
public class StringMapAttribute
extends MapAttribute
{
static final long serialVersionUID = -297013353044083805L;
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(StringMapAttribute.class.getName());
//
// FIELDS
//
// CONSTRUCTORS
/**
* Builds an empty map
*/
public StringMapAttribute ()
{
super();
}
/**
* Builds an empty map with a given initial capacity (if you know
* the capacity in advance, use this constructor, it may save you
* the performance hit induced by the map growing to a bigger capacity).
*/
public StringMapAttribute (int initialCapacity)
{
super(initialCapacity);
}
//
// METHODS
/**
* Returns the attribute corresponding to the given String key.
*/
public Attribute get (final String key)
{
return (Attribute)getMap().get(new StringAttribute(key));
}
/**
* Like get() but returns the attribute value as a String.
*/
public String sget (final String key)
{
final Attribute a = get(key);
if (a == null) return null;
return a.toString();
}
/**
* Puts in the map a new entry. A previous entry with the same key name
* will get overriden.
*/
public void put (final String key, final Attribute value)
{
getMap().put(new StringAttribute(key), value);
}
/**
* Puts an entry composed of two strings (a StringAttribute will also
* be created for the value).
*/
public void puts (final String key, final String value)
{
getMap().put(new StringAttribute(key), new StringAttribute(value));
}
/**
* Removes a given entry.
*/
public void remove (final String key)
{
log.debug("remove() key '"+key+"'");
getMap().remove(new StringAttribute(key));
}
/**
* Returns true if this StringMapAttribute instance contains the
* given key.
*/
public boolean hasKey (final String key)
{
return (this.get(key) != null);
}
/**
* Returns the set of key names (String instances) of this map that
* match the given regex.
*/
public java.util.Set keyNamesMatching (final String regex)
{
final java.util.Set result = new java.util.HashSet();
final java.util.Iterator it = this.keySet().iterator();
while (it.hasNext())
{
final String key = it.next().toString();
if (key.matches(regex)) result.add(key);
}
return result;
}
/**
* This returns a set of String keys (not StringAttribute keys)
*/
public java.util.Set stringKeySet ()
{
java.util.Set initialSet = super.keySet();
java.util.Set set = new java.util.HashSet();
java.util.Iterator it = initialSet.iterator();
while (it.hasNext())
{
set.add(it.next().toString());
}
return set;
}
/**
* Returns an alphabetically sorted iterator on the keys of this
* StringMapAttribute.
* The keys returned are String instances, not StringAttribute ones.
* It's then easy to get the value attributes with
*
* Attribute a = smap.get(key);
*
*/
public java.util.Iterator alphaStringIterator ()
{
final java.util.List keyList =
new java.util.LinkedList(this.stringKeySet());
java.util.Collections.sort(keyList);
return keyList.iterator();
}
/**
* This is a particular iterator : it iterates on the value of a field
* given by its name.
* For instance, if in this StringMapAttribute you have a field named
* 'toto' that points to a StringAttribute containing "foo, bar",
* this method will return an iterator on [ "foo", "bar" ].
* Similarly, if 'toto' would point to a ListAttribute containing
* [ new StringAttribute("foo2"), new StringAttribute("bar2") ], it
* would return an iterator on [ "foo2", "bar2" ].
* If the fieldName points to nothing, null is returned.
*/
public java.util.Iterator fieldIterator (final String fieldName)
{
final Attribute a = this.get(fieldName);
if (a == null) return null;
if (a instanceof ListAttribute)
{
final java.util.List l = AttributeUtils.list2java((ListAttribute)a);
return l.iterator();
}
final String[] ss = a.toString().trim().split(", *");
return java.util.Arrays.asList(ss).iterator();
}
/**
* The counterpart of setField().
* It's actually a call to
* CollectionAttribute.lookupAttribute(fieldName, this);
*/
public Attribute getField (final String fieldName)
{
return CollectionAttribute.lookupAttribute(fieldName, this);
}
/**
* Removes a field (just a shortcut for setField(fieldName, null)).
*/
public void unsetField (final String fieldName)
{
setField(fieldName, null);
}
/**
* setField() accepts fieldNames that contain a path, like
* "customer.name.firstname". For the rest, it behaves like a puts().
*/
public void setField
(final String fieldName,
final Object value)
{
//log.debug("setField() fieldName is >"+fieldName+"<");
Attribute aValue = null;
if (value != null)
{
if (value instanceof Attribute)
aValue = (Attribute)value;
else
aValue = new StringAttribute(value.toString());
// wouldn't it be better to use AttributeUtils.java2owfe() here ?
}
final int idot = fieldName.lastIndexOf(".");
if (idot < 0)
{
//log.debug("setField() local effect");
if (aValue == null)
this.remove(fieldName);
else
this.put(fieldName, aValue);
return;
}
final CollectionAttribute container =
CollectionAttribute.lookupContainer(fieldName, this);
if (container == null)
{
if (log.isDebugEnabled())
{
log.debug
("setField() could not follow path >"+fieldName+
"<. Did not set or unset anything.");
}
return;
}
final String key = fieldName.substring(idot+1);
//log.debug("setField() local key >"+key+"<");
container.cput(key, aValue);
}
}