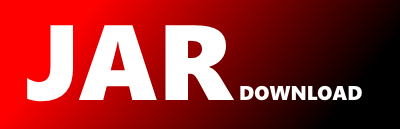
src.openwfe.org.engine.impl.dispatch.SmtpDispatcher Maven / Gradle / Ivy
/*
* Copyright (c) 2001-2006, John Mettraux, OpenWFE.org
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* . Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* . Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* . Neither the name of the "OpenWFE" nor the names of its contributors may be
* used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* $Id: SmtpDispatcher.java 2658 2006-05-24 10:30:29Z jmettraux $
*/
//
// SmtpDispatcher.java
//
// [email protected]
//
// generated with
// jtmpl 1.0.04 20.11.2001 John Mettraux ([email protected])
//
package openwfe.org.engine.impl.dispatch;
import javax.mail.Message;
import javax.mail.Message.RecipientType;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.MessagingException;
import javax.mail.internet.MimeMessage;
import javax.mail.internet.InternetAddress;
import openwfe.org.Utils;
import openwfe.org.MapUtils;
import openwfe.org.ServiceException;
import openwfe.org.ApplicationContext;
//import openwfe.org.xml.XmlCoder;
import openwfe.org.mail.MailUtils;
import openwfe.org.engine.workitem.WorkItem;
import openwfe.org.engine.workitem.InFlowWorkItem;
import openwfe.org.engine.dispatch.DispatchingException;
import openwfe.org.engine.dispatch.AbstractWorkItemDispatcher;
import openwfe.org.engine.expressions.FlowExpressionId;
/**
* A very simple dispatcher, dispatches to a directory
*
* CVS Info :
*
$Author: jmettraux $
*
$Date: 2006-05-24 12:30:29 +0200 (Wed, 24 May 2006) $
*
$Id: SmtpDispatcher.java 2658 2006-05-24 10:30:29Z jmettraux $
*
* @author [email protected]
*/
public class SmtpDispatcher
extends AbstractWorkItemDispatcher
{
private final static org.apache.log4j.Logger log = org.apache.log4j.Logger
.getLogger(SmtpDispatcher.class.getName());
//
// CONSTANTS (and co)
/* *
* Use this parameter ('mail-from') to indicate who should be the
* apparent author of the email
* /
public final static String P_MAIL_FROM
= "mail-from";
*/
/**
* Sets the email address that will receive the dispatched workitem by
* using the 'recipient' parameter for this Dispatcher in the
* participant map.
*/
public final static String P_RECIPIENT
= "recipient";
/**
* Tells the dispatcher it should look for the recipient in the workitem
* field whose name is given as value of this 'recipient-field' parameter.
*/
public final static String P_RECIPIENT_FIELD
= "recipient-field";
/**
* Use this 'cc' parameter to set a 'carbon-copy' recipient, maybe an
* archive account.
*/
public final static String P_CC
= "cc";
/**
* Message emitted by this dispatched have "openwfe.workitem::"
* prefixing their subjects.
*/
public final static String SUBJECT_PREFIX
= "openwfe.workitem::";
//
// FIELDS
private String recipient = null;
private String recipientField = null;
private String cc = null;
//
// CONSTRUCTORS
/**
* Inits this dispatcher.
*/
public void init
(final String serviceName,
final ApplicationContext context,
final java.util.Map serviceParams)
throws
ServiceException
{
super.init(serviceName, context, serviceParams);
this.recipient =
MapUtils.getAsString(serviceParams, P_RECIPIENT);
this.recipientField =
MapUtils.getAsString(serviceParams, P_RECIPIENT_FIELD);
this.cc =
MapUtils.getAsString(serviceParams, P_CC);
}
//
// METHODS
/**
* Dispatches the workitem as the XML body of an email message over
* SMTP.
*/
public Object dispatch (final WorkItem wi)
throws DispatchingException
{
try
{
final Session mailSession = MailUtils.getMailSession(getParams());
final MimeMessage m = new MimeMessage(mailSession);
MailUtils.setMailFrom(m, getParams());
String sRecipient = this.recipient;
if (this.recipientField != null)
{
final String s = wi.getAttributes().sget(this.recipientField);
if (s != null) sRecipient = s;
}
if (sRecipient == null)
{
throw new DispatchingException
("Cannot dispatch to 'null' recipient");
}
MailUtils.addRecipients(m, RecipientType.TO, sRecipient);
MailUtils.addRecipients(m, RecipientType.CC, this.cc);
m.setSubject(determineSubject(wi));
final byte[] payload = (byte[])instantiateEncoder()
.encode(wi, getContext(), getParams());
final StringBuffer sb = new StringBuffer();
sb
.append(getWorkItemCoderName())
.append("\n")
.append(new String(payload, Utils.getEncoding()));
m.setText(sb.toString(), Utils.getEncoding());
mailSession.getTransport("smtp").send(m);
log.debug("dispatch() mail sent to '"+sRecipient+"'");
}
catch (final Throwable t)
{
log.debug
("Failed to dispatch workitem over SMTP", t);
throw new DispatchingException
("Failed to dispatch workitem over SMTP", t);
}
return null;
}
/**
* Determines the mail subject out of the workitem
*/
protected String determineSubject (final WorkItem wi)
{
String subject = SUBJECT_PREFIX + wi.getClass().getName();
if (wi instanceof InFlowWorkItem)
{
final InFlowWorkItem ifwi = (InFlowWorkItem)wi;
final FlowExpressionId id = ifwi.getLastExpressionId();
final StringBuffer sb = new StringBuffer();
sb
.append(SUBJECT_PREFIX)
.append(id.getEngineId())
.append(":")
.append(id.getWorkflowInstanceId())
.append(":")
.append(id.getExpressionId());
subject = sb.toString();
}
return subject;
}
}