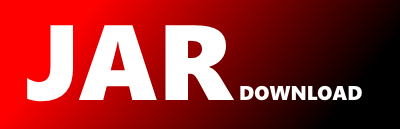
src.openwfe.org.engine.control.shell.ControlShell Maven / Gradle / Ivy
/*
* Copyright (c) 2005, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: ControlShell.java 1882 2005-05-17 16:41:07Z jmettraux $
*/
package openwfe.org.engine.control.shell;
import java.rmi.Naming;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import openwfe.org.rmi.session.WorkSessionServer;
import openwfe.org.shell.CmdHandler;
import openwfe.org.engine.control.ControlSession;
import openwfe.org.engine.expressions.FlowExpression;
import openwfe.org.engine.expressions.FlowExpressionId;
//import openwfe.org.engine.expressions.FreezableExpression;
/**
* An RMI shell for controlling flows in an engine
*
* CVS Info :
*
$Author: jmettraux $
*
$Date: 2005-05-17 18:41:07 +0200 (Tue, 17 May 2005) $
*
$Id: ControlShell.java 1882 2005-05-17 16:41:07Z jmettraux $
*
* @author [email protected]
* @author [email protected]
*/
public class ControlShell
{
public final static String BANNER = "\n" + ControlShell.class.getName() + "\n$Revision: 1882 $ John Mettraux, Jay Lawrence\n";
//
// FIELDS
private String url;
private ControlSession session;
private String prompt;
private String language = null;
private BufferedReader reader;
private java.util.List lastExpressionList = null;
//
// CONSTRUCTOR
public ControlShell
(final String url,
final ControlSession session,
final BufferedReader reader)
{
this.url = url;
this.session = session;
prompt = url + "> ";
this.reader = reader;
}
//
// help methods
private String toString (final FlowExpression fe)
{
final boolean isUnapplied = (fe.getApplyTime() == null);
final StringBuffer sb = new StringBuffer();
if (fe.getState() != null)
sb.append(fe.getState().getIdentifier());
else
sb.append("-");
if (isUnapplied)
sb.append("u");
else
sb.append("-");
sb.append(" ");
sb.append(fe.getId().getWorkflowInstanceId());
sb.append(" ");
sb.append(fe.getId().getWorkflowDefinitionName());
sb.append(" ");
sb.append(fe.getId().getWorkflowDefinitionRevision());
sb.append(" ");
sb.append(fe.getId().getExpressionName());
sb.append(" ");
sb.append(fe.getId().getExpressionId());
return sb.toString();
}
//
// an expressionComparator by Jay Lawrence
static final java.util.Comparator expressionComparator =
new java.util.Comparator()
{
public int compare( Object fe1, Object fe2 )
{
final FlowExpressionId feId1 = ((FlowExpression)fe1).getId();
final FlowExpressionId feId2 = ((FlowExpression)fe2).getId();
return
feId1.getWorkflowInstanceId()
.compareTo(feId2.getWorkflowInstanceId());
}
};
private void displayExpressionList
(final String title, final java.util.List list)
{
System.out.println(title);
java.util.Collections.sort(list, expressionComparator);
this.lastExpressionList = list;
if (list == null)
{
System.out.println(" X no results");
return;
}
int i = 0;
final java.util.Iterator it = list.iterator();
while (it.hasNext())
{
final FlowExpression fe = (FlowExpression)it.next();
System.out.println(" - "+i+" "+toString(fe));
i++;
}
}
private FlowExpressionId getExpression (final String sExpressionId)
{
if (this.lastExpressionList == null)
{
throw new IllegalArgumentException
("No exceptions were 'list'ed before");
}
int index = -1;
try
{
index = Integer.parseInt(sExpressionId);
}
catch (NumberFormatException nfe)
{
throw new IllegalArgumentException
("'"+sExpressionId+"' is not a number");
}
if (index < 0 || index-1 > this.lastExpressionList.size())
{
throw new IllegalArgumentException
("No expression at index "+index);
}
return ((FlowExpression)this.lastExpressionList.get(index)).getId();
}
//
// Commands and their implementation
public void help_list ()
{
System.out.println("list");
System.out.println(" lists both frozen and unapplied expressions");
}
public Boolean do_list (final String[] args)
{
try
{
displayExpressionList
("expressions :", this.session.listExpressions());
return Boolean.FALSE;
}
catch (Exception e)
{
e.printStackTrace();
return Boolean.FALSE;
}
}
public void help_unfreeze ()
{
System.out.println("unfreezex ");
System.out.println(" defreeze an expression, the engine will immediately try to apply it");
}
public Boolean do_unfreeze (final String[] args)
{
if (args.length < 1)
{
help_unfreeze();
return Boolean.FALSE;
}
try
{
this.session.unfreezeExpression(getExpression(args[0]));
}
catch (Throwable t)
{
t.printStackTrace();
}
return Boolean.FALSE;
}
public void help_freeze ()
{
System.out.println("freezex ");
System.out.println(" freezes an expression, the engine will immediately try to apply it");
}
public Boolean do_freeze (final String[] args)
{
if (args.length < 1)
{
help_freeze();
return Boolean.FALSE;
}
try
{
this.session.freezeExpression(getExpression(args[0]));
}
catch (Throwable t)
{
t.printStackTrace();
}
return Boolean.FALSE;
}
public void help_cancel ()
{
System.out.println("cancel ");
System.out.println(" cancels an expression (and all its children)");
System.out.println(" and replies to its parent expression.");
}
public Boolean do_cancel (final String[] args)
{
if (args.length < 1)
{
help_cancel();
return Boolean.FALSE;
}
try
{
this.session.cancelExpression(getExpression(args[0]));
}
catch (Throwable t)
{
t.printStackTrace();
}
return Boolean.FALSE;
}
public Boolean do_quit (final String[] args)
{
return CmdHandler.DO_EXIT;
}
public Boolean do_exit (final String[] args)
{
return CmdHandler.DO_EXIT;
}
//
// main stuff
public void run ()
{
final CmdHandler parser = new CmdHandler(this);
parser.commandLoop(prompt, reader);
System.out.println("Bye.");
System.exit(0);
}
public static void main (String[] args)
{
final String DEFAULT_URL = "rmi://localhost:7089/controlSessionServer";
System.out.println(BANNER);
if (args.length > 0)
{
String arg = args[0].trim().toLowerCase();
if (arg.equals("-help") ||
arg.equals("--help") ||
arg.equals("-h") ||
arg.equals("-?"))
{
System.out.println("OpenWFE engine control client");
System.out.println();
System.out.println("Usage: " + ControlShell.class.getName() + " [ [ []]");
System.out.println();
System.out.println("Default URL is " + DEFAULT_URL);
System.exit(0);
}
}
try
{
String url = args.length > 0 ? args[0] : DEFAULT_URL;
BufferedReader reader = new BufferedReader
(new InputStreamReader(System.in));
//
// Get the username/password if not specified on the command line.
String username;
if (args.length > 1)
{
username = args[1];
}
else
{
System.out.print("username> ");
username = reader.readLine();
}
String password;
if (args.length > 2)
{
password = args[2];
}
else
{
System.out.print("password> ");
password = reader.readLine();
}
//
// Login to the session server.
WorkSessionServer sessionServer =
(WorkSessionServer)Naming.lookup(url);
ControlSession session =
(ControlSession)sessionServer.login(username, password);
new ControlShell(url, session, reader).run();
}
catch (Exception e)
{
e.printStackTrace();
}
}
}