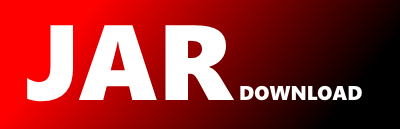
src.openwfe.org.engine.expressions.IfExpression Maven / Gradle / Ivy
/*
* Copyright (c) 2001-2006, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: IfExpression.java 2667 2006-05-24 22:33:06Z jmettraux $
*/
//
// IfExpression.java
//
// [email protected]
//
// generated with
// jtmpl 1.0.04 31.10.2002 John Mettraux ([email protected])
//
package openwfe.org.engine.expressions;
import openwfe.org.OpenWfeException;
import openwfe.org.ApplicationContext;
import openwfe.org.engine.Definitions;
import openwfe.org.engine.expool.ExpressionPool;
import openwfe.org.engine.workitem.InFlowWorkItem;
import openwfe.org.engine.expressions.map.ExpressionMap;
import openwfe.org.engine.impl.functions.BasicFunctions;
/**
* If the first child of the expression evaluates to true, the second child
* of the expression will be evaluated else the third.
*
* CVS Info :
*
$Author: jmettraux $
*
$Date: 2006-05-25 00:33:06 +0200 (Thu, 25 May 2006) $
*
$Id: IfExpression.java 2667 2006-05-24 22:33:06Z jmettraux $
*
* @author [email protected]
*/
public class IfExpression
extends CleanCompositeFlowExpression
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(IfExpression.class.getName());
//
// CONSTANTS & co
/**
* If this if expression has a 'test' attribute set, it means it's a
* 'shorcut if' and the condition to eval is not the first children, but
* the value of this 'test' attribute.
*/
public final static String A_TEST
= "test";
//
// FIELDS
/*
* 'childOffset' when set to 1 when that there is a conditional clause.
* => the 'then' is at position 1 (0+1) and the 'else' at 2. (1+1)
*
* If set to 0, it means a 'test' attribute was passed to the 'if' tag
* => the 'then' is at position 0 (0+0) and the 'else' at 2. (1+0)
*/
private int childOffset = 1;
private boolean conditionTreated = false;
//
// CONSTRUCTORS
//
// BEAN METHODS
public boolean isConditionTreated () { return this.conditionTreated; }
public void setConditionTreated (boolean b) { this.conditionTreated = b; }
//
// METHODS
public void apply (final InFlowWorkItem wi)
throws ApplyException
{
final String test = lookupAttribute(A_TEST, wi);
if (test == null)
//
// the classical case (until OpenWFE 1.5.4) :
//
//
//
//
//
{
final FlowExpressionId next =
(FlowExpressionId)getChildren().get(0);
getExpressionPool().apply(next, wi);
return;
}
//
// the 'shortcut' case :
//
//
//
//
this.childOffset = 0;
try
{
applyConsequence(evalTest(test), wi);
}
catch (final OpenWfeException e)
{
if (e instanceof ApplyException)
throw (ApplyException)e;
throw new ApplyException
("failed to apply (shortcutted) consequence", e);
}
}
/**
* Evals the 'test' attribute of the shortcutted if expression :
* returns true if the test evaluates to true.
*/
protected boolean evalTest (final String test)
{
return BasicFunctions.eval(test);
}
/**
* The 'reply' method of this if expression.
*/
public void reply (final InFlowWorkItem wi)
throws ReplyException
{
if (this.conditionTreated)
{
//ValueUtils.cleanBooleanResult(wi);
if (log.isDebugEnabled())
{
log.debug("reply() condition treated. Replying to parent.");
log.debug("reply() parent is "+this.getParent());
}
replyToParent(wi);
return;
}
final boolean booleanResult = ValueUtils.lookupBooleanResult(wi);
try
{
applyConsequence(booleanResult, wi);
}
catch (final OpenWfeException e)
{
if (e instanceof ReplyException)
throw (ReplyException)e;
throw new ReplyException
("Failed to trigger consequence", e);
}
}
/**
* This method applies the 'then' or the 'else' child (at the
* end it also replies to the parent expression of the if).
*/
protected void applyConsequence
(final boolean booleanResult, final InFlowWorkItem wi)
throws
OpenWfeException
{
FlowExpressionId next = null;
FlowExpressionId alternative = null;
FlowExpressionId thenChild = null;
if (getChildren().size() > this.childOffset)
{
thenChild =
(FlowExpressionId)getChildren().get(this.childOffset);
//thenChild =
// (FlowExpressionId)getExpressionChildAt(this.childOffset);
}
FlowExpressionId elseChild = null;
if (getChildren().size() > this.childOffset+1)
{
elseChild =
(FlowExpressionId)getChildren().get(this.childOffset+1);
}
if (booleanResult)
{
//log.debug("reply() triggering 'then' branch");
next = thenChild;
alternative = elseChild;
}
else
{
//log.debug("reply() triggering 'else' branch");
next = elseChild;
alternative = thenChild;
}
this.conditionTreated = true;
this.storeItself();
removeBranch(alternative);
//
// removes the unnecessary (and unevaluated) branch
if (next != null)
{
getExpressionPool().apply(next, wi);
}
else
{
//ValueUtils.cleanBooleanResult(wi);
replyToParent(wi);
}
}
}