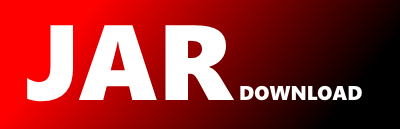
src.openwfe.org.engine.expressions.AbstractCompositeFlowExpression Maven / Gradle / Ivy
/*
* Copyright (c) 2001-2006, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: AbstractCompositeFlowExpression.java 2575 2006-05-08 11:52:39Z jmettraux $
*/
//
// AbstractCompositeFlowExpression.java
//
// [email protected]
//
// generated with
// jtmpl 1.0.04 20.11.2001 John Mettraux ([email protected])
//
package openwfe.org.engine.expressions;
import openwfe.org.ReflectionUtils;
import openwfe.org.ApplicationContext;
import openwfe.org.engine.workitem.InFlowWorkItem;
import openwfe.org.engine.expressions.raw.RawExpression;
/**
* The beginning of the implementation of a CompositeFlowExpression : examples
* of fully implemented composite flowExpressions include SequenceExpression,
* ConcurrenceExpression and the like.
* A composite expression is an expression with a list of children. These
* children are referenced as FlowExpressionId instances. Of course, each
* FlowExpressionId points to a FlowExpression in the expression pool.
*
* CVS Info :
*
$Author: jmettraux $
*
$Date: 2006-05-08 13:52:39 +0200 (Mon, 08 May 2006) $
*
$Id: AbstractCompositeFlowExpression.java 2575 2006-05-08 11:52:39Z jmettraux $
*
* @author [email protected]
*/
public abstract class AbstractCompositeFlowExpression
extends AbstractFlowExpression
implements CompositeFlowExpression
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(CompositeFlowExpression.class.getName());
//
// FIELDS
/**
* A list of FlowExpressionId instances pointing to the children
* of this composite expression.
*/
private java.util.List children = new java.util.ArrayList(0);
//
// CONSTRUCTORS
//
// BEAN METHODS
public java.util.List getChildren () { return this.children; }
public void setChildren (final java.util.List l) { this.children = l; }
//
// OVERRIDEN METHODS
/**
* This method sets the workflow instance id in the expression and
* in all of its children
*/
public void initWorkflowInstanceId (final String workflowInstanceId)
{
super.initWorkflowInstanceId(workflowInstanceId);
final java.util.Iterator it = this.children.iterator();
while (it.hasNext())
{
final FlowExpressionId fei = (FlowExpressionId)it.next();
fei.setWorkflowInstanceId(workflowInstanceId);
}
}
//
// METHODS from CompositeFlowExpression
/**
* A droflo method, moves up a child up one position
*/
public void moveUpChild (FlowExpressionId fei)
{
int i = this.children.indexOf(fei);
this.children.remove(i);
i--;
if (i < 0) i = 0;
this.children.add(i, fei);
}
/**
* A droflo method, moves up a child down one position
*/
public void moveDownChild (FlowExpressionId fei)
{
int i = this.children.indexOf(fei);
this.children.remove(i);
i++;
if (i >= this.children.size())
{
this.children.add(fei);
return;
}
this.children.add(i, fei);
}
/* *
* Replacing a child with another expression.
* Of course children are not directly referenced, instead FlowExpressionId
* instances are used.
* /
public boolean replaceChild
(final FlowExpressionId thisFei, final FlowExpressionId thatFei)
{
return Utils.replace(getChildren(), thisFei, thatFei);
}
*/
//
// METHODS from FlowExpression
/**
* Cancels this expression, this result in the cancelling of
* each of the child expression this composite references.
*/
public InFlowWorkItem cancel ()
throws ApplyException
{
InFlowWorkItem wi = null;
java.util.Iterator it = this.children.iterator();
//
// returns the first non-null workitem returned
while (it.hasNext())
{
final FlowExpressionId fei = (FlowExpressionId)it.next();
//log.debug("cancel() - "+fei);
final InFlowWorkItem i = getExpressionPool().childCancel(fei);
if (wi == null) wi = i;
}
//log.debug("cancel() done.");
super.cancel();
return wi;
}
//
// METHODS
/**
* Adds a child to the expression (as last child)
*/
public void addChild (final FlowExpressionId fei)
{
//this.children.add(fei);
this.addChild(fei, -1);
}
/**
* Adds a child to the expression (at a given position).
* A position of -1 would mean, 'insert at the end'.
*/
public void addChild
(final FlowExpressionId fei, final int position)
{
if (position > -1)
this.children.add(position, fei);
else
this.children.add(fei);
}
/**
* Removes a child from this composite expression.
*/
public void removeChild (final FlowExpressionId fei)
{
this.children.remove(fei);
}
/**
* Returns the last child.
*/
protected FlowExpression getLastChild ()
{
return getExpressionPool().fetch(getLastChildId());
}
/**
* Returns the last child's expression id.
*/
protected FlowExpressionId getLastChildId ()
{
return (FlowExpressionId)getChildren().get(getChildren().size() - 1);
/*
return (FlowExpressionId)Utils.getLastElementOfClass
(getChildren(), FlowExpressionId.class);
*/
}
/**
* Inits the expression.
*/
public void init
(final ApplicationContext context,
final FlowExpressionId environmentId,
final FlowExpressionId parentId,
final FlowExpressionId id,
final RawExpression generatingExpression,
final Object raw,
final InFlowWorkItem currentWi)
throws
BuildException
{
super.init
(context,
environmentId,
parentId,
id,
generatingExpression,
raw,
currentWi);
if (generatingExpression != null)
this.children = generatingExpression.prepareChildren(currentWi);
}
/**
* Clones this expression, takes care of deep copying everything in it.
*/
public Object clone ()
{
final AbstractCompositeFlowExpression clone =
(AbstractCompositeFlowExpression)super.clone();
clone.setChildren(AbstractFlowExpression.deepCopy(getChildren()));
return clone;
}
/**
* This method is used by ExpressionPool.dump().
*/
public org.jdom.Element dump ()
{
final org.jdom.Element elt = super.dump();
final java.util.Iterator it = this.children.iterator();
while (it.hasNext())
{
final FlowExpressionId fei = (FlowExpressionId)it.next();
final org.jdom.Element eChild = new org.jdom.Element("child");
eChild.setText(fei.toString());
elt.addContent(eChild);
}
return elt;
}
/**
* Removes asynchronously the child at the given index.
* (used by CaseExpression for instance).
*/
protected void removeBranch (final int childIndex)
{
//log.debug("removeBranch() ["+childIndex+"]");
if (childIndex >= this.children.size()) return;
removeBranch((FlowExpressionId)this.children.get(childIndex));
}
/**
* This protected method is called to remove asynchronously
* an alternative (that has become unnecessary) branch.
*/
protected void removeBranch (final FlowExpressionId fei)
{
(new Thread()
{
public void run ()
{
//
// release the now unncessary (and unevaluated) child
try
{
if (fei != null)
getExpressionPool().removeExpression(fei);
}
catch (final Throwable t)
{
log.info
("removeBranch() minor failure", t);
}
}
}).start();
}
}