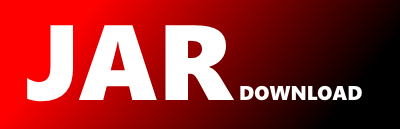
src.openwfe.org.engine.expool.ExpressionPool Maven / Gradle / Ivy
/*
* Copyright (c) 2001-2006, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: ExpressionPool.java 2846 2006-06-20 21:58:03Z jmettraux $
*/
//
// ExpressionPool.java
//
// [email protected]
//
// generated with
// jtmpl 1.0.04 20.11.2001 John Mettraux ([email protected])
//
package openwfe.org.engine.expool;
import openwfe.org.time.Time;
import openwfe.org.time.Scheduler;
import openwfe.org.time.Schedulable;
import openwfe.org.engine.workitem.InFlowWorkItem;
import openwfe.org.engine.expressions.Environment;
import openwfe.org.engine.expressions.FlowExpression;
import openwfe.org.engine.expressions.ApplyException;
import openwfe.org.engine.expressions.ReplyException;
import openwfe.org.engine.expressions.FlowExpressionId;
/**
* The 'expression pool' is at the heart of the OpenWFE process engine.
* It's litterally a pool of expressions that are pieces of live workflow
* instances.
* An expression pool is backed up by an 'expression store' for storage
* of the expression. Caching and persistence work is done by the store.
*
* CVS Info :
*
$Author: jmettraux $
*
$Date: 2006-06-20 23:58:03 +0200 (Tue, 20 Jun 2006) $
*
$Id: ExpressionPool.java 2846 2006-06-20 21:58:03Z jmettraux $
*
* @author [email protected]
*/
public interface ExpressionPool
extends Schedulable
{
//
// CONSTANTS
/**
* With the '{@value}' parameter, you can set after how much
* time an expression with a timeout may, er well, time out.
* ParticipantExpression is such an expression.
*/
public final static String P_EXPRESSION_TIMEOUT
= "expressionTimeout";
/**
* The default value for an expression timeout ({@value}).
*/
public final static Long DEFAULT_EXPRESSION_TIMEOUT
= new Long(Time.parseTimeString("7d")); // 7 days before timeout occurs
/**
* '{@value}' : how frequently should the purge daemon of the
* engine's expression pool wake up and check for expressions that could
* time out ?
* Set this here with a time value (like "4h2m30s")
*/
public final static String P_PURGE_FREQUENCY
= "purgeFrequency";
/**
* When 'purgeFrequency' is not set, the purge daemon will be launched
* every {@value}.
*/
public final static String DEFAULT_PURGE_FREQUENCY
= "4h"; // 4 hours
/**
* 'when' expressions' validity are checked at regular interval, use this
* '{@value}' parameter name to tune your expression pool as you
* wish.
*/
public final static String P_WHEN_FREQUENCY
= "whenFrequency";
/**
* By default, the daemon in charge of the 'when' expressions will check
* every '{@value}' (two minutes) for WhenExpression validity.
*/
public final static String DEFAULT_WHEN_FREQUENCY
= "2m";
/**
* If this param '{@value}' is set to false, the expression pool
* will not attempt to reload schedulable expressions to reschedule
* them.
* This is especially useful for an InMemoryExpressionPool.
*/
public final static String P_RESCHEDULE
= "reschedule";
/**
* By default, this param '{@value}' is set to true; when set to
* false, launchSub() calls will not return immediately, which is useful
* for frameworks like 'webflow'.
* Else the 'true' setting is the way to go.
*/
public final static String P_ASYNC_LAUNCH
= "asyncLaunch";
//
// METHODS
/**
* Returns the default expression timeout for this expression pool.
*/
public Long getExpressionTimeOut ();
/**
* Usually called by WorkflowInstanceBuilders to add a freshly created
* expression to the pool.
*/
public void add (FlowExpression fe)
throws PoolException;
/**
* Stores the flow expression (as it may have changed).
*/
public void update (FlowExpression fe)
throws PoolException;
/**
* Retrieves an expression from the pool.
* If the expression cannot be retrieved from the pool, null will be
* returned.
*/
public FlowExpression fetch (FlowExpressionId fei);
/**
* Fetches the root environement tied to the engine.
*/
public Environment fetchEngineEnvironment ();
/**
* Returns the environment at the root of a flow (the flow containing
* the requesting expression).
*/
public Environment fetchRootEnvironment (FlowExpression requester);
/**
* Given an expression id, returns the environment id of the
* flow expression.
*/
public FlowExpressionId getEnvironmentId (FlowExpressionId fei);
/**
* given an id, returns the root expression of the flow
*/
public FlowExpression fetchRootOfFlow (FlowExpressionId fei);
/**
* Applies an expression.
* This method is usually called by expressions on their subexpressions or
* by a launchListener on a rootExpression of a workflow just instantiated.
*/
public void apply
(FlowExpressionId fei, InFlowWorkItem wi)
throws
ApplyException;
/**
* A shortcut to
* apply (flowExpressionId, inFlowWorkItem).
*/
public void apply (FlowExpression fe, InFlowWorkItem wi)
throws ApplyException;
/**
* Applies an expression (calls the launch() method of that expression.
*/
public void launch
(FlowExpressionId fei, InFlowWorkItem wi)
throws
ApplyException;
/**
* A shortcut to
* launch (flowExpressionId, inFlowWorkItem).
*/
public void launch (FlowExpression fe, InFlowWorkItem wi)
throws ApplyException;
/**
* This method replies to the father of the expression passed as parameter
* then releases this expression.
*/
public void replyToParent (FlowExpression fe, InFlowWorkItem wi)
throws ReplyException;
/**
* Replies to an expression.
* This method should only be called by listeners
*/
public void reply (FlowExpressionId fei, InFlowWorkItem wi)
throws ReplyException;
/**
* Releases a flow expression.
* This method is called by the replyToParent method, as the
* expression tree gets diminished.
*/
public void removeExpression (FlowExpression fe);
/**
* Releases a flow expression indicated by its id.
*/
public void removeExpression (FlowExpressionId fei);
/**
* (child cancel) Cancels an expression (simply calls its cancel method and
* removes it from the pool).
* Will return a workitem if the cancelled expression was handling one.
*/
public InFlowWorkItem childCancel
(FlowExpressionId childId)
throws
ApplyException;
/**
* (root cancel) Cancels a whole branch, starting with its top expression
* fei.
*/
public void cancel (FlowExpressionId fei);
/**
* Cancels a whole process instance.
*
* @param fei the FlowExpressionId of any expression belonging to the
* flow that will get cancelled.
*/
public void cancelFlow (FlowExpressionId fei);
/**
* Forgets an expression (it stays in the pool, but its reference to its
* parent expression is replaced by a 'gone-parent' tag, so that its
* execution continues but its results will get forgotten (never reaching
* the parent flow)).
*/
public void forget (FlowExpressionId fei);
public void undo (FlowExpression caller, String doName)
throws ApplyException;
public void redo (FlowExpression caller, String doName)
throws ApplyException;
/**
* Retrieves a given class of expressions. If the parameter is set to
* null, will return all the expressions.
*/
public java.util.Iterator contentIterator (Class assignClass);
/**
* Returns the count of expressions store in this expool.
*/
public int size ();
/**
* Returns an XML representation of the current state of the expression
* pool : the forest (a set of expression trees / workflow instances).
*/
public org.jdom.Document dump ();
/* *
* Given an expression and a the id of one of its child, resolves
* a potential 'behalf' for this child.
* /
public FlowExpressionId resolveBehalf
(final FlowExpression requester, final FlowExpressionId childId);
*/
//
// 'next' and 'previous' stuff
/* *
* Sets the 'next' field of the 'subject' expression.
* /
public void setNext
(FlowExpressionId subject, FlowExpressionId next)
throws
PoolException;
/* *
* Sets the 'previous' field of the 'subject' expression.
* /
public void setPrevious
(FlowExpressionId subject, FlowExpressionId previous)
throws
PoolException;
*/
//
// schedule stuff
/**
* Returns the scheduler that the expression pool uses.
*/
public Scheduler getScheduler ();
/**
* This method is used by Schedulable flow expressions when reregistering
* themselves for a further schedule (for instance, an irresolved 'when'
* will reregister for further evaluation).
*/
public void schedule (FlowExpressionId fei);
/**
* Schedules a (Schedulable) flow expression for a given 'at' point in
* time.
* This is used by the SleepExpression.
*/
public void scheduleAt (long at, FlowExpressionId fei);
//
// CONTROL METHODS
/**
* Returns a list of all expressions in the pool.
*/
public java.util.List listExpressions ();
/**
* Unfreezes an expression.
*/
public void unfreezeExpression (FlowExpressionId freezableExpressionId)
throws PoolException;
/**
* Freezes a flow : freezes all of its freezable expressions.
*/
public void freezeFlow (String workflowInstanceId)
throws PoolException;
/**
* Freezes an expression.
*/
public void freezeExpression (FlowExpressionId freezableExpressionId)
throws PoolException;
//
// VARIABLE METHODS
/**
* Looks up the value of a variable. Will lookup until it reaches the
* engine environment.
*/
public Object lookupVariable
(FlowExpression requester, String variableName);
/**
* Looks up the environment that contains the given variable name.
*/
public Environment lookupContainingEnvironment
(FlowExpression requester, String variableName);
/**
* Looks up the value of a variable, but will only look in the local
* environment, will look in parent environments.
*/
public Object lookupLocalVariable
(FlowExpression requester, String variableName);
/**
* Sets a variable.
* If the variableName is prefixed, this method will take care of
* storing the variable in the appropriate [upper] expression.
*/
public void setVariable
(FlowExpression requester, String variableName, Object value);
}