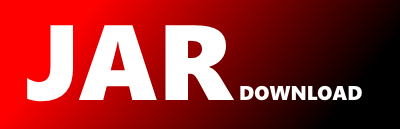
src.openwfe.org.engine.control.rest.RestControlSession Maven / Gradle / Ivy
/*
* Copyright (c) 2005, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: RestControlSession.java 2414 2006-03-10 22:15:03Z jmettraux $
*/
//
// RestControlSession.java
//
// [email protected]
//
// generated with
// jtmpl 1.1.01 2004/05/19 ([email protected])
//
package openwfe.org.engine.control.rest;
import java.nio.channels.SelectionKey;
import openwfe.org.ServiceException;
import openwfe.org.time.Time;
import openwfe.org.rest.RestService;
import openwfe.org.rest.RmiBridgedRestSession;
import openwfe.org.engine.Definitions;
import openwfe.org.engine.control.ControlSession;
import openwfe.org.engine.workitem.WorkItemCoderLoader;
import openwfe.org.engine.expressions.FlowExpression;
import openwfe.org.engine.expressions.FlowExpressionId;
import openwfe.org.engine.impl.workitem.xml.XmlWorkItemCoder;
/**
* Accessing the control interface of an engine through REST
*
* CVS Info :
*
$Author: jmettraux $
*
$Id: RestControlSession.java 2414 2006-03-10 22:15:03Z jmettraux $
*
* @author [email protected]
*/
public class RestControlSession
extends RmiBridgedRestSession
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(RestControlSession.class.getName());
//
// CONSTANTS & co
//
// FIELDS
private ControlSession session = null;
private XmlWorkItemCoder coder = null;
//
// CONSTRUCTORS
public void init
(final RestService service,
final Long sessionId,
final String username,
final String password)
throws
ServiceException
{
super.init(service, sessionId, username, password);
//
// locating the coder that will be used
final WorkItemCoderLoader loader = Definitions
.getWorkItemCoderLoader(this.getService().getContext());
this.coder = (XmlWorkItemCoder)loader.getXmlCoder();
log.info
("WorkItemCoder successfully located");
}
//
// METHODS from RestSession
protected boolean authentify
(final String username, final String password)
throws
ServiceException
{
try
{
this.session = (ControlSession)getWorkSessionServer()
.login(username, password);
}
catch (final Exception e)
{
throw new ServiceException("login failed", e);
}
return true;
}
//
// METHODS
protected org.jdom.Element encode (final FlowExpression fe)
{
final org.jdom.Element eExpression = new org.jdom.Element("expression");
if (fe.getApplyTime() != null)
eExpression.setAttribute("apply-time", fe.getApplyTime());
if (fe.getState() != null)
{
eExpression.setAttribute
("state", fe.getState().getName());
eExpression.setAttribute
("state-since", Time.toIsoDate(fe.getState().getSince()));
}
eExpression.addContent(this.coder.encode(fe.getId()));
return eExpression;
}
//
// METHODS for REST actions
protected FlowExpressionId parseFei
(final SelectionKey key,
final String[] headers)
throws
Exception
{
return this.coder.decodeFlowExpressionId(parseBody(key, headers));
}
public void do_listexpressions
(final SelectionKey key,
final String[] headers)
throws
Exception
{
final java.util.List expressions = this.session.listExpressions();
org.jdom.Element eExpressions = new org.jdom.Element("expressions");
final java.util.Iterator it = expressions.iterator();
while (it.hasNext())
{
final FlowExpression fe = (FlowExpression)it.next();
eExpressions.addContent(encode(fe));
}
reply(key, eExpressions);
}
public void do_cancelexpression
(final SelectionKey key,
final String[] headers)
throws
Exception
{
this.session.cancelExpression(parseFei(key, headers));
reply(key, new org.jdom.Element("ok"));
}
public void do_freezeexpression
(final SelectionKey key,
final String[] headers)
throws
Exception
{
this.session.freezeExpression(parseFei(key, headers));
reply(key, new org.jdom.Element("ok"));
}
public void do_unfreezeexpression
(final SelectionKey key,
final String[] headers)
throws
Exception
{
this.session.unfreezeExpression(parseFei(key, headers));
reply(key, new org.jdom.Element("ok"));
}
//
// STATIC METHODS
}