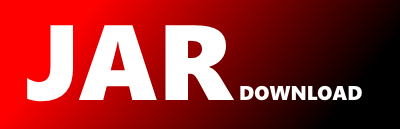
src.openwfe.org.engine.expressions.GetValueExpression Maven / Gradle / Ivy
/*
* Copyright (c) 2001-2006, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: GetValueExpression.java 2667 2006-05-24 22:33:06Z jmettraux $
*/
//
// GetValueExpression.java
//
// [email protected]
//
// generated with
// jtmpl 1.1.01 2004/05/19 ([email protected])
//
package openwfe.org.engine.expressions;
import openwfe.org.xml.XmlUtils;
import openwfe.org.engine.Definitions;
import openwfe.org.engine.workitem.Attribute;
import openwfe.org.engine.workitem.XmlAttribute;
//import openwfe.org.engine.workitem.StringAttribute;
import openwfe.org.engine.workitem.CollectionAttribute;
import openwfe.org.engine.workitem.InFlowWorkItem;
import openwfe.org.engine.expressions.raw.RawExpression;
import openwfe.org.engine.expressions.xeme.XemeUtils;
import openwfe.org.engine.impl.workitem.xml.XmlWorkItemCoder;
/**
* The child (or content) of this expression is 'piped' into the replied
* workitem as the value of the field '__result__'.
*
* CVS Info :
*
$Author: jmettraux $
*
$Id: GetValueExpression.java 2667 2006-05-24 22:33:06Z jmettraux $
*
* @author [email protected]
*/
public class GetValueExpression
extends ZeroChildExpression
implements WithChildren
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(GetValueExpression.class.getName());
//
// CONSTANTS & co
//
// FIELDS
//
// CONSTRUCTORS
//
// METHODS from FlowExpression
public void apply (final InFlowWorkItem wi)
throws ApplyException
{
final String expressionName = this.getId().getExpressionName();
final String key = lookupAttribute(A_VALUE, wi);
//log.debug("apply() key >"+key+"<");
//log.debug("apply() expressionName >"+expressionName+"<");
Object value = null;
if (expressionName.startsWith("q"))
//
// quote
{
value = handleQuote(wi, key);
}
else if (expressionName.startsWith("a"))
//
// attribute (workitem fragment)
{
value = handleAttribute(wi, key);
}
else if (key != null)
{
if (expressionName.startsWith("v"))
//
// variable lookup
{
value = handleVariable(wi, key);
}
else if (expressionName.startsWith("f"))
//
// field lookup
{
value = handleField(wi, key);
}
}
if (value != null)
{
if (value instanceof Attribute)
//
// after handleAttribute
{
ValueUtils.setResult
(wi, value);
}
else
//
// other cases (v, f, q)
{
ValueUtils.setResult
(wi, new XmlAttribute((org.jdom.Content)value));
}
}
else
{
ValueUtils.cleanResult(wi);
}
//
// resume flow
applyToParent(wi);
}
//
// METHODS
protected org.jdom.Content handleVariable
(final InFlowWorkItem wi, final String key)
{
final Object o = this.lookupVariable(key);
if (log.isDebugEnabled())
{
log.debug("handleVariable() key >"+key+"<");
log.debug("handleVariable() input >"+o+"<");
if (o != null)
{
log.debug
("handleVariable() input.class "+o.getClass().getName());
}
}
final org.jdom.Content c = XemeUtils.toXemeResult(this, o);
if (log.isDebugEnabled())
log.debug("handleVariable() result is\n"+XmlUtils.xmlToString(c));
return c;
}
protected org.jdom.Content handleField
(final InFlowWorkItem wi, final String key)
{
final Attribute a = CollectionAttribute
.lookupAttribute(key, wi.getAttributes());
return XemeUtils.toXemeResult(this, a);
}
protected Attribute handleAttribute
(final InFlowWorkItem wi, final String key)
{
//log.debug("handleAttribute()");
Attribute value = null;
try
{
value = ValueUtils.determineWorkitemFragment(this, wi);
}
catch (final ValueException e)
{
//log.debug
// ("handleAttribute() failed to fetch workitem fragment : "+
// e.getMessage());
log.debug
("handleAttribute() failed to fetch workitem fragment", e);
//
// resuming anyway ... value == null
}
if (value == null)
value = ValueUtils.getResult(wi);
return value;
}
public org.jdom.Content handleQuote
(final InFlowWorkItem wi, final String key)
{
final String sContent = this.lookupAttribute(A_CONTENT, wi);
if (sContent != null)
{
//log.debug("handleQuote() content >"+sContent+"<");
try
{
final org.jdom.Element eQuote =
XmlUtils.extractXmlElement(sContent);
//return (org.jdom.Content)eQuote.getChildren().get(0);
//return (org.jdom.Content)eQuote.getContent().get(0);
for (int i=0; i 0)
//return new StringAttribute(sContent.trim());
return XemeUtils.toXemeResult(this, sContent.trim());
}
//return new StringAttribute(key);
return XemeUtils.toXemeResult(this, key);
}
//
// STATIC METHODS
}