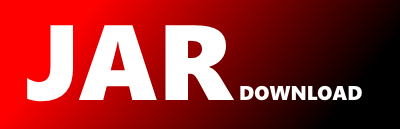
src.openwfe.org.engine.expressions.CursorExpression Maven / Gradle / Ivy
/*
* Copyright (c) 2006, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: CursorExpression.java 2852 2006-06-22 07:04:12Z jmettraux $
*/
//
// CursorExpression.java
//
// [email protected]
//
// generated with
// jtmpl 1.0.04 20.11.2001 John Mettraux ([email protected])
//
package openwfe.org.engine.expressions;
import openwfe.org.Utils;
import openwfe.org.engine.launch.LaunchException;
import openwfe.org.engine.workitem.InFlowWorkItem;
/**
* A sequence that can go forward and backward and can also be broken.
*
* CVS Info :
*
$Author: jmettraux $
*
$Date: 2006-06-22 09:04:12 +0200 (Thu, 22 Jun 2006) $
*
$Id: CursorExpression.java 2852 2006-06-22 07:04:12Z jmettraux $
*
* @author [email protected]
*/
public class CursorExpression
extends CleanCompositeFlowExpression
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(CursorExpression.class.getName());
//
// CONSTANTS
/**
* "__cursor_command__" may be set to "back", "break" or whatever other
* string.
* When set to 'back', the cursor will go back one step.
* When set to 'break', the cursor will exit.
*/
public final static String F_COMMAND
= "__cursor_command__";
/**
* When this attribute 'command-field' is set, its value is the name
* of the field that should hold the 'cursor command'.
*/
public final static String A_COMMAND_FIELD
= "command-field";
/**
* The 'back' command
*/
public final static String C_BACK
= "back";
/**
* The 'skip' command
*/
public final static String C_SKIP
= "skip";
/**
* The 'break' command
*/
public final static String C_BREAK
= "break";
/**
* The 'cancel' command : a synonym for 'break'.
*/
public final static String C_CANCEL
= "cancel";
/**
* The 'rewind' command
*/
public final static String C_REWIND
= "rewind";
/**
* The 'continue' command (it is equivalent to 'rewind')
*/
public final static String C_CONTINUE
= "continue";
/**
* The 'disallow' attribute; its value is a comma-separated list of
* commands not allowed for this cursor.
* For example <cursor disallow="break, rewind">...
*/
public final static String A_DISALLOW
= "disallow";
//
// FIELDS
private int currentId = -1;
private FlowExpressionId currentFei = null;
private String cursorCommandField = null;
//
// CONSTRUCTORS
//
// BEAN METHODS
/**
* Returns the current position of the cursor among the children list.
*/
public int getCurrentId () { return this.currentId; }
/**
* Returns the fei of the currently 'launched/running' step of the cursor.
*/
public FlowExpressionId getCurrentFei () { return this.currentFei; }
/**
* Sets the current position of the cursor among the children list.
*/
public void setCurrentId (final int i)
{
this.currentId = i;
}
public void setCurrentFei (final FlowExpressionId fei)
{
this.currentFei = fei;
}
/**
* By default, the field that should hold the cursor command is
* "__cursor_command__", but if this value is set, then it's set to
* the name of the field that should hold the 'cursor command'.
*/
public String getCursorCommandField ()
{
return this.cursorCommandField;
}
public void setCursorCommandField (final String s)
{
this.cursorCommandField = s;
}
//
// METHODS from FlowExpression
private java.util.List lookupDisallow (final InFlowWorkItem wi)
{
final String s = this.lookupAttribute(A_DISALLOW, wi);
if (s == null) return new java.util.ArrayList(0);
final String[] ss = s.split(", *");
return java.util.Arrays.asList(ss);
}
/**
* Either launches a subProcess, either launches an external process
*/
public void apply (final InFlowWorkItem wi)
throws ApplyException
{
//log.debug("apply()");
this.cursorCommandField = lookupAttribute
(A_COMMAND_FIELD,
wi,
F_COMMAND);
if (log.isDebugEnabled())
log.debug("apply() command field is >"+this.cursorCommandField+"<");
if (getChildren() == null || getChildren().size() < 1)
{
if (isLoop())
{
log.warn("apply() loop with no children !!!");
/*
apply(wi);
return;
*/
throw new ApplyException("loop with no children !");
}
else
{
applyToParent(wi);
return;
}
}
// determining the 'disallow' attribute value
final java.util.List lDisallow = lookupDisallow(wi);
// determining the 'command'
//String sCommand = wi.getAttributes().sget(F_COMMAND);
String sCommand = wi.getAttributes().sget(this.cursorCommandField);
if (sCommand == null)
sCommand = "";
else
sCommand = sCommand.trim();
//log.debug("apply() sCommand is >"+sCommand+"<");
if (lDisallow.contains(sCommand)) sCommand = "";
if (log.isDebugEnabled())
log.debug("apply() sCommand' is >"+sCommand+"<");
if (sCommand.equals(C_BREAK) || sCommand.equals(C_CANCEL))
//
// exiting the cursor
{
applyToParent(wi);
return;
}
if (sCommand.equals(C_REWIND) || sCommand.equals(C_CONTINUE))
{
this.currentId = 0;
}
else if (sCommand.startsWith(C_BACK) || sCommand.startsWith(C_SKIP))
{
this.currentId += extractStep(sCommand);
}
else
{
this.currentId++;
}
if (this.currentId < 0) this.currentId = 0;
if (this.currentId >= getChildren().size())
//
// no more children to apply
{
if (isLoop())
{
this.currentId = 0;
}
else
{
applyToParent(wi);
return;
}
}
//wi.getAttributes().puts(F_COMMAND, "");
wi.getAttributes().puts(this.cursorCommandField, "");
// reset the cursor command
FlowExpressionId next = null;
this.storeItself();
next = (FlowExpressionId)getChildren().get(this.currentId);
//log.debug("apply() current is now "+this.currentId);
try
{
this.currentFei = getLauncher().launchSub
(wi,
this.getId(), // parent
next, // templateId
null,
true); // async call (will return immediately)
this.storeItself();
// taking care of storing the 'currentFei'
}
catch (final LaunchException e)
{
log.warn
("apply() next expression failed .... "+next.toString(), e);
}
}
private int extractStep (final String cursorCommand)
{
if (log.isDebugEnabled())
log.debug("extractStep() extract step from '"+cursorCommand+"'");
final String[] ss = cursorCommand.split("\\s+");
int step = 1;
if (ss.length > 1)
{
try
{
step = Integer.parseInt(ss[1]);
}
catch (final Throwable t)
{
if (log.isDebugEnabled())
{
log.debug
("extractStep() "+
"cannot determine step in '"+cursorCommand+"'", t);
}
}
}
if (cursorCommand.startsWith(C_BACK))
step = -step;
return step;
}
/**
* If the launch was done, will reply to the parent expression, else
* it will trigger the launch (if all children did reply).
* This method is synchronized to avoid collision between children replies.
*/
public void reply (final InFlowWorkItem wi)
throws ReplyException
{
try
{
apply(wi);
}
catch (final ApplyException e)
{
throw new ReplyException("reply failure", e);
}
}
/**
* Overrides AbstractFlowExpression.applyToParent(), to make sure the
* children are removed as well from the expression pool (they are just
* templates anyway).
*/
public void applyToParent (final InFlowWorkItem wi)
throws ApplyException
{
wi.getAttributes().puts(this.cursorCommandField, "");
// reset the cursor command
//
// clean templates
final java.util.Iterator it = getChildren().iterator();
while (it.hasNext())
{
final FlowExpressionId childId = (FlowExpressionId)it.next();
getExpressionPool().removeExpression(childId);
}
//
// reply to parent
super.applyToParent(wi);
}
/**
* Overrides AbstractCompositeFlowExpression.cancel() and makes sure
* currently running templated children are cancelled as well.
*/
public InFlowWorkItem cancel ()
throws ApplyException
{
final InFlowWorkItem wi =
getExpressionPool().childCancel(this.currentFei);
super.cancel();
return wi;
}
//
// METHODS
/**
* This class instances will always return false, but extending
* classes like 'loop' may reply with true.
*/
public boolean isLoop ()
{
return false;
}
}